How to Merge Arrays in Ruby
-
Merge Arrays in Ruby Using the Array
concat()
Method -
Merge Arrays in Ruby Using the Concatenation Operator (
+
) -
Merge Arrays in Ruby Using the Array
push()
Method With Splatted Array -
Merge Arrays in Ruby Using the Array
append()
Method -
Merge Arrays in Ruby Using the Array
union()
Method -
Merge Arrays in Ruby Using the Array
|
Operator - Conclusion
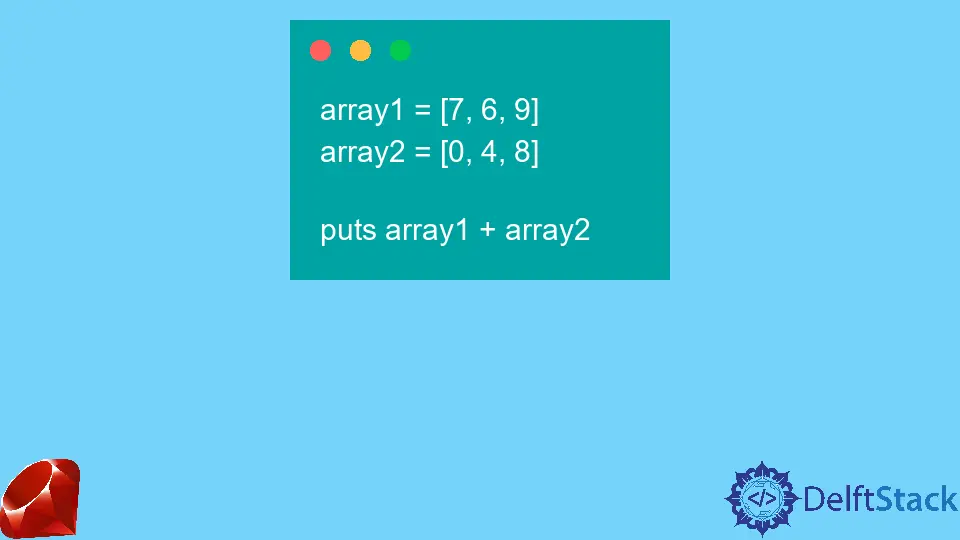
In Ruby programming, merging arrays is a common operation, whether you’re consolidating data, eliminating duplicates, or creating comprehensive datasets. Ruby provides a robust set of methods and operators, each tailored to specific needs, making the array merging process both flexible and powerful.
In this article, we’ll explore various techniques to merge arrays in Ruby, discussing the syntax, functionality, and use cases of methods such as concat()
, the +
operator, push()
with a splatted array, append()
, union()
, and the union operator (|
).
Merge Arrays in Ruby Using the Array concat()
Method
To merge two arrays in Ruby, we can use the concat()
method, which allows us to combine the elements of two arrays into a single array.
The concat()
method is invoked on an array and takes another array as an argument. The elements of the second array are then appended to the end of the first array.
It’s important to note that this method modifies the original array in place, making it a destructive operation.
The syntax of the concat()
method is as follows:
array1.concat(array2)
array1
: The array to which elements will be added.array2
: The array whose elements will be appended toarray1
.
Let’s delve into a complete working example to illustrate the usage of the concat()
method.
arr1 = [1, 2, 3]
arr2 = [4, 5, 6]
arr1.concat(arr2)
puts arr1
In the provided example, we have two arrays, arr1
and arr2
, each containing numerical elements. The concat()
method is then applied to arr1
, with arr2
as the argument.
This results in the modification of arr1
, where the elements of arr2
are added to the end of arr1
.
The key point here is that the concat()
method is destructive, meaning it alters the original array (arr1
), unlike non-destructive methods that create a new array. If preservation of the original array is desired, alternatives like the +
operator can be used for merging.
Output:
1
2
3
4
5
6
In this output, the elements of arr1
and arr2
have been successfully merged using the concat()
method. The resulting array contains all elements in the order they were added.
This method proves useful when you need to merge arrays without creating a new one and the specific use case requires modifying the original array in place.
Merge Arrays in Ruby Using the Concatenation Operator (+
)
The concatenation operator (+
) provides an alternative method for merging arrays. Unlike the destructive nature of the concat()
method, the +
operator creates a new array containing elements from both source arrays, leaving the original arrays unchanged.
The +
operator is applied between two arrays, combining their elements into a new array. Its syntax is as follows:
merged_array = array1 + array2
array1
: The first array whose elements will be included in the merged array.array2
: The second array whose elements will be appended to the merged array.
Let’s illustrate the usage of the +
operator with a practical example:
array1 = [1, 2, 3]
array2 = [4, 5, 6]
merged_array = array1 + array2
puts merged_array
In this example, the +
operator is used to merge the two arrays array1
and array2
, creating a new array, merged_array
. Unlike the concat()
method, this operation does not modify the original arrays (array1
and array2
).
The puts
statement is used to output the contents of the merged_array
. It’s crucial to understand that the +
operator, in this context, creates a new array rather than altering the existing ones.
This non-destructive behavior can be advantageous in scenarios where preserving the original arrays is essential.
Output:
1
2
3
4
5
6
Here, the +
operator successfully combines the elements from array1
and array2
into a new array (merged_array
). This method is particularly useful when you want to merge arrays without modifying the original ones.
