How to Create a Set of Sets in Python
- Understanding Sets in Python
- Method 1: Using Nested Sets
- Method 2: Using Frozensets
- Method 3: Combining Sets with List Comprehensions
- Conclusion
- FAQ
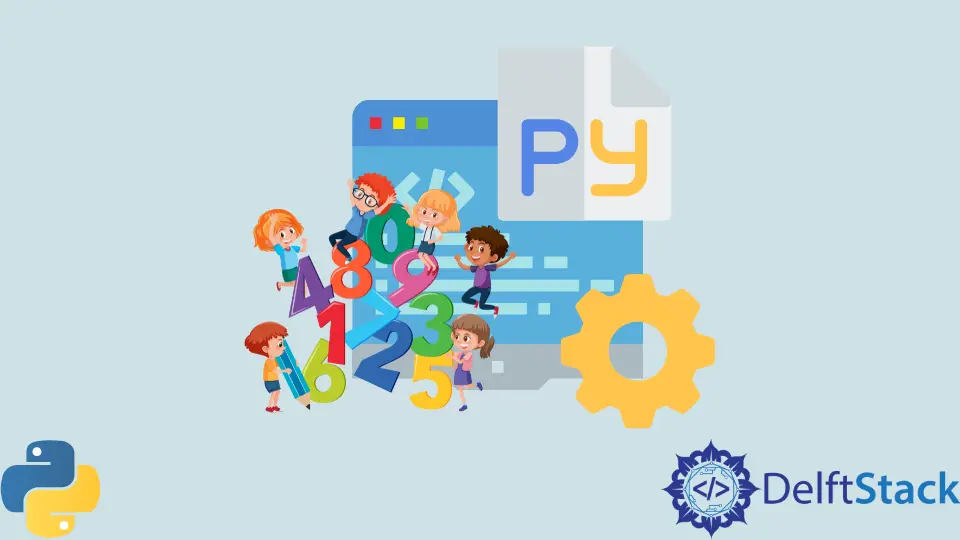
Creating a set of sets in Python is a unique and powerful concept that allows you to manage collections of unique elements effectively. Sets, as we know, are unordered collections that do not allow duplicate values. When you create a set of sets, you can harness the power of sets while maintaining the uniqueness of each subset.
This article will guide you through the various methods to create a set of sets in Python, complete with clear code examples and explanations. Whether you are a beginner or an experienced programmer, this comprehensive guide will enhance your understanding of Python’s set data structure and its versatility.
Understanding Sets in Python
Before we dive into creating a set of sets, it’s essential to grasp what sets are in Python. A set is a built-in data type that allows you to store multiple items in a single variable. The elements in a set are unordered, meaning that their positions do not matter, and they must be unique, which means no duplicate values are allowed.
To create a basic set, you can use curly braces or the set()
function. Here’s a simple example:
my_set = {1, 2, 3}
Output:
{1, 2, 3}
In this example, my_set
contains three unique elements. Now that we have a basic understanding of sets, let’s explore how to create a set of sets.
Method 1: Using Nested Sets
One of the simplest ways to create a set of sets in Python is by nesting sets within a parent set. This method allows you to group multiple sets together, maintaining their unique properties. Here’s how you can do it:
set1 = {1, 2}
set2 = {3, 4}
set3 = {5, 6}
set_of_sets = {set1, set2, set3}
Output:
{{1, 2}, {3, 4}, {5, 6}}
In this example, we first create three individual sets: set1
, set2
, and set3
. Then, we combine these sets into a parent set called set_of_sets
. Each subset retains its uniqueness, and you can access them individually if needed.
This method is straightforward and effective, especially when you need to manage different collections of elements that do not overlap. However, it’s crucial to remember that the sets themselves must also be immutable. This means you cannot add or remove elements from the sets that are part of the parent set after its creation.
Method 2: Using Frozensets
While sets are mutable, meaning you can change their contents, frozensets are immutable and can be used as elements of another set. This property makes frozensets ideal for creating a set of sets. Let’s take a look at how to implement this:
frozenset1 = frozenset([1, 2])
frozenset2 = frozenset([3, 4])
frozenset3 = frozenset([5, 6])
set_of_frozensets = {frozenset1, frozenset2, frozenset3}
Output:
{frozenset({1, 2}), frozenset({3, 4}), frozenset({5, 6})}
In this example, we create three frozensets: frozenset1
, frozenset2
, and frozenset3
. These frozensets are then combined into a parent set called set_of_frozensets
. The advantage of using frozensets is that they can be modified in terms of their parent set, allowing you to manage collections without worrying about the immutability of their contents.
Using frozensets is particularly useful when you need to ensure that the subsets remain unchanged, making this approach suitable for scenarios where data integrity is critical.
Method 3: Combining Sets with List Comprehensions
Another effective way to create a set of sets is by using list comprehensions. This method allows you to generate sets dynamically and combine them into a parent set. Here’s how you can achieve this:
sets = [{1, 2}, {3, 4}, {5, 6}]
set_of_sets = {frozenset(s) for s in sets}
Output:
{frozenset({1, 2}), frozenset({3, 4}), frozenset({5, 6})}
In this example, we start with a list of sets and use a set comprehension to convert each set into a frozenset before adding it to set_of_sets
. This approach is particularly powerful because it allows for easy manipulation and generation of sets based on conditions or existing data.
Using list comprehensions not only makes your code cleaner but also enhances readability. This method is highly recommended if you are dealing with dynamic data or need to create sets based on specific criteria.
Conclusion
Creating a set of sets in Python can be accomplished through various methods, each with its advantages. Whether you choose to use nested sets, frozensets, or list comprehensions, understanding these techniques will empower you to manage collections of unique elements effectively. As you explore the power of sets in Python, you’ll find that they offer a flexible and efficient way to handle data. So go ahead and experiment with these methods to see how they can enhance your programming skills!
FAQ
-
What is a set in Python?
A set in Python is an unordered collection of unique elements, allowing you to store multiple items in a single variable without duplicates. -
Can I add elements to a frozenset?
No, frozensets are immutable, meaning you cannot add or remove elements once they are created. -
What is the difference between a set and a frozenset?
The primary difference is that sets are mutable, allowing changes, while frozensets are immutable and cannot be modified after creation. -
How can I check if a set contains a specific element?
You can use thein
keyword to check for membership, likeelement in my_set
. -
Can sets contain other sets?
No, sets cannot contain other sets directly, but they can contain frozensets since frozensets are immutable.