遍歷 Ruby 陣列
Nurudeen Ibrahim
2023年1月30日
Ruby
Ruby Array
-
使用
each
方法遍歷 Ruby 陣列 -
使用
for
迴圈遍歷 Ruby 陣列 -
使用
reverse_each
方法遍歷 Ruby 陣列 -
使用
each_with_index
方法遍歷 Ruby 陣列 -
使用
map
方法遍歷 Ruby 陣列 -
使用
select
方法遍歷 Ruby 陣列 -
使用
reject
方法遍歷 Ruby 陣列
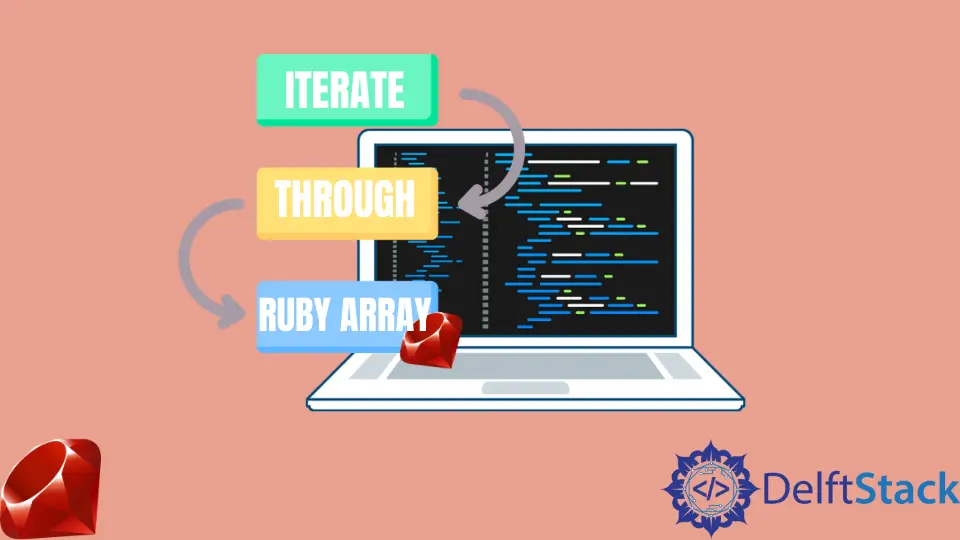
Ruby 中的陣列類包含 Enumerable mixin,因此它有幾個橫向方法。在本教程中,我們將研究這些可列舉的方法和其他在 Ruby 中遍歷陣列的方法。
使用 each
方法遍歷 Ruby 陣列
這是 Ruby 中最常用的迭代器。
示例程式碼:
fruits = ['Orange', 'Apple', 'Banana']
fruits.each do |fruit|
puts fruit
end
輸出:
Orange
Apple
Banana
使用 for
迴圈遍歷 Ruby 陣列
此方法可能會產生不良副作用,因此不推薦使用。
示例程式碼:
fruits = ['Orange', 'Apple', 'Banana']
for fruit in fruits
puts fruit
end
輸出:
Orange
Apple
Banana
如果在上面的示例中早先定義了一個 fruit
變數,for
迴圈將用 fruits
陣列的最後一個元素覆蓋它。這是一個範圍界定問題,其行為如下所示。
示例程式碼:
fruit = 'Mango'
fruits = ['Orange', 'Apple', 'Banana']
for fruit in fruits
puts fruit
end
puts fruit
輸出:
Orange
Apple
Banana
Banana
使用 reverse_each
方法遍歷 Ruby 陣列
顧名思義,這與 each
方法類似,但順序相反。
示例程式碼:
fruits = ['Orange', 'Apple', 'Banana']
fruits.reverse_each do |fruit|
puts fruit
end
輸出:
Banana
Apple
Orange
使用 each_with_index
方法遍歷 Ruby 陣列
此方法在你需要獲取陣列的每個元素和索引的情況下很有用。
示例程式碼:
fruits = ['Orange', 'Apple', 'Banana']
fruits.each_with_index do |fruit|
puts "#{index}. #{fruit}"
end
輸出:
0. Orange
1. Apple
2. Banana
儘管上面的示例演示了遍歷陣列的最簡單方法,但是當你需要遍歷陣列並對其中的每個元素執行一些操作時,這些方法通常會派上用場 - 例如,遍歷電子郵件地址列表和向他們每個人傳送訊息。
我們將在本教程中提到的其餘方法與上面提到的方法有些不同,它們將一個陣列轉換為另一個陣列。
使用 map
方法遍歷 Ruby 陣列
如果你想修改陣列的每個元素並將它們作為新陣列,這很有用。
示例程式碼:
numbers = [2, 4, 6]
doubles = numbers.map do |n|
n * 2
end
puts doubles
輸出:
[4, 8, 12]
請注意,還有一個 collect
方法,map
的別名,它們的行為方式相同。
使用 select
方法遍歷 Ruby 陣列
顧名思義,select
方法允許你僅選擇陣列中滿足特定邏輯條件的特定元素。它還會生成一個新陣列。
示例程式碼:
numbers = [2, 3, 4, 5, 6, 7, 8, 9]
perfect_squares = numbers.select do |n|
Math.sqrt(n) % 1 == 0
end
puts perfect_squares
輸出:
[4, 9]
請注意,還有一個 find_all
方法,它是 select
的別名,它們的行為方式相同。
使用 reject
方法遍歷 Ruby 陣列
reject
方法與 select
方法相反,當你需要拒絕陣列的某些特定元素並將其餘元素作為新陣列返回時,它很有用。
示例程式碼:
numbers = [-2, -1, 0, 1, 2, 3]
positive_integers = numbers.reject do |n|
n < 1
end
puts positive_integers
輸出:
[1, 2, 3]
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe