How to Get Substring in Ruby
-
the
substring
Method in Ruby - Extracting a Substring in Ruby
- Use the Index Method for Substring Removal or Replacement in Ruby
- Conclusion
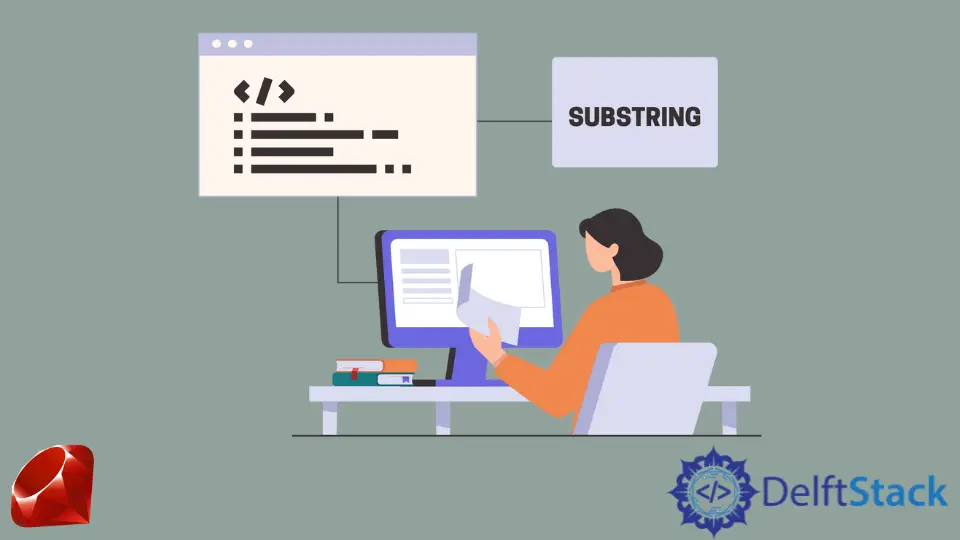
A string carries an arbitrary sequence of bytes and operates on this sequence and is one of the basic elements of all programming languages. Being a part of the basic building of any program, one should have a grip on working with strings and know how to perform complex operations using strings.
We will introduce in this article how to create a substring from a string in Ruby with examples.
the substring
Method in Ruby
A substring is a range or specific array of characters taken from an existing string. Operating a substring is quite an appropriate approach when the user wants to work on a specific part of the string (like the initial, final, and middle part of the string).
There is no substring
method in Ruby, and hence, we rely upon ranges and expressions. If we want to use the range, we have to use periods between the starting and ending index of the substring to get a new substring from the main string.
We can also use commas (,
) between 2 numbers only if we want to use the starting point of the substring. The second number will represent the number of elements we want to have in our substring from the starting point.
For a range example, let’s first examine the string a "virus"
, which has 5 characters from 0 to 4. If we want to get a substring in a reverse manner, we can use the negative end on a range, and we count backward from the length of the string.
Let’s discuss in the following section the methods on how to get a substring in Ruby.
Use Period (..
) Ranges to Get Substring in Ruby
Dots (.
) can be used as the period characters in Ruby, and we can use the period characters within ranges. We can use 2 or 3 periods.
Unlike Python, we cannot use colons (:
) inside the ranges. Instead, we can use period (.
) ranges.
As shown below, let’s use the above scenario to get a substring in Ruby.
Code:
StringForSub = 'virus'
StartSub = StringForSub[0..3]
MidSub = StringForSub[2..3]
EndSub = StringForSub[3..-1]
puts StartSub
puts MidSub
puts EndSub
In the code above, we initialize the string variable StringForSub
with the value 'virus'
and create three substrings using different index ranges from the original string. StartSub
includes characters from index 0 to 3, representing the first four characters.
The MidSub
encompasses characters from index 2 to 3, capturing the middle part. EndSub
starts from index 3 to the end, effectively extracting the last three characters.
Lastly, we use puts
statements to output each substring to the console.
Output:
The above output makes it easy to create substrings from the main string using the indexing method.
Use Commas (,
) to Get Substring in Ruby
If we want to use commas (,
) inside the ranges, we use 2 numbers. The first will be the starting point of the new substring, and the second number will be the length of the substring.
This next example uses the substring comma syntax in Ruby.
Code:
mainString = 'Ruby'
endSub = mainString[1, 3]
puts endSub
midSub = mainString[1, 2]
puts midSub
In the code above, we set the string variable mainString
to 'Ruby'
and generate two substrings using the string element reference []
method. The endSub
is extracted from index 1, including the subsequent 3 characters ('uby'
), which we output using the puts
statement.
Similarly, midSub
is created from index 1, encompassing the next 2 characters ('ub'
), and displayed using puts
.
Output:
The output shows the efficient use of the string element reference method in Ruby to extract specific substrings based on index and length parameters.
We can also use commas (,
) inside the indexing method to get a range by providing start and ending values for our new substring.
Extracting a Substring in Ruby
Any malicious code can be avoided by locating and sanitizing input from users by locating a specific substring among strings. A substring can be located by using different methods, such as:
-
Ruby
include?()
method. -
RegEx method.
-
Ruby String Element Reference
[]
.
the Ruby include?()
Method
The include?()
method is used to check if a substring is present within a string. This built-in method is the easiest method to locate a substring.
Basic Syntax:
string_to_search.include?(substring)
In the syntax, string_to_search
is the main string you want to search within, and substring
is the substring you want to check for. The method returns true
if the substring is found within the string and false
otherwise.
Let’s have an example in which we will use a string as information input by the user to check if the content contains any spam element or not.
