How to Remove Duplicates From a Ruby Array
-
Use the
uniq
Method to Remove Duplicates From a Ruby Array -
Use the
uniq!
Method to Remove Duplicates From a Ruby Array -
Use the
to_set
Method to Remove Duplicates From a Ruby Array - Use Set Operations to Remove Duplicates From a Ruby Array
-
Use the
select
Method to Remove Duplicates From a Ruby Array -
Use the
uniq
Method With a Block to Remove Duplicates From a Ruby Array - Conclusion
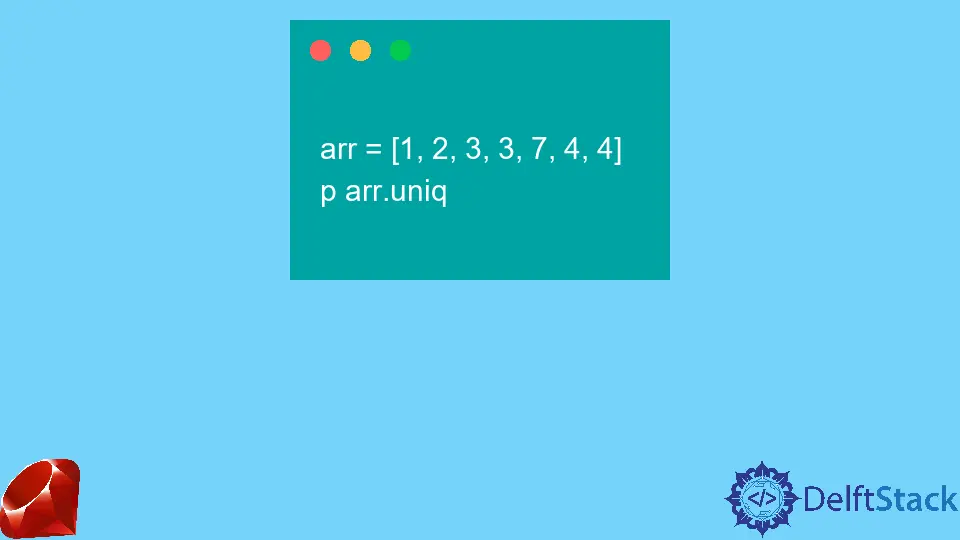
In this article, we’ll discuss the different methods of removing duplicates from an array in Ruby. Each method is accompanied by examples, offering code snippets and corresponding output for better understanding.
Whether you prioritize simplicity, in-place modification, or unique set operations, this guide provides a diverse set of options to cater to various programming needs when working with Ruby arrays.
Use the uniq
Method to Remove Duplicates From a Ruby Array
The uniq
method is the most common approach for removing Ruby array duplicates. When applied to an array, it returns a new array containing only the unique elements from the original array.
The order of elements in the resulting array is based on their first occurrence in the original array. The uniq
method does not modify the original array; instead, it produces a new array without duplicates.
Example code:
arr = [1, 2, 3, 3, 7, 4, 4]
p arr.uniq
Output:
[1, 2, 3, 7, 4]
In the code, we initialize an array arr
containing elements, some of which are duplicates. The uniq
method is then applied to the array, and the result is printed using p
.
The uniq
method effectively eliminates duplicate elements, leaving us with a new array that contains unique elements. In this specific example, the output of p arr.uniq
would be [1, 2, 3, 7, 4]
, reflecting the modified array without any duplicate entries.
Use the uniq!
Method to Remove Duplicates From a Ruby Array
The uniq!
method in Ruby is similar to the uniq
method but with a crucial difference: it modifies the original array in place. When applied to an array, uniq!
removes duplicate elements from the array and modifies the array itself to contain only unique elements.
If there are no duplicates, it returns nil
. If modifications are made, it returns the modified array. This method can be useful when you want to alter the existing array and do not need a separate copy with unique elements.
Example code:
arr = [1, 2, 3, 3, 7, 4, 4]
p arr.uniq!
Output:
[1, 2, 3, 7, 4]
In this code, we initialize an array arr
with various elements, including duplicates. The objective is to modify the original array in place, removing any duplicate entries.
We achieve this by using the uniq!
method, which directly modifies the array by eliminating duplicate elements. The p arr.uniq!
statement prints the modified array after the removal of duplicates.
In this case, the output would be [1, 2, 3, 7, 4]
, representing the original array with duplicate elements removed. The exclamation mark in uniq!
indicates the in-place modification of the array.
Use the to_set
Method to Remove Duplicates From a Ruby Array
Another way of removing duplicates is by converting the array to a set.
The to_set
method in Ruby is not specifically designed to remove duplicates from an array. Instead, it is used to convert an array to a Set, which is a collection of unordered, unique elements.
When applied to an array, to_set
creates a new Set object containing only the unique elements from the original array. The order of elements in the Set is not guaranteed to be the same as in the original array.
