How to Check if a Set Is a Subset of Another Set in Python
-
Use
<=
to Check if a Set Is a Subset of Another Set in Python -
Use the
issubset()
Function to Check if a Set Is a Subset of Another Set in Python -
Use the
all()
Function to Check if a Set Is a Subset of Another Set in Python - Conclusion
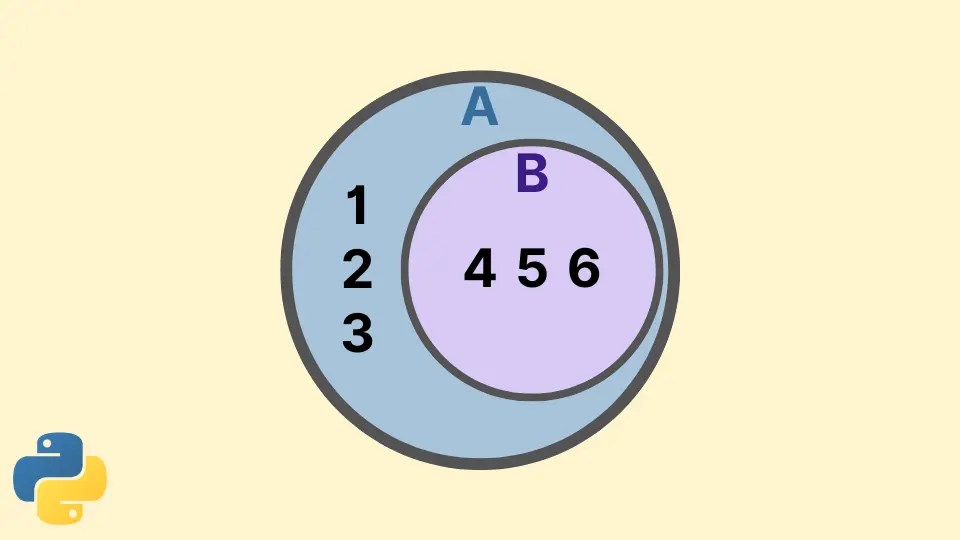
In Mathematics, we have sets representing a collection of mathematical objects like numbers, variables, and more. They can have a different subset, another set containing all the elements of another set (called the superset).
In Python, we have the set
object representing sets and performing different set-related operations. It is an unordered collection of items that contain no duplicate elements.
This tutorial will discuss verifying that one set is a subset of another in Python.
Use <=
to Check if a Set Is a Subset of Another Set in Python
In Python, we can perform different mathematical comparisons for sets. The <=
operator can check if a set is a subset of another set in Python.
Example:
s1 = {1, 3}
s2 = {5, 6, 8, 1, 3, 4}
print(s1 <= s2)
Output:
True
We can use the set()
function to explicitly convert other iterables to a set. This function is helpful when working with other objects like lists, and we want to check whether an object contains the elements of another object.
Remember, the duplicate elements get removed in a set.
See the code below.
s1 = [1, 3]
s2 = [5, 6, 8, 1, 3, 4]
print(set(s1) <= set(s2))
Output:
True
Use the issubset()
Function to Check if a Set Is a Subset of Another Set in Python
The issubset()
function checks if a set is a subset of another set. If another iterable is passed to this function, it will temporarily convert it to a set and return True or False accordingly.
See the code below.
s1 = {1, 3}
s2 = {5, 6, 8, 1, 3, 4}
print(s1.issubset(s2))
Output:
True
Use the all()
Function to Check if a Set Is a Subset of Another Set in Python
The all()
function takes an iterable and returns True if all the elements of this object are True. We can iterate through a list and compare every element to check if it is present in another list using the in
operator.
If all the elements return True, then the all()
function will return True, confirming that list one is a subset of list two; else, it returns False.
We implement this in the code below.
s1 = {1, 3}
s2 = {5, 6, 8, 1, 3, 4}
ch = [i in s2 for i in s1]
print(all(ch))
Output:
True
Conclusion
To conclude, we have discussed how to verify that a set is a subset of another set in Python. The first two methods involved set operations.
The <=
operator and the issubset()
function directly return True or False. The final method involves checking all elements individually and then giving the result.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn