How to Convert Array to Hash in Ruby
- Convert Array to Hash in Ruby
-
Use
Array.to_h
to Convert Array to Hash in Ruby -
Use
Hash::[]
to Convert Array to Hash in Ruby -
Use
Enumerable#each_with_object
to Convert Array to Hash in Ruby - Conclusion
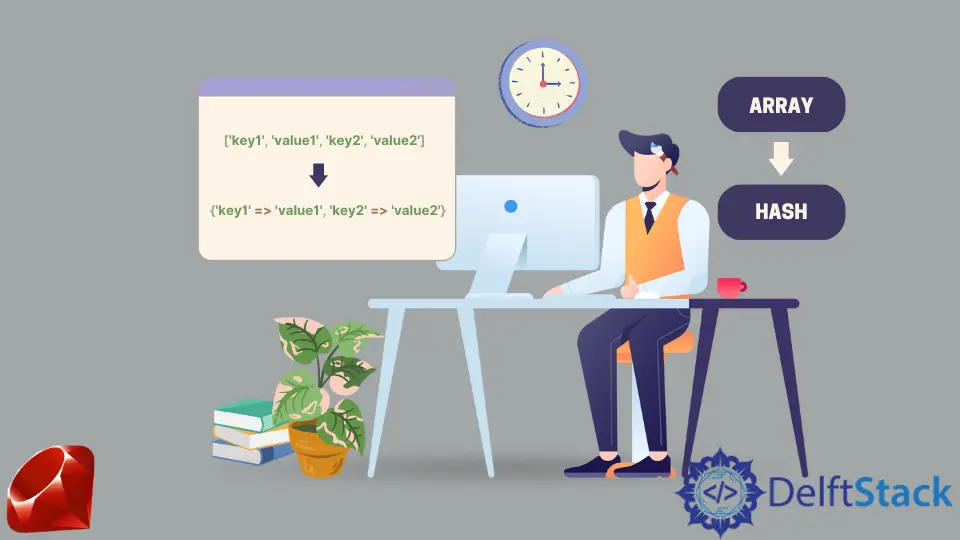
In this article, we’ll discuss the methods for converting arrays into hashes in Ruby.
Convert Array to Hash in Ruby
Arrays and hashes are two essential data structures in Ruby, each serving distinct purposes. While arrays provide an ordered collection of elements accessible by index, hashes offer a way to store data in key-value pairs, enabling efficient retrieval and manipulation.
This article will shortly discuss the solution to convert the following array:
%w[key1 value1 key2 value2]
Into a hash with the following format:
{ 'key1' => 'value1', 'key2' => 'value2' }
Use Array.to_h
to Convert Array to Hash in Ruby
Ruby version 2.1.10 introduced the convenient to_h
method for arrays, designed to interpret a 2-element array into a hash. The process involves converting the original array into a 2-element array using each_slice(2).to_a
and then applying to_h
.
Basic Syntax:
result_hash = array.each_slice(2).to_a.to_h
In the syntax, the array
is our original array containing alternating key-value pairs or subarrays representing key-value pairs. The each_slice(2)
method is applied to the array, grouping its elements into subarrays of size 2.
Next, the to_a
converts the grouped elements into a nested array, and the to_h
method is then applied to the nested array, interpreting it as key-value pairs and creating a hash.
Lastly, the result_hash
is the variable that holds the resulting hash.
Let’s look into an example. We first need to convert our original array to a 2-element array.
Code:
puts %w[key1 value1 key2 value2].each_slice(2).to_a
In this code, we use the %w
syntax to create an array containing alternating key-value pairs: ['key1', 'value1', 'key2', 'value2']
. The each_slice(2)
method is then applied to group these elements into subarrays of size 2.
Finally, to_a
converts these subarrays into a nested array.
Output:
key1
value1
key2
value2
Then, we apply the to_h
method after to_a
to convert the array into a hash.
Code:
puts %w[key1 value1 key2 value2].each_slice(2).to_a.to_h
In this code, the subsequent to_a
call converts these subarrays into a nested array. Building on this, we apply to_h
, a method specific to Ruby arrays, which seamlessly converts this nested array into a hash.
Output:
{ "key1"=>"value1", "key2"=>"value2" }
This one-liner code transforms an array of alternating key-value pairs into a hash.
Use Hash::[]
to Convert Array to Hash in Ruby
The Hash::[]
method in Ruby is an alternative way to convert an array of key-value pairs into a hash. This method directly creates a hash from a list of arguments, where each odd-indexed argument becomes a key, and the following even-indexed argument becomes its corresponding value.
Code:
puts Hash['key1', 'value1', 'key2', 'value2']
In this code, we utilize the Hash::[]
method to directly create a hash from a list of key-value pairs. The arguments 'key1'
, 'value1'
, 'key2'
, and 'value2'
are supplied, with each odd argument serving as a key and each even argument as a corresponding value.
The result is an instantly generated hash.
Output:
{"key1"=>"value1", "key2"=>"value2"}
This single line of code demonstrates the efficiency and simplicity of creating a hash in Ruby by directly specifying key-value pairs using the Hash::[]
method.
Use Enumerable#each_with_object
to Convert Array to Hash in Ruby
The Enumerable#each_with_object
method in Ruby is a flexible way to iterate over a collection (such as an array) while building an object (in this case, a hash). It allows you to define an initial object (in this case, an empty hash) and then perform a block of code for each element in the collection, gradually building up the object.
Code:
result = %w[key1 value1 key2 value2].each_slice(2).each_with_object({}) do |(key, value), hash|
hash[key] = value
end
puts result
In this code, we utilize the %w
syntax to create an array with alternating key-value pairs: ['key1', 'value1', 'key2', 'value2']
. Employing each_slice(2)
, we group these elements into pairs, and then, with each_with_object({})
, we iterate through the pairs, gradually building a hash.
The block destructures each pair into key
and value
, and the hash is constructed by assigning each key-value pair. The resulting hash is stored in the result
variable, and the final puts result
command prints the output.
Output:
{"key1"=>"value1", "key2"=>"value2"}
This one-liner succinctly showcases the use of each_with_object
to efficiently convert an array of key-value pairs into a hash in Ruby.
Conclusion
In conclusion, our exploration of converting arrays to hashes in Ruby has provided a comprehensive overview of versatile methods catering to diverse needs and preferences. We’ve covered the Array.to_h
method, introduced in Ruby version 2.1.10
, which elegantly interprets a 2-element array into a hash.
Additionally, the direct and powerful Hash::[]
method proves efficient for instant hash creation from key-value pairs. Finally, we introduced the flexible Enumerable#each_with_object
method, offering fine-grained control and custom logic integration during the conversion process.
These techniques enhance code readability, efficiency, and flexibility, empowering programmers to navigate the intricacies of data manipulation with confidence and precision.