How to Join Two Sets in Python
-
Join Sets in Python Using the Union Operator (
|
) -
Join Sets in Python Using the
update()
Method -
Join Sets in Python Using the
union()
Method -
Join Sets in Python Using
reduce()
andoperator.or_
-
Join Sets in Python Using
itertools.chain()
-
Join Sets in Python Using Unpacking Operator (
*
) - Conclusion
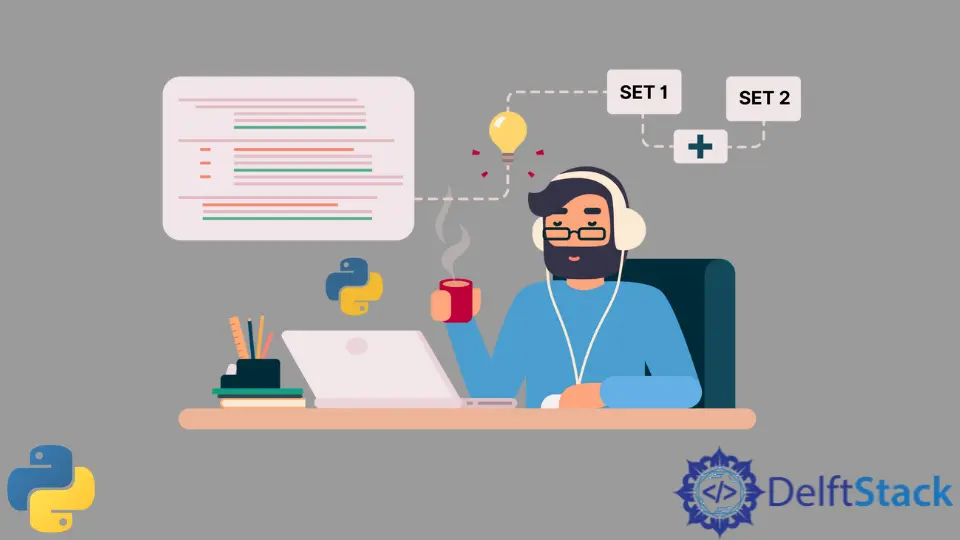
In Python, sets provide a versatile and efficient way to handle collections of unique elements. When it comes to joining two sets, there are several methods and operators at our disposal.
Let’s explore various techniques for combining sets, discussing their syntax and functionality, and providing practical examples.
Join Sets in Python Using the Union Operator (|
)
When you need to combine two sets, the union operator (|
) comes in handy. This is a binary operator that allows you to merge the elements of two sets into a single set, excluding duplicates.
The result is a new set containing all unique elements from both input sets. This operation does not modify the original sets; instead, it creates a new set with the combined elements.
Consider the following example, where we have two sets, set1
and set2
, and we want to combine them using the union operator:
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result_set = set1 | set2
print(result_set)
Here, we first define two sets, set1
and set2
, each containing unique elements. The union operator (|
) is then applied to these sets, creating a new set, result_set
, that contains all unique elements from both set1
and set2
.
The union operation is straightforward: it combines the elements of both sets, excluding duplicates. The resulting set, result_set
, reflects the union of set1
and set2
.
Output:
{1, 2, 3, 4, 5, 6}
As seen in the output, the union operator successfully combines the elements of set1
and set2
, creating a new set with all unique elements from both sets.
Join Sets in Python Using the update()
Method
In Python, the update()
method is a powerful tool when it comes to combining two sets. The update()
method is a set method in Python that adds elements from another set (or any iterable) to the current set.
It modifies the set in place, meaning it directly affects the set on which it is called rather than creating a new set.
Here is the syntax of the update()
method:
set.update([iterable])
Where:
set
: The set on which theupdate()
method is called. It will be modified in place.iterable
: An iterable (e.g., another set, list, tuple, or string) whose elements will be added to the set.
Consider the following example, where we have two sets, set1
and set2
, and we want to join them using the update()
method:
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
set1.update(set2)
print(set1)
In this code snippet, we start by defining two sets, set1
and set2
, each containing unique elements. The update()
method is then applied to set1
, and we pass set2
as an argument.
This method adds all elements from set2
to set1
, effectively joining the two sets. Importantly, this operation is performed in place, meaning set1
is modified directly.
Output:
{1, 2, 3, 4, 5, 6}
As we can see in the output, the update()
method successfully combines the elements of set1
and set2
. The resulting set, set1
, now contains all unique elements from both sets.
Join Sets in Python Using the union()
Method
In Python, the union()
method offers an alternative way to combine two sets. The union()
method takes another set as an argument, computes the union of the two sets, and produces a new set containing all unique elements from both sets.
Importantly, this operation does not modify the sets on which it is called; instead, it creates and returns a new set.
Here is the syntax of the union()
method:
set.union(*others)
Where:
set
: The set on which theunion()
method is called.others
: One or more sets or iterables whose elements will be included in the union.
