How to Square Array Element in Ruby
-
Method 1: Using the
map
Method -
Method 2: Using the
each
Method -
Method 3: Using
each_with_index
-
Method 4: Using
inject
- Conclusion
- FAQ
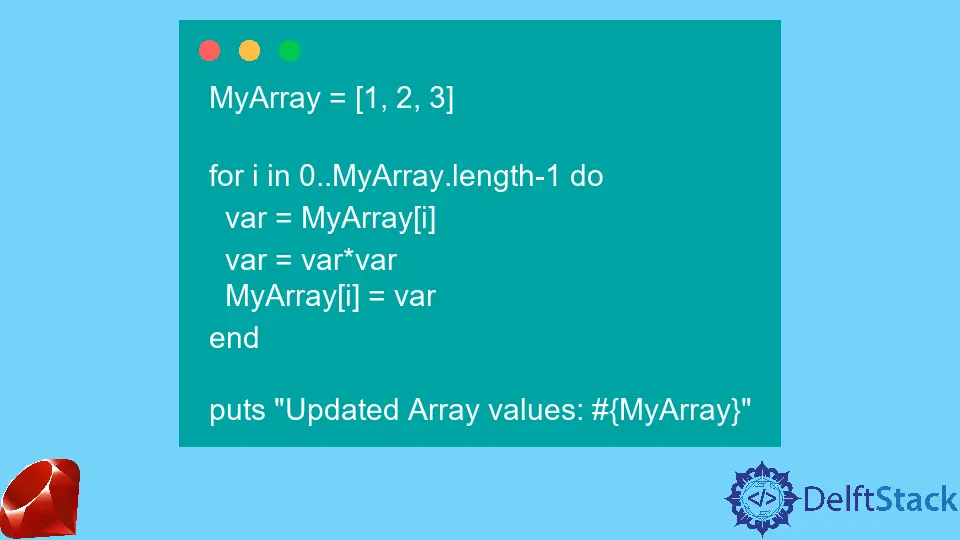
In the world of programming, manipulating arrays is a common task. If you’re working with Ruby and need to square each element in an array, you’re in the right place.
This tutorial will guide you through various methods to achieve this, from using built-in methods to more manual iterations. Whether you’re a beginner or an experienced developer, understanding how to square array elements in Ruby can enhance your coding skills. We’ll explore different techniques, providing clear examples and explanations along the way. By the end of this article, you’ll have a solid grasp of how to square array elements in Ruby, making your programming tasks smoother and more efficient.
Method 1: Using the map
Method
One of the most elegant ways to square each element in an array in Ruby is by using the map
method. This method is part of Ruby’s Enumerable module, which allows you to transform elements in an array easily. It creates a new array containing the results of running the block once for every element in the original array.
Here’s how you can use the map
method to square the elements of an array:
numbers = [1, 2, 3, 4, 5]
squared_numbers = numbers.map { |number| number ** 2 }
Output:
[1, 4, 9, 16, 25]
In this example, we start with an array called numbers
, which contains integers from 1 to 5. The map
method iterates through each element, applying the block of code that squares the number (number ** 2
). The result is a new array, squared_numbers
, that contains the squared values. This method is concise and leverages Ruby’s expressive syntax, making it a favorite among developers.
Method 2: Using the each
Method
If you prefer a more manual approach, you can use the each
method to iterate through the array and square each element. This method allows you to perform operations on each element without creating a new array right away.
Here’s how you can square array elements using each
:
numbers = [1, 2, 3, 4, 5]
squared_numbers = []
numbers.each do |number|
squared_numbers << number ** 2
end
Output:
[1, 4, 9, 16, 25]
In this example, we initialize an empty array called squared_numbers
. As we iterate through the numbers
array using each
, we square each number and append it to the squared_numbers
array using the <<
operator. This method provides more control over the process, allowing you to add additional logic if needed. While it may be slightly more verbose than using map
, it can be beneficial in scenarios where you want to perform multiple operations or checks.
Method 3: Using each_with_index
For situations where you might need the index of each element while squaring it, each_with_index
is a great option. This method allows you to access both the element and its index, which can be useful for more complex operations.
Here’s how you can use each_with_index
to square array elements:
numbers = [1, 2, 3, 4, 5]
squared_numbers = []
numbers.each_with_index do |number, index|
squared_numbers[index] = number ** 2
end
Output:
[1, 4, 9, 16, 25]
In this case, we again start with an empty array, squared_numbers
. The each_with_index
method allows us to iterate through the numbers
array while providing access to the current index. We square each number and assign it directly to the corresponding index in the squared_numbers
array. This method is particularly useful when you need to maintain the original index positions of the elements, ensuring that the output array aligns perfectly with the input.
Method 4: Using inject
Another powerful method in Ruby is inject
, which can be used to accumulate results in a more functional programming style. This method is particularly useful for reducing an array to a single value, but it can also be adapted to create a new array of squared values.
Here’s how you can use inject
to square array elements:
numbers = [1, 2, 3, 4, 5]
squared_numbers = numbers.inject([]) do |result, number|
result << number ** 2
end
Output:
[1, 4, 9, 16, 25]
In this example, we start with an empty array passed as an argument to inject
. The block takes two parameters: result
, which accumulates the squared values, and number
, which represents the current element in the iteration. Inside the block, we square the number and append it to the result
array. This method showcases Ruby’s flexibility and allows for a more functional approach to array manipulation.
Conclusion
Squaring array elements in Ruby can be accomplished through various methods, each with its unique advantages. Whether you opt for the concise elegance of map
, the manual control of each
, the indexed approach of each_with_index
, or the functional style of inject
, Ruby provides you with powerful tools to achieve your goals. By mastering these techniques, you’ll be better equipped to handle array manipulations in your Ruby applications. So, dive in, experiment with these methods, and enhance your Ruby programming skills!
FAQ
-
What is the fastest way to square elements in an array in Ruby?
The fastest method is typically using themap
method, as it is optimized for transforming arrays. -
Can I square elements in a multi-dimensional array?
Yes, you can use nested iterations with methods likemap
oreach
to access and square elements in a multi-dimensional array. -
Are there any performance considerations when squaring large arrays?
For large arrays, usingmap
orinject
is generally more efficient. However, performance can vary based on Ruby versions and the specific operations being performed. -
Can I square elements conditionally in Ruby?
Yes, you can use conditional statements within your iteration methods to square elements based on specific criteria. -
What if I want to square elements and store them in the original array?
You can use theeach_with_index
method to modify the original array directly by assigning squared values back to their respective indices.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn