在 Ruby 中使用索引對映陣列
Nurudeen Ibrahim
2023年1月30日
Ruby
Ruby Array
Ruby Map
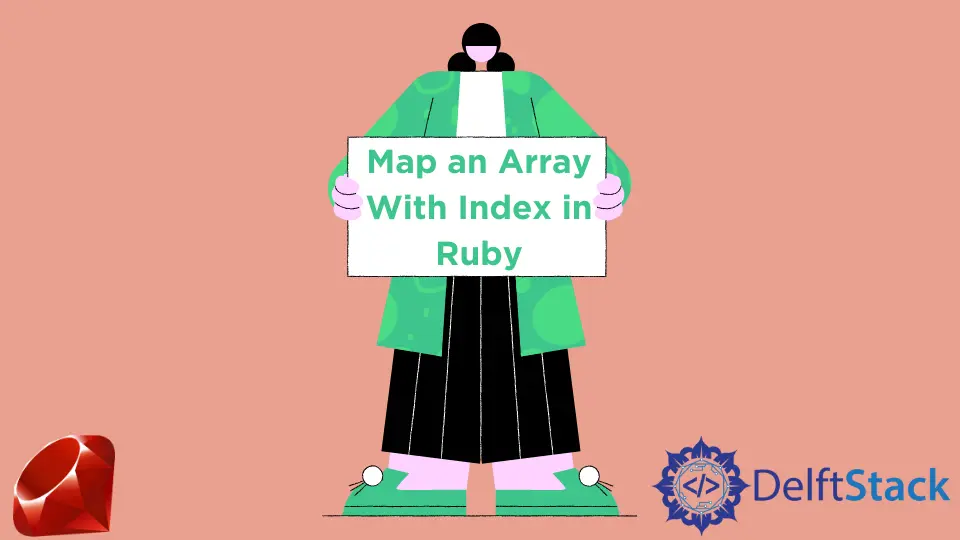
map
方法在 Ruby 中非常方便,當你需要將一個陣列轉換為另一個陣列時,它會派上用場。例如,下面的程式碼使用 map
將小寫字母陣列轉換為大寫字母陣列。
示例程式碼:
small_letters = ['a', 'b', 'c', 'd']
capital_letters = small_letters.map { |l| l.capitalize }
puts capital_letters
輸出:
["A", "B", "C", "D"]
在上面的示例中,假設我們要對映並獲取陣列中每個字母的相應索引。我們如何做到這一點?下面列出了實現這一目標的不同方法。
在 Ruby 中結合 map
和 each_with_index
方法
each_with_index
是一個 Ruby 可列舉的方法。
它執行名稱所指示的操作。它遍歷陣列或雜湊並提取每個元素及其各自的索引。在 each_with_index
的結果上呼叫 map
可以讓我們訪問索引。
這裡是 each_with_index
的官方文件以獲得更多解釋。
示例程式碼:
small_letters = ['a', 'b', 'c', 'd']
numbered_capital_letters = small_letters.each_with_index.map { |l, i| [i + 1, l.capitalize] }
puts numbered_capital_letters
輸出:
[[1, "A"], [2, "B"], [3, "C"], [4, "D"]]
在 Ruby 中結合 map
和 with_index
方法
with_index
類似於 each_with_index
但略有不同。
與 each_with_index
不同,with_index
不能直接在陣列上呼叫。陣列需要首先顯式轉換為 Enumerator。
由於 map
自動處理轉換,執行順序在這裡很重要,在呼叫 .with_index
之前呼叫 .map
。
示例程式碼:
small_letters = ['a', 'b', 'c', 'd']
numbered_capital_letters = small_letters.map.with_index { |l, i| [i + 1, l.capitalize] }
puts numbered_capital_letters
輸出:
[[1, "A"], [2, "B"], [3, "C"], [4, "D"]]
還值得一提的是,with_index
接受一個引數,即索引的偏移量,索引應該從哪裡開始。我們可以使用該引數,而不必在 {}
塊內執行 i + 1
。
示例程式碼:
small_letters = ['a', 'b', 'c', 'd']
numbered_capital_letters = small_letters.map.with_index(1) { |l, i| [i, l.capitalize] }
puts numbered_capital_letters
輸出:
[[1, "A"], [2, "B"], [3, "C"], [4, "D"]]
這裡是 with_index
的官方文件以獲得更多解釋。
將 map
與 Ruby 範圍一起使用
Ruby 範圍是一組具有開始和結束的值。
例如,1..10
或 1...11
是一個 Range,表示從 1
到 10
的一組值。我們可以將它與 map
方法一起使用,以實現與上面示例中相同的結果。
示例程式碼:
small_letters = ['a', 'b', 'c', 'd']
numbered_capital_letters = (0...small_letters.length).map { |i| [i + 1, small_letters[i].capitalize] }
puts numbered_capital_letters
輸出:
[[1, "A"], [2, "B"], [3, "C"], [4, "D"]]
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
相關文章 - Ruby Array
- Ruby 中 each_with_index 和 each.with_index 的區別
- 在 Ruby 中將陣列轉換為字串
- 在 Ruby 中過濾陣列
- 在 Ruby 中對一個陣列求和
- 在 Ruby 中檢查值是否存在於陣列中