Consider the following example, where we have two sets, set1
and set2
, and we want to join them using the union()
method:
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result_set = set1.union(set2)
print(result_set)
Here, we begin by defining two sets, set1
and set2
, each containing unique elements. The union()
method is then applied to set1
, with set2
passed as an argument.
This method computes the union of the two sets and returns a new set, which we assign to the variable result_set
. The original sets, set1
and set2
, remain unchanged.
Output:
{1, 2, 3, 4, 5, 6}
As evident from the output, the union()
method successfully combines the elements of set1
and set2
into a new set with all unique elements. This method provides an efficient way to join sets while preserving the original sets.
Join Sets in Python Using reduce()
and operator.or_
The functools
module provides the reduce()
function, which, when combined with the operator.or_
function, offers an alternative method for joining two sets. This approach is particularly useful for scenarios where you want to perform set union iteratively across multiple sets.
The reduce()
function from the functools
module is a versatile tool that applies a specified function (in this case, operator.or_
) cumulatively to the items of an iterable (in this case, a list of sets).
The operator.or_
function performs a bitwise or
operation on two sets, effectively yielding their union. By combining these two, we can iteratively join sets.
Here is the syntax of the reduce()
function:
functools.reduce(function, iterable[, initializer])
Where:
function
: A function that takes two arguments and performs the operation to cumulatively apply to the items of the iterable.iterable
: An iterable (e.g., a list, tuple, or string) whose items will be processed by the function cumulatively.initializer
(optional): If provided, it is placed before the items of the iterable in the calculation and serves as a default when the iterable is empty.
Consider the following example, where we use reduce()
along with operator.or_
to join two sets:
import operator
from functools import reduce
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result_set = reduce(operator.or_, [set1, set2])
print(result_set)
In this code, we start by importing the necessary modules, operator
and functools
. We then define two sets, set1
and set2
, each containing unique elements.
The reduce()
function is applied to the list [set1, set2]
using the operator.or_
function as the combining operation. This iteratively performs set union, resulting in a new set assigned to result_set
.
Output:
{1, 2, 3, 4, 5, 6}
As evident from the output, the combination of reduce()
and operator.or_
successfully joins the elements of set1
and set2
, creating a new set with all unique elements.
It’s crucial to note that the reduce
function behaves differently in Python 2.x and Python 3. In Python 2.x, reduce
is a built-in function, but in Python 3, it has been moved to the functools
module and is accessible as functools.reduce
.
To ensure compatibility across both Python 2 and 3, we must use functools.reduce
in our code.
Join Sets in Python Using itertools.chain()
In Python, the itertools.chain()
method provides a versatile and efficient way to join multiple sets or iterables. Unlike some of the set-specific methods, itertools.chain()
can be used to concatenate elements from different iterable types.
The itertools.chain()
method is part of the itertools
module in Python. It takes multiple iterables as arguments and returns an iterator that produces items from the first iterable until it is exhausted, then it continues to the next iterable.
This makes it suitable for combining sets, lists, or any other iterable types.
Here is the syntax of the itertools.chain()
method:
itertools.chain(*iterables)
Where:
iterables
: One or more iterables (e.g., sets, lists, tuples, or strings) whose elements will be combined in the iterator.
Consider the following example, where we use itertools.chain()
to join two sets:
import itertools
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result_set = set(itertools.chain(set1, set2))
print(result_set)
Here, we begin by importing the itertools
module. We then define two sets, set1
and set2
, each containing unique elements.
The itertools.chain()
method is applied to these sets, creating an iterator that produces items from set1
until it is exhausted and then continues with set2
. To obtain a set from the iterator, we wrap it with set()
, resulting in the variable result_set
.
Importantly, this operation does not modify the original sets; it creates a new set with the combined elements.
Output:
{1, 2, 3, 4, 5, 6}
This method provides a flexible and general approach for joining different iterable types, making it a valuable tool in scenarios where you need to concatenate elements from various sources.
Join Sets in Python Using Unpacking Operator (*
)
In Python, the unpacking operator (*
) is a straightforward tool for combining elements from multiple iterables, including sets.
The unpacking operator (*
) is a versatile feature in Python that allows us to unpack the elements of an iterable, such as a set, list, or tuple. When used with sets, it concatenates the elements of the sets and creates a new set with all unique items.
Consider the following example, where we use the unpacking operator to join two sets:
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
result_set = {*set1, *set2}
print(result_set)
Here, we start by defining two sets, set1
and set2
, each containing unique elements. The *set1
and *set2
syntax unpacks the elements of each set, and the resulting iterable is used to create a new set (result_set
).
Importantly, this operation does not modify the original sets; instead, it creates a new set with the combined elements.
Output:
{1, 2, 3, 4, 5, 6}
As evident from the output, the unpacking operator successfully combines the elements of set1
and set2
into a new set with all unique elements.
Conclusion
Choosing the appropriate method for joining sets depends on your specific use case and preferences. Whether you prioritize readability, conciseness, or compatibility, Python offers a range of options to suit your needs.
Experiment with these techniques and discover the one that best fits your requirements for joining sets in Python.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook