How to Iterate Through a Ruby Array
-
Iterate Through a Ruby Array Using the
each
Method -
Iterate Through a Ruby Array Using the
for
Loop -
Iterate Through a Ruby Array Using the
reverse_each
Method -
Iterate Through a Ruby Array Using the
each_with_index
Method -
Iterate Through a Ruby Array Using the
map
Method -
Iterate Through a Ruby Array Using the
select
Method -
Iterate Through a Ruby Array Using the
reject
Method - Conclusion
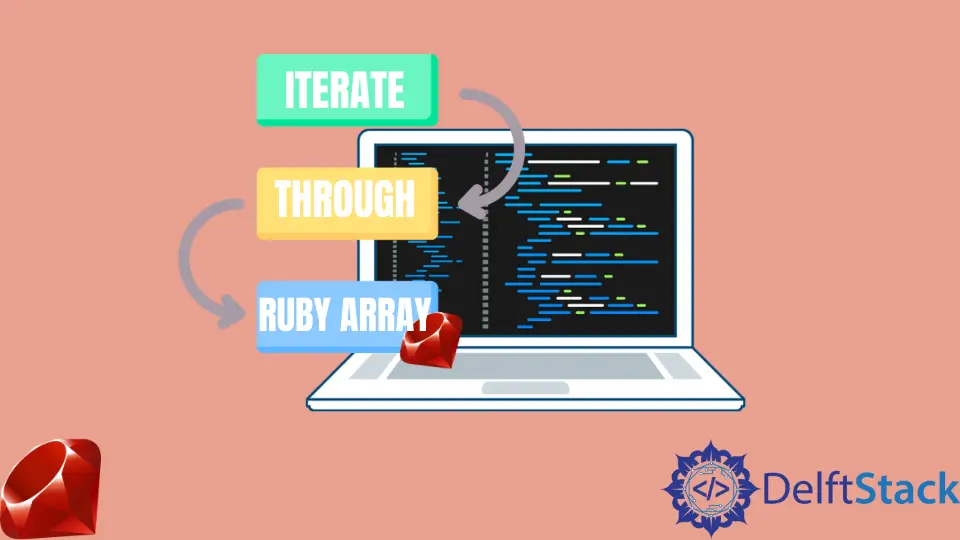
The array
class in Ruby includes the Enumerable mixin
, and as a result, it has several transversal methods.
In this tutorial, we will be looking at these enumerable methods and other ways of iterating through an array in Ruby.
Iterate Through a Ruby Array Using the each
Method
The each
method in Ruby is an enumerable method used for iterating through elements in an array. It allows you to perform a specific action for each element by providing a block of code.
During each iteration, the block is executed with the current element as an argument. This is the most used iterator in Ruby.
Example:
fruits = %w[Orange Apple Banana]
fruits.each do |fruit|
puts fruit
end
Output:
Orange
Apple
Banana
In the code, we initialize an array named fruits
containing three string elements: "Orange," "Apple," and "Banana"
. We then utilize the each
method, iterating through each element of the fruits
array.
Within the do-end
block, we print each fruit. As a result, the output displays each fruit on a new line.
Iterate Through a Ruby Array Using the for
Loop
The for
loop can be used to iterate through elements in an array in Ruby. The loop iterates over each element of the array, and a block of code is executed for each iteration.
Example:
fruits = %w[Orange Apple Banana]
for fruit in fruits
puts fruit
end
Output:
Orange
Apple
Banana
In the following code, we initialize an array named fruits
containing three string elements. Subsequently, we employ a for
loop to iterate through each element of the fruits
array.
Inside the loop, we use the puts
statement to print each fruit to the console. It’s important to note that the use of the for
loop in Ruby can have unintended consequences, such as potential scoping issues, and in this case, the loop iterates through each fruit, printing them on separate lines.
Although using a for
loop is an effective way to iterate through an array, this method can have an undesirable side-effect, and as a result, it’s not recommended.
As an example, if a fruit
variable had earlier been defined in the code snippet above, the for
loop would overwrite it with the last element of the fruits
array. It is a scoping issue, and the behavior is illustrated below.
Example:
fruit = 'Mango'
fruits = %w[Orange Apple Banana]
for fruit in fruits
puts fruit
end
puts fruit
Output:
Orange
Apple
Banana
Banana
In this code, we use a for
loop to iterate through each element of the fruits
array. Inside the loop, the puts
statement prints each fruit to the console.
However, a notable behavior of the for
loop in Ruby is that it reassigns the variable used for iteration, in this case, fruit
, potentially causing unexpected results. As a consequence, when we subsequently use puts fruit
outside the loop, it prints the last element of the array, "Banana"
, as the loop has overwritten the original value of fruit
.
Iterate Through a Ruby Array Using the reverse_each
Method
As the name implies, this method works like the each
method but in reverse order.
In Ruby, the reverse_each
method is an enumerable method used to iterate through elements in an array in reverse order. It is similar to the each
method but iterates from the last element to the first.
This method is useful when you need to perform actions on array elements in reverse sequence.
Example:
fruits = %w[Orange Apple Banana]
fruits.reverse_each do |fruit|
puts fruit
end
Output:
Banana
Apple
Orange
In this code, we initialize an array named fruits
containing three string elements. Subsequently, we use the reverse_each
method to iterate through the elements of the fruits
array in reverse order.
Inside the block associated with reverse_each
, we use the puts
statement to print each fruit to the console. As a result, the output displays each fruit on a new line in reverse order.
Iterate Through a Ruby Array Using the each_with_index
Method
The each_with_index
method in Ruby is an enumerable method used for iterating through elements in an array while also providing the index of each element during each iteration. This method is useful in a situation where you need to get each element of an array and the index.
Example:
fruits = %w[Orange Apple Banana]
fruits.each_with_index do |fruit, index|
puts "#{index + 1}. #{fruit}"
end
Output:
1. Orange
2. Apple
3. Banana
In the following code, we use the each_with_index
method to iterate through the elements of the fruits
array. Within the associated block, we use the puts
statement to print each fruit along with its corresponding index.
The expression "#{index + 1}. #{fruit}"
combines the index (adjusted by adding 1
for human-readable numbering) and the fruit
, creating a formatted output. Consequently, the output displays each fruit with its index, starting from 1
.
Although the above example demonstrates the simplest way of iterating through an array, this method usually comes in handy when you need to traverse through an array and carry out some operations on each of its elements - for example, iterating through a list of email addresses and sending a message to each of them.
Iterate Through a Ruby Array Using the map
Method
Note: This method, as well as the next ones, is a bit different from the ones mentioned above in the sense that they transform an array into another one.
The map
method is an enumerable method used for iterating through elements in an array and creating a new array based on the results of applying a given block of code to each element. This method is useful if you want to modify each element of an array and have them as a new array.
Example:
numbers = [2, 4, 6]
doubles = numbers.map do |n|
n * 2
end
puts doubles
Output:
4
8
12
In this code, we define an array named numbers
containing three integer elements. We then utilize the map
method to iterate through each element of the numbers
array, doubling each value in the process.
The block within the map
method, represented by do |n|
, computes the doubled value for each element. The result is a new array named doubles
that stores the doubled values.
Finally, the puts
statement prints the contents of the doubles
array to the console. Consequently, the output displays each doubled value on a new line.
Bear in mind that there is also a collect
method in Ruby, an alias to map
, and both behave the same way.
Iterate Through a Ruby Array Using the select
Method
As the name implies, the select
method allows you to pick only specific elements of an array that meet a specific logical condition. It evaluates a block of code for each element and includes only those for which the block returns true
in the resulting array.
This method also produces a new array.
Example:
numbers = [2, 3, 4, 5, 6, 7, 8, 9]
perfect_squares = numbers.select do |n|
(Math.sqrt(n) % 1).zero?
end
puts perfect_squares
Output:
4
9
In this code, we start with an array named numbers
containing eight integer elements. We then utilize the select
method to iterate through each element of the numbers
array.
Inside the block specified by do |n|
, we use the Math.sqrt
function to calculate the square root of each number, and the condition Math.sqrt(n) % 1 == 0
checks if the square root is a whole number. The select
method filters the elements that satisfy this condition, creating a new array named perfect_squares
.
Finally, the puts
statement prints the contents of the perfect_squares
array to the console. Note that there is also a find_all
method which is an alias to select
, and both behave the same way.
Iterate Through a Ruby Array Using the reject
Method
In Ruby, the reject
method is an enumerable method used for iterating through elements in an array and creating a new array that excludes elements satisfying a specified condition. This method is the opposite of select
, and it’s useful when you need to reject some specific elements of an array and return the remaining ones as a new array.
Example:
numbers = [-2, -1, 0, 1, 2, 3]
positive_integers = numbers.reject do |n|
n < 1
end
puts positive_integers
Output:
1
2
3
In this code, we initialize an array named numbers
containing six integer elements, including both positive and negative values. The reject
method is then employed to iterate through each element of the numbers
array.
Within the block specified by do |n|
, the condition n < 1
is evaluated to identify elements that are not positive integers. The reject
method filters out elements that meet this condition, creating a new array named positive_integers
.
Lastly, we print the contents of the positive_integers
array to the console. This showcases how the reject
method effectively excludes elements based on a specified logical condition, providing a streamlined way to obtain a modified array.
Conclusion
This Ruby tutorial covered essential array iteration methods in Ruby, emphasizing the use of enumerable methods and providing insights into their practical applications. The each
method proved fundamental for basic iteration, while caution was advised regarding the use of the for
loop due to potential scoping issues.
The reverse_each
method facilitated reverse order iteration, and each_with_index
was highlighted for scenarios requiring both element values and their indices. Additionally, the transformative nature of the map
method was discussed, along with methods like select
and reject
for filtering elements based on specific conditions.
The article aimed to provide a comprehensive guide for developers seeking efficient and expressive ways to manipulate arrays in Ruby.