How to Convert Set to String in Python
-
Method 1: Using the
join()
Method - Method 2: Using List Comprehension
-
Method 3: Using the
str()
Function - Conclusion
- FAQ
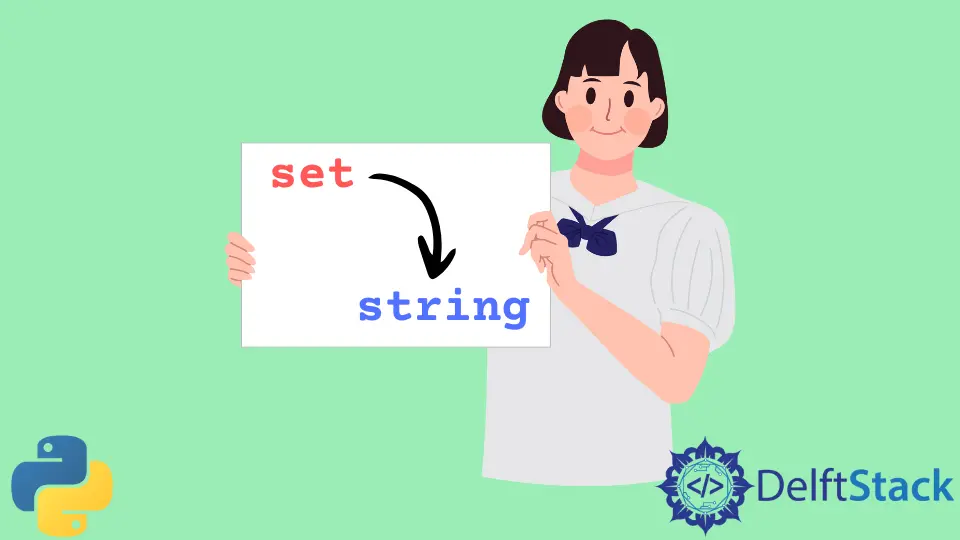
Converting a set to a string in Python is a common task that can help you present data in a more readable format. Whether you’re preparing data for display or simply need a string representation for logging or debugging, knowing how to perform this conversion is essential.
In this tutorial, we will explore several methods to convert a set to a string in Python, complete with clear code examples and detailed explanations. By the end of this guide, you will have a solid understanding of how to manipulate sets and strings effectively in Python, enhancing your programming skills and making your code more efficient.
Method 1: Using the join()
Method
One of the most straightforward ways to convert a set to a string in Python is by using the join()
method. This method is particularly useful when you want to create a string from the elements of a set, with a specific separator between them.
Here’s how you can do it:
my_set = {"apple", "banana", "cherry"}
result = ", ".join(my_set)
print(result)
Output:
banana, cherry, apple
In this example, we create a set called my_set
with three fruit names. We then use the join()
method, specifying ", "
as the separator. It’s important to note that sets are unordered collections, so the output may vary each time you run the code. The join()
method effectively concatenates the elements of the set into a single string, separated by the specified delimiter.
This method is not only efficient but also flexible, as you can easily change the separator to suit your needs. For instance, if you want to separate the items with a hyphen or a newline character, you can simply modify the string passed to join()
. This makes it a versatile option for converting sets to strings in Python.
Method 2: Using List Comprehension
Another effective way to convert a set to a string is by utilizing list comprehension. This method allows you to create a list from the set elements and then convert that list to a string using join()
.
Here’s how you can implement this approach:
my_set = {"apple", "banana", "cherry"}
result = ", ".join([str(item) for item in my_set])
print(result)
Output:
banana, cherry, apple
In this code snippet, we use a list comprehension to iterate through each item in my_set
, converting each item to a string (though they are already strings in this case). The resulting list is then passed to the join()
method, which concatenates the elements into a single string, separated by a comma and a space.
The beauty of this method lies in its flexibility. If your set contains non-string elements, the str(item)
conversion ensures that they are properly transformed into strings before concatenation. This makes it a robust option for converting a diverse range of sets into string format.
Method 3: Using the str()
Function
If you’re looking for a quick and straightforward way to convert a set to a string, you can use the built-in str()
function. This function will return a string representation of the set, including the curly braces that denote it as a set.
Here’s a simple example:
my_set = {"apple", "banana", "cherry"}
result = str(my_set)
print(result)
Output:
{'banana', 'cherry', 'apple'}
In this example, we directly pass my_set
to the str()
function, which returns a string representation of the set. While this method is easy to implement, it may not always produce the desired format. The output includes the curly braces and the elements are not separated by a specific character.
This approach is best suited for debugging or logging purposes, where you need a quick view of the set’s contents without worrying about formatting. However, if you need a cleaner string representation, consider using one of the previous methods.
Conclusion
Converting a set to a string in Python can be accomplished in several ways, each with its own advantages. The join()
method offers flexibility and customization, while list comprehension provides a robust solution for diverse data types. The str()
function is perfect for quick, informal outputs. Understanding these methods enhances your ability to manipulate data in Python effectively. Whether you are preparing data for display or debugging your code, knowing how to convert sets to strings is a valuable skill that will serve you well in your programming journey.
FAQ
-
How do I convert a set to a string without separators?
You can use thestr()
function to get a string representation of the set, which includes the curly braces. -
Can I customize the separator when converting a set to a string?
Yes, you can use thejoin()
method to specify any separator you like when converting a set to a string.
-
What happens if my set contains non-string elements?
You can use list comprehension withstr(item)
to convert non-string elements to strings before concatenation. -
Is the order of elements preserved when converting a set to a string?
No, sets are unordered collections, so the order of elements may vary each time you convert them to a string. -
Which method is the most efficient for converting large sets to strings?
Thejoin()
method is generally the most efficient for larger sets, especially when combined with list comprehension.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python