Merge Arrays in Ruby Using the Array push()
Method With Splatted Array
In Ruby, the push()
method is commonly used to add elements to the end of an array. When combined with the splat operator (*
), which is used to expand the elements of another array, it becomes a powerful tool for merging arrays.
This method is non-destructive, as it adds elements to the end of an array without altering the original arrays. Its syntax is as follows:
array1.push(*array2)
array1
: The array to which elements will be added.array2
: The array whose elements will be appended toarray1
.
Let’s walk through a practical example showcasing the usage of the push()
method with a splatted array:
array1 = [1, 2, 3]
array2 = [4, 5, 6]
array1.push(*array2)
puts array1
In this example, we have two arrays, array1
and array2
, similar to the previous examples. The push()
method is applied to array1
, and the splat operator (*
) is used to expand the elements of array2
, appending them to the end of array1
.
Importantly, this operation does not modify the original arrays.
The puts
statement is utilized to output the contents of the merged array (array1
). Unlike the concat()
method, this approach is non-destructive, making it useful when you want to combine arrays without altering the source arrays.
Output:
1
2
3
4
5
6
Here, the push()
method with a splatted array successfully combines the elements from array1
and array2
. The resulting array (array1
) contains all the elements in the desired order.
Merge Arrays in Ruby Using the Array append()
Method
The append()
method is a convenient way to add elements to an array. Introduced in Ruby 2.6 and later versions, it can be used to merge arrays effectively.
Similar to the push()
method, append()
adds elements to the end of an array, but it offers a more descriptive name for this operation.
The append()
method takes one or more arguments, which can include individual elements or another array. Below is its syntax:
array1.append(*array2)
array1
: The array to which elements will be added.array2
: The array whose elements will be appended toarray1
.
Let’s dive into a complete working example illustrating the usage of the append()
method:
array1 = [1, 2, 3]
array2 = [4, 5, 6]
array1.append(*array2)
puts array1
Here, we use the append()
method employed on array1
, and the splat operator (*
) is used to expand the elements of array2
, appending them to the end of array1
. This operation does not modify the original arrays, maintaining their integrity.
The puts
statement is used to output the contents of the merged array (array1
). Like the push()
method with a splatted array, using append()
for array merging is also a non-destructive approach, making it a suitable choice when you want to combine arrays without changing the original arrays.
Output:
1
2
3
4
5
6
As you can see, the append()
method with a splatted array successfully combines the elements from array1
and array2
.
Merge Arrays in Ruby Using the Array union()
Method
The union()
method is another powerful tool for merging arrays while ensuring uniqueness of elements.
The union()
method is invoked on an array and takes another array as an argument. It returns a new array containing elements from both arrays, excluding duplicates.
It provides a clear and concise syntax for merging arrays with unique elements:
merged_array = array1.union(array2)
array1
: The first array whose elements will be included in the merged array.array2
: The second array whose elements will be appended to the merged array.
Let’s explore a complete working example to showcase the usage of the union()
method:
array1 = [1, 2, 3]
array2 = [3, 4, 5]
merged_array = array1.union(array2)
puts merged_array
In this example, the union()
method is applied to array1
, taking array2
as an argument. This operation creates a new array, merged_array
, which contains unique elements from both arrays, ensuring that duplicates are excluded.
The puts
statement is utilized to output the contents of the merged array (merged_array
).
It’s important to note that the union()
method is non-destructive, meaning it does not modify the original arrays (array1
and array2
). Instead, it creates a fresh array with unique elements from both source arrays.
Output:
1
2
3
4
5
Here, the union()
method successfully combines the elements from array1
and array2
into a new array (merged_array
). The resulting array contains all unique elements in the order they appear in the original arrays.
This method is particularly useful when you want to merge arrays while ensuring that each element appears only once in the resulting array.
Merge Arrays in Ruby Using the Array |
Operator
The |
operator, also known as the union operator, provides a concise and intuitive way to merge arrays while ensuring unique elements in the resulting array. This operator creates a new array by combining elements from two arrays, excluding duplicates.
Its syntax is as follows:
merged_array = array1 | array2
array1
: The first array whose elements will be included in the merged array.array2
: The second array whose elements will be appended to the merged array.
Let’s dive into a complete working example to illustrate the usage of the |
operator:
array1 = [1, 2, 3]
array2 = [3, 4, 5]
merged_array = array1 | array2
puts merged_array
In this example, we applied the |
operator between the two arrays array1
and array2
. This operation creates a new array, merged_array
, containing unique elements from both arrays while excluding duplicates.
The puts
statement is used to output the contents of the merged array (merged_array
).
It’s essential to note that the |
operator is non-destructive, meaning it does not modify the original arrays (array1
and array2
). Instead, it produces a fresh array with unique elements.
Output:
1
2
3
4
5
The union operator (|
) successfully combines the elements from array1
and array2
into a new array (merged_array
). The resulting array contains all unique elements from both source arrays (in the order they appear).
This method is particularly useful when you want to merge arrays while ensuring each element appears only once in the resulting array.
Conclusion
Ruby provides a versatile set of methods and operators for efficiently merging arrays based on specific requirements, whether you choose the concat()
method, the +
operator, the push()
method with a splatted array, the append()
method, the union()
method, or the union operator (|
), each approach offers a unique advantage in array manipulation.
Understanding the syntax, behavior, and use cases of these methods allows you to tailor your array merging strategies to meet the demands of your code. The ability to combine arrays while considering factors like preservation of original content, uniqueness, and clarity is a valuable skill in Ruby programming.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn