How to Convert Set to List in Python
-
Method 1: Using the
list()
Constructor - Method 2: Using List Comprehension
-
Method 3: Using the
*
Operator - Conclusion
- FAQ
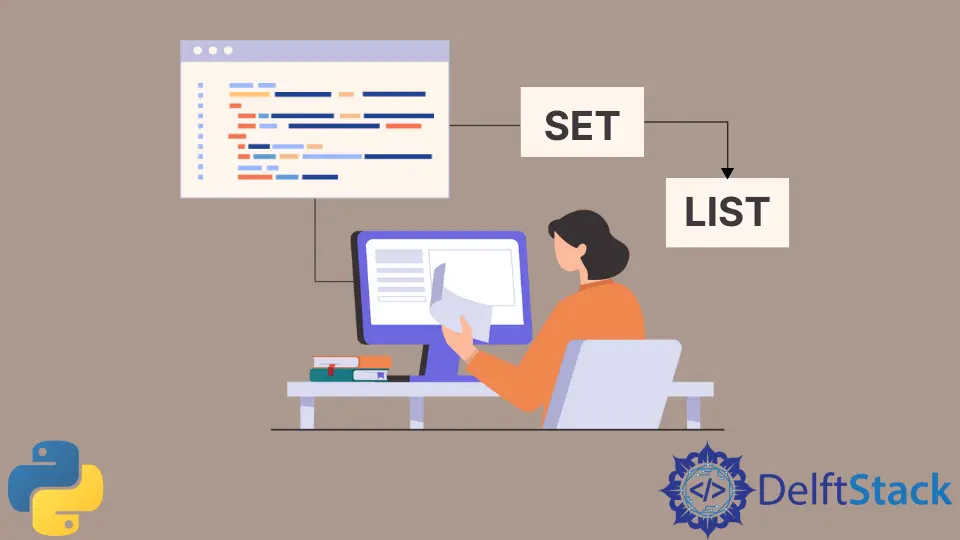
Converting a set to a list in Python is a common task that many developers encounter. Sets are unordered collections of unique elements, while lists are ordered collections that can contain duplicates. Understanding how to switch between these two data types can enhance your data manipulation skills in Python.
In this tutorial, we will explore several methods to convert a set to a list, providing clear examples and explanations for each method. Whether you’re a beginner or an experienced programmer, this guide will help you navigate this essential Python functionality with ease.
Method 1: Using the list()
Constructor
One of the simplest and most straightforward ways to convert a set to a list in Python is by using the built-in list()
constructor. This method is efficient and easy to understand, making it an excellent choice for beginners.
Here’s how you can do it:
my_set = {1, 2, 3, 4, 5}
my_list = list(my_set)
print(my_list)
Output:
[1, 2, 3, 4, 5]
The list()
constructor takes an iterable as an argument and returns a new list containing the elements of that iterable. In this case, we passed our set, my_set
, to the list()
function. The result is a new list, my_list
, that contains all the elements from the set. It’s important to note that the order of elements in the resulting list may vary since sets do not maintain order. However, the unique elements from the set will be preserved in the list format.
Method 2: Using List Comprehension
Another effective way to convert a set to a list is by utilizing list comprehension. This method is not only concise but also allows for additional processing of the elements if needed.
Here’s an example:
my_set = {10, 20, 30, 40, 50}
my_list = [item for item in my_set]
print(my_list)
Output:
[10, 20, 30, 40, 50]
In this example, we created a new list called my_list
by iterating over each element in my_set
using list comprehension. The expression [item for item in my_set]
constructs a new list by taking each item
from the set. Like the list()
constructor, this method also results in a list that contains the unique elements from the set. You can easily modify the comprehension to apply transformations or filters on the elements, making it a versatile option for data manipulation.
Method 3: Using the *
Operator
The unpacking operator *
is another elegant way to convert a set to a list. This method leverages the unpacking feature in Python, which allows you to expand the elements of an iterable into a list.
Here’s how you can implement this method:
my_set = {'apple', 'banana', 'cherry'}
my_list = [*my_set]
print(my_list)
Output:
['banana', 'cherry', 'apple']
In this code snippet, we created a new list, my_list
, by unpacking the elements of my_set
using the *
operator. This method is particularly useful for those who prefer a more concise syntax. However, similar to previous methods, keep in mind that the order of elements in the resulting list may not match the original set due to the unordered nature of sets. This technique is efficient and can be easily integrated into larger data processing tasks.
Conclusion
Converting a set to a list in Python is a fundamental skill that can enhance your programming capabilities. In this tutorial, we explored three effective methods: using the list()
constructor, list comprehension, and the unpacking operator. Each method has its advantages, and the choice often depends on your specific needs and coding style. By mastering these techniques, you can handle data more flexibly and efficiently in your Python projects.
FAQ
-
What is the difference between a set and a list in Python?
A set is an unordered collection of unique elements, while a list is an ordered collection that can contain duplicates. -
Can I convert a list to a set in Python?
Yes, you can convert a list to a set using theset()
constructor. -
Will converting a set to a list maintain the order of elements?
No, sets do not maintain order, so the resulting list may have elements in a different order than they appeared in the set. -
Are there performance differences between the methods used to convert a set to a list?
Generally, all methods have similar performance for small to moderately sized sets, but using thelist()
constructor is often the simplest and most readable. -
Can I convert a set of strings to a list?
Yes, you can convert a set of strings to a list using any of the methods described in this tutorial.
Related Article - Python Set
- How to Join Two Sets in Python
- How to Add Values to a Set in Python
- How to Check if a Set Is a Subset of Another Set in Python
- How to Convert Set to String in Python
- Python Set Pop() Method
- How to Create a Set of Sets in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python