Python Set Pop() Method
- Understanding the Set Data Structure
- The pop() Method: How It Works
- Handling Empty Sets with pop()
- Practical Applications of the pop() Method
- Conclusion
- FAQ
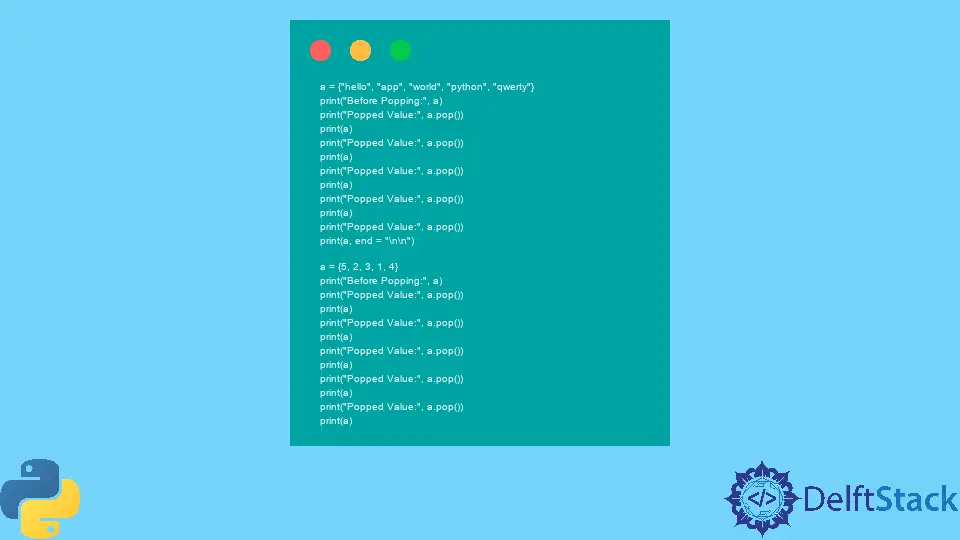
In the world of Python programming, sets are a powerful data structure that allows for the storage of unique elements. One of the most useful methods associated with sets is the pop()
method. This method not only provides a way to remove an arbitrary element from a set but also returns that element, enabling dynamic data manipulation. Whether you’re building a simple application or diving into more complex data structures, understanding how to effectively use the pop()
method can enhance your programming skills.
In this article, we will explore the pop()
method of a set in Python, providing clear examples and explanations to ensure you grasp its functionality.