If the goal is to eliminate duplicates from an array, using uniq
or uniq!
is generally more straightforward. However, converting an array to a Set using to_set
can be useful in scenarios where the uniqueness of elements and the unordered nature of a Set are specifically required.
Example Code:
require 'set'
arr = [1, 2, 3, 3, 7, 4, 4]
p arr.to_set.to_a
Output:
[1, 2, 3, 7, 4]
In this code, we start with an array named arr
containing various elements, some of which are duplicates. The goal is to create a new array that includes unique elements.
To achieve this, we use the to_set
method to convert the array into a set, taking advantage of the set’s inherent property of uniqueness. Next, we apply to_a
to convert the set back to an array.
The p arr.to_set.to_a
statement then prints the resulting array, which, in this case, would be [1, 2, 3, 7, 4]
.
This demonstrates an approach where the array is temporarily converted to a set to automatically eliminate duplicates, providing a concise way to obtain an array with unique elements.
Use Set Operations to Remove Duplicates From a Ruby Array
When a set operation is used on arrays, the arrays are implicitly converted to sets. Thus, as discussed in the previous method, the duplicates inside the array are removed.
Example code of using the intersection (&
) operator:
arr = [1, 2, 3, 3, 7, 4, 4]
p(arr & arr)
Output:
[1, 2, 3, 7, 4]
In the above example, we initialize an array named arr
with various elements, including duplicates. Then, we use the &
(intersection) operator on the array with itself.
The p(arr & arr)
statement prints the resulting array, which, in this specific example, would be [1, 2, 3, 7, 4]
. The intersection (&
) operator, in this context, acts as a concise way to find the common elements and effectively removes the duplicates.
Example code of using the union (|
) operator:
arr = [1, 2, 3, 3, 7, 4, 4]
p(arr | arr)
Output:
[1, 2, 3, 7, 4]
In this code, we initialize an array named arr
with various elements, some of which are duplicates. Next, we use the |
(union) operator on the array with itself.
The p(arr | arr)
statement prints the resulting array, which, in this specific example, would be [1, 2, 3, 7, 4]
. The union (|
) operator acts as a concise way to combine the arrays while automatically eliminating duplicate entries, providing a straightforward method to obtain an array with unique values.
Use the select
Method to Remove Duplicates From a Ruby Array
The select
method is typically used for filtering elements based on a given condition. However, it can also be leveraged to remove duplicates from a Ruby array by employing the with_index
method to keep only the first occurrence of each element.
Example Code:
original_array = [1, 2, 2, 3, 4, 4, 5]
unique_array = original_array.select.with_index { |e, i| original_array.index(e) == i }
print unique_array
Output:
[1, 2, 3, 4, 5]
In this code, we begin with an array named original_array
that contains various elements, including duplicates. The objective is to create a new array named unique_array
that retains only the first occurrence of each unique element.
We achieve this by using the select
method in combination with with_index
. The block within select
checks if the index of the first occurrence of each element is equal to the current index.
Consequently, only the elements that meet this condition are included in the unique_array
. The print unique_array
statement outputs the result, which is [1, 2, 3, 4, 5]
.
This approach effectively filters the original array, keeping only the first occurrence of each unique element in the new array.
Use the uniq
Method With a Block to Remove Duplicates From a Ruby Array
You can use the uniq
method with a block to customize the uniqueness comparison logic when removing duplicates from a Ruby array.
The block is applied to each element of the array, and elements are considered duplicates if the block returns the same value for them. This method provides a way to define custom logic for determining uniqueness, allowing for more flexibility in handling specific cases where the default equality comparison may not be sufficient.
Example Code:
ary = [1, 42, 42, 3, 4, 4, 5, 42]
unique_array = ary.uniq { |num| num == 42 ? 42 : num }
puts unique_array.inspect
Output:
[1, 42, 3, 4, 5]
In this code, we start with an array named ary
that contains various elements, including duplicates. The goal is to create a new array, unique_array
, by removing duplicates based on a specific condition.
We achieve this using the uniq
method with a block. The block compares each element to the number 42
, and if true, uses 42
as the key for uniqueness; otherwise, it uses the original element.
The resulting unique_array
is then printed using puts unique_array.inspect
. In this example, the output would be [1, 42, 3, 4, 5]
, illustrating the modified array with duplicates removed based on the specified condition in the block.
This method allows for a customized approach to handling duplicates during the uniqueness comparison process.
Conclusion
When working with Ruby arrays, the choice of method for removing duplicates depends on factors such as desired outcomes, modification preferences, and code readability. The uniq
method stands out as a straightforward and widely used approach, while alternative methods such as uniq!
, to_set
, set operations, and the select
method offer flexibility for specific scenarios.
Developers can select the most suitable method based on their unique requirements and coding style.