How to Combine Array to String in Ruby
-
Using the
join
Method -
Using
reduce
for Custom Concatenation - Using String Interpolation
- Conclusion
- FAQ
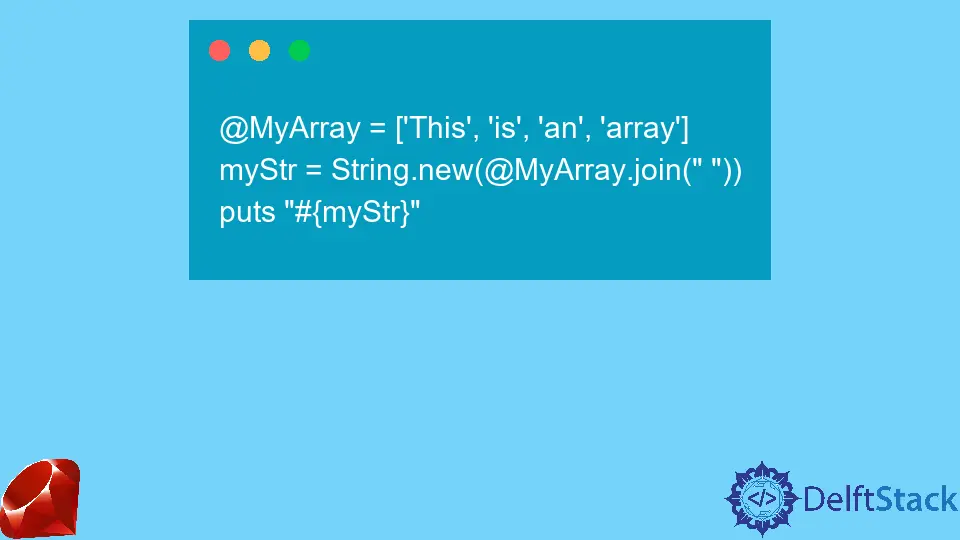
Combining arrays into strings is a common task in programming, and Ruby makes it particularly easy with its built-in methods. Whether you’re working with a list of names, numbers, or any other data type, you might find yourself needing to convert an array into a single string.
This tutorial will discuss how to combine an array to a string in Ruby, exploring various methods to achieve this. By the end, you’ll have a solid understanding of how to manipulate strings and arrays in Ruby, enhancing your coding skills and efficiency. So, let’s dive into the different ways to combine arrays into strings!
Using the join
Method
One of the simplest and most effective ways to combine an array into a string in Ruby is by using the join
method. This method takes an array and concatenates its elements into a single string, separated by a specified delimiter. If no delimiter is provided, it will default to an empty string.
Here’s how you can use the join
method:
names = ["Alice", "Bob", "Charlie"]
result = names.join(", ")
puts result
Output:
Alice, Bob, Charlie
The join
method is straightforward. In this example, we have an array called names
containing three string elements. By calling join(", ")
, we specify that each name should be separated by a comma and a space when combined into a single string. The result is a clean, readable string that can be easily used in output or further processing.
This method is not only efficient but also flexible. You can change the delimiter to any character or string you prefer, such as a hyphen, space, or even a newline character. This versatility makes join
an essential tool for Ruby developers when handling string manipulation.
Using reduce
for Custom Concatenation
Another effective method for combining an array into a string is using the reduce
method. This method is particularly useful when you want more control over the concatenation process or when you need to apply additional logic during the combination.
Here’s an example of how to use reduce
:
words = ["Hello", "world", "from", "Ruby"]
result = words.reduce("") do |accumulator, word|
accumulator.empty? ? word : "#{accumulator} #{word}"
end
puts result
Output:
Hello world from Ruby
In this example, we have an array called words
containing several string elements. The reduce
method iterates over each element, allowing us to build a string incrementally. We start with an empty string as the initial value. For each word in the array, we check if the accumulator is empty. If it is, we simply return the current word; otherwise, we concatenate it to the accumulator with a space in between.
This approach offers flexibility in how you build your final string. You can easily modify the logic to add different separators, apply transformations to each word, or even filter out certain elements based on specific conditions. The reduce
method is powerful and can be adapted for various use cases, making it an excellent choice for more complex string combinations.
Using String Interpolation
String interpolation is another handy technique in Ruby that allows you to combine arrays into strings. While it’s not a dedicated method like join
or reduce
, it can be very effective when you want to format your output dynamically.
Here’s how you can use string interpolation to combine an array:
fruits = ["apple", "banana", "cherry"]
result = "#{fruits.join(', ')} are delicious."
puts result
Output:
apple, banana, cherry are delicious.
In this example, we have an array called fruits
. By using string interpolation, we can embed the output of the join
method directly within a larger string. This allows us to create a complete sentence that includes the combined fruits followed by a statement about their deliciousness.
String interpolation is not only concise but also enhances readability. It allows you to create complex strings without the need for extensive concatenation, making your code cleaner and easier to maintain. This method is particularly useful when you want to include array elements in a more complex string structure.
Conclusion
Combining arrays into strings in Ruby is a fundamental skill that can greatly enhance your programming capabilities. Whether you choose to use the join
method for its simplicity, the reduce
method for its flexibility, or string interpolation for dynamic formatting, each technique has its strengths. By mastering these methods, you can efficiently manipulate strings and arrays, making your code more effective and easier to read. As you continue to explore Ruby, these techniques will serve as essential tools in your development toolkit.
FAQ
- What is the difference between
join
andreduce
in Ruby?
Thejoin
method combines array elements into a string with a specified delimiter, whilereduce
allows for more complex concatenation logic and customization.
-
Can I use
join
without a delimiter?
Yes, if you calljoin
without any arguments, it will concatenate the elements without any separator. -
Is string interpolation the same as concatenation?
No, string interpolation is a way to embed variable values directly within a string, while concatenation involves combining strings using operators like+
. -
Are there performance differences between these methods?
Generally,join
is more efficient for simple concatenation, whilereduce
may have a slight overhead due to its iterative nature, especially for large arrays. -
Can I combine arrays of different data types into a string?
Yes, Ruby allows you to combine arrays with mixed data types using methods likejoin
, but you may need to convert non-string elements to strings first.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - Ruby Array
- %i and %I in Ruby
- How to Square Array Element in Ruby
- How to Merge Arrays in Ruby
- How to Convert Array to Hash in Ruby
- How to Remove Duplicates From a Ruby Array