Code:
mainStr = 'User submitted this content. It is a spam'
if mainStr.include?('spam')
puts 'Spam Content! Content Deleted'
else
puts 'Content Saved'
end
In the code above, we check the presence of the substring 'spam'
in the string variable mainStr
using the include?
method. If the substring is found, we output 'Spam Content! Content Deleted'
within the if
block; otherwise, we output 'Content Saved'
within the else
block.
Output:
The output is determined by the presence or absence of the word 'spam'
in the main string, allowing us to identify and manage potential spam content efficiently.
It can detect the substring from the main string, but one thing to remember is that it is a case-sensitive method.
Since the method’s return value is a Boolean value (true
or false
) indicating if the substring is available or not, respectively; therefore, we implement an if-else
statement to act accordingly.
A disadvantage of using the include?
method is that it is case-sensitive, and spam can return a false
statement. It is essential to convert the whole string into uppercase or lowercase and then locate a specific substring within a string.
In order to convert the whole string into uppercase, the method is as follows.
Code - Using upcase
:
mainStr = 'User submitted this content. It is a SPAM!'
if mainStr.include?('spam'.upcase)
puts 'Spam Content! Content Deleted'
else
puts 'Content Saved'
end
In the code above, we check if the uppercase version of the substring 'spam'
is present in the string variable mainStr
using the include?
method. If found, we output 'Spam Content! Content Deleted'
; otherwise, we output 'Content Saved'
.
Output:
The output is determined by the presence or absence of the uppercase word 'SPAM'
in the main string, enabling us to efficiently identify and handle potential spam content.
Let’s imagine the same scenario, but we have to use the lowercase.
Code - Using downcase
:
mainStr = 'User submitted this content. It is a SPAM!'
if mainStr.include?('spam'.downcase)
puts 'Spam Content! Content Deleted'
else
puts 'Content Saved'
end
In the code, we check for the presence of the lowercase version of the substring 'spam'
in the string variable mainStr
using the include?
method. If found, we output 'Spam Content! Content Deleted'
; otherwise, we output 'Content Saved'
.
Output:
The output depends on whether the lowercase word 'spam'
is present in the main string, allowing us to efficiently identify and manage potential spam content.
As you can see, we can use substrings to make our system secure by checking for the substrings from the submitted data for spam or some other type of content that we don’t want to be saved inside our application.
the RegEx Method in Ruby
Ruby returns nil
using this method if the specific substring is not found within the string. In this method, a simple regular expression is used to check if the string the specified regular expression pattern spam
.
Code:
mainStr = 'User submitted this content. It is a spam!'
puts mainStr =~ /spam/
In the code above, we use the =~
operator with the regular expression /spam/
to find the index of the first occurrence of the pattern 'spam'
in the string variable mainStr
.
Output:
The output, which is the index where 'spam'
is first found, is 37
in this case. This code efficiently identifies the position of the specified substring within the string.
We can also find the required substring from a string using the RegEx method and return the substring’s location inside a string. If the substring isn’t found, it will return nil
.
String Element Reference []
Method in Ruby
The Ruby string reference method passes either a string, an index value, or a regular expression inside a pair of square brackets. In Ruby, you can use the string element reference []
method to extract a substring from a string based on its index or a range of indices.
Basic Syntax:
substring = main_string[start_index, length]
In the syntax, the main_string
is the original string from which you want to extract a substring. The start_index
is the index in the string where the extraction should begin.
The length
(optional) is the number of characters to extract. If not provided, it will extract characters from the start_index
to the end of the string.
Code:
mainStr = 'spam'
puts mainStr['spam']
puts mainStr['[not-spam]']
In the code above, we initialize the string variable mainStr
with the value 'spam'
. We utilize the regular expression syntax /spam/
with the string element reference []
method to extract a substring from the main string that matches the specified pattern 'spam'
.
Output:
The output is 'spam'
, indicating that the substring 'spam'
is found in the main string. This code succinctly demonstrates the use of regular expressions to efficiently extract substrings from a string based on a specified pattern.
Use the Index Method for Substring Removal or Replacement in Ruby
If there is a need to replace or remove a certain substring from the main string, we can use the simple assigning method. We can use the index method to change the value.
Code:
mainStr = 'Hello World!'
mainStr[0..4] = 'Bye'
puts mainStr
In the code above, we have a string variable mainStr
initialized with the value 'Hello World!'
. We use indexing and assignment to replace a portion of the main string.
Specifically, we replace characters from index 0 to 4 (inclusive) with the new string 'Bye'
.
Output:
The output is 'Bye World!'
, demonstrating that the original substring 'Hello'
has been replaced with 'Bye'
. This code succinctly illustrates how to modify a portion of a string using indexing and assignment in Ruby.
Use the indexing method to replace or remove a certain part of the main string by just assigning the value to the part of the string.
Conclusion
In conclusion, understanding how to manipulate and extract substrings from strings is crucial for effective programming. Strings, as fundamental elements in programming languages, play a vital role in various operations.
In this article, we introduced methods for creating substrings in Ruby, focusing on the absence of a direct substring method and relying on ranges, expressions, and indexing. We explored the use of period (..
) ranges and commas ('start, length'
) for substring extraction, providing examples to illustrate their application.
Additionally, we discussed the significance of substring extraction in real-world scenarios, such as detecting and handling spam content in user-submitted data. By utilizing methods like include?()
, regular expressions, and the string element reference []
, we can efficiently locate and manage substrings within strings.
Overall, mastering substring manipulation in Ruby enhances one’s ability to build secure and functional applications.