How to Map an Array With Index in Ruby
-
Understanding Ruby’s
map
Method -
Combine
map
andeach_with_index
Methods in Ruby -
Combine
map
andwith_index
Methods in Ruby -
Use
map
Together With the Ruby Range - Conclusion
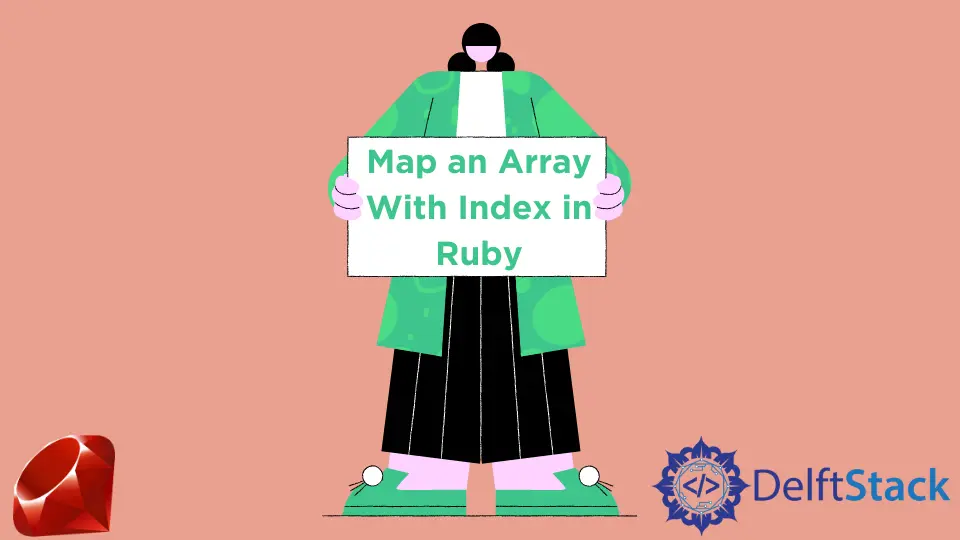
Array manipulation is a fundamental aspect of programming, and in the Ruby programming language, the map
method stands out as a versatile tool for transforming array elements. When it comes to mapping an array with its indices, Ruby provides several approaches, each offering elegance and flexibility.
In this article, we’ll explore various methods, including each_with_index
, with_index
, and the Ruby Range, to seamlessly map arrays with their corresponding indices. Understanding these techniques not only empowers you to manipulate arrays efficiently but also enhances the expressiveness and readability of your Ruby code.
Understanding Ruby’s map
Method
The map
method is a fundamental component of Ruby’s Enumerable module, offering a clean and efficient way to transform each element of an array based on a provided block. This block of code is applied to every item in the array, and the results are collected into a new array.
The original array remains unchanged, making map
a non-destructive method. The basic syntax of the map
method is straightforward:
new_array = original_array.map { |element| # transformation code here }
Here, original_array
is the array you want to transform, and the block of code within the curly braces defines how each element should be modified. The result is stored in new_array
.
Let’s delve into the inner workings of the map
method through a simple example. Suppose we have an array of numbers and want to create a new array with each number squared.
numbers = [1, 2, 3, 4, 5]
squared_numbers = numbers.map { |num| num**2 }
puts squared_numbers
Output:
In this example, the map
method iterates through each element of the numbers
array, applies the block of code ({ |num| num**2 }
) to square each element, and collects the results into a new array (squared_numbers
). The final output is an array containing the squared values.
The true power of map
lies in its ability to succinctly express transformations. Let’s take a look at another scenario:
words = %w[ruby map method]
uppercase_words = words.map { |word| word.upcase }
puts uppercase_words
Output:
Here, the map
method iterates over each element of the words
array and applies the block of code within the curly braces to transform each element. In this case, the block takes each word
and uses the upcase
method to convert it to uppercase.
Combine map
and each_with_index
Methods in Ruby
Now, let’s take it a step further by incorporating the each_with_index
method to map an array with its corresponding indices. This combination proves invaluable when you need to associate each element with its position in the array.
The each_with_index
method, belonging to the Enumerable module in Ruby, allows us to iterate through a Ruby array while simultaneously accessing the index of each element. When paired with the map
method, it becomes a powerful tool for mapping an array with its indices.
The combined syntax looks like this:
new_array = original_array.each_with_index.map { |element, index| # transformation code here }
Here, original_array
is the array you want to iterate over, and the block of code within the curly braces defines both the element and its index. The transformed results are collected into a new array, preserving the original array.
Now, let’s apply this combination to a real-world scenario. Consider a situation where we have an array of words, and we want to create a new array that includes both the word and its index.
words = %w[ruby map method]
numbered_words = words.each_with_index.map { |word, index| "#{index + 1}: #{word}" }
puts numbered_words
In this example, we start with the array words
containing three elements. We use each_with_index
to iterate over each element and gain access to both the word
and its index
.
Inside the block, we construct a new string that includes the index (adjusted to start from 1) and the original word. The map
method then collects these transformed strings into a new array, resulting in numbered_words
.
Code Output:
In this output, each word from the original array is now accompanied by its corresponding index, forming a new array that provides valuable information about the position of each element. This combination of each_with_index
and map
showcases the elegance and expressiveness of Ruby, allowing developers to efficiently handle complex transformations while maintaining code clarity.
Combine map
and with_index
Methods in Ruby
The with_index
method, like each_with_index
, belongs to the Enumerable
module in Ruby. It allows us to iterate through an array, providing access to both the element and its index.
When combined with the map
method, it streamlines the process of transforming an array by eliminating the need for a separate each_with_index
call. The combined syntax is as follows:
new_array = original_array.map.with_index(offset) { |element, index| # transformation code here }
Here, original_array
is the array to iterate over, and the block within the curly braces defines both the element and its index. The transformed results are collected into a new array. The optional offset
argument determines the starting index.
Let’s apply this method to a practical scenario. Imagine we have an array of temperatures, and we want to create a new array that includes both the temperature and its corresponding day.
temperatures = [22, 24, 20, 18, 25]
numbered_temperatures = temperatures.map.with_index(1) { |temp, day| "Day #{day}: #{temp}°C" }
puts numbered_temperatures
In this example, the array temperatures
contains five elements representing daily temperature values. We use map.with_index(1)
to iterate over each temperature, gaining access to both the temp
and its day
(index).
Inside the block, a new string is constructed that includes the day and temperature. The map
method then collects these transformed strings into a new array, resulting in numbered_temperatures
.
Code Output:
In this output, each temperature from the original array is paired with its corresponding day, forming a new array that provides meaningful information about each element’s position.
Use the with_index
Method With the offset
Parameter
It’s also worth mentioning that with_index
accepts an argument that is the index’s offset
, where the index should start from. This feature is particularly useful when you want to customize the starting index rather than relying on the default zero-based indexing.
By passing an argument to with_index
, you can control the initial value of the index. This eliminates the need to manually add an offset, such as i + 1
, within the block that defines the transformation logic.
Let’s explore this concept with an example:
letters = %w[a b c d]
offset_numbered_letters = letters.map.with_index(10) { |letter, index| "#{index}: #{letter}" }
puts offset_numbered_letters
In this example, the with_index(10)
argument signifies that the index should start from the value 10 instead of the default 0. As a result, the output will be:
Here, each letter from the original array is paired with an index starting from 10, demonstrating how the with_index
offset simplifies the process of customizing the initial index value.
This feature becomes particularly handy in situations where a specific numbering convention or starting point is required for your array elements. By incorporating the with_index
offset, you enhance the readability and maintainability of your code, as the adjustment is applied globally to the entire iteration process rather than within the block for each element.
Use map
Together With the Ruby Range
Now, let’s extend our toolkit by exploring another approach – mapping an array with its indices using the Ruby Range.
The Ruby Range is a versatile construct representing a set of values within a specified range. When combined with the map
method, it becomes a handy tool for mapping an array with its indices.
The basic syntax looks like this:
new_array = (start_index...end_index).map { |index| # transformation code here }
In this syntax, start_index
and end_index
define the range of indices for the array, and the block within the curly braces specifies the transformation logic. The map
method efficiently collects the transformed results into a new array.
Let’s apply the Ruby Range approach to a real-world example. Suppose we have an array of programming languages, and we want to create a new array with each language followed by its index.
languages = %w[Ruby Python JavaScript Java]
numbered_languages = (0...languages.length).map { |index| "#{index + 1}: #{languages[index]}" }
puts numbered_languages
In this example, we start with the array languages
containing four elements. The Ruby Range (0...languages.length)
generates a sequence of indices from 0 to one less than the length of the array.
The map
method iterates through each index, and within the block, we construct a new string that includes the adjusted index (starting from 1) and the original programming language. The results are collected into a new array, resulting in numbered_languages
.
Code Output:
In this output, each programming language from the original array is accompanied by its corresponding index, forming a new array that provides valuable information about the position of each element.
Conclusion
In Ruby programming, mapping arrays with their indices is a common task, and the language provides multiple avenues to achieve this goal. Whether you prefer the clarity of each_with_index
, the succinctness of with_index
, or the simplicity of the Ruby Range, each method offers a unique approach to array transformation.
The key is to choose the method that aligns with your coding style and specific requirements. By mastering these techniques, you’ll find yourself equipped to elegantly navigate and manipulate arrays while maintaining the expressive and readable nature that defines Ruby code.
Related Article - Ruby Array
- %i and %I in Ruby
- How to Combine Array to String in Ruby
- How to Square Array Element in Ruby
- How to Merge Arrays in Ruby
- How to Convert Array to Hash in Ruby
- How to Remove Duplicates From a Ruby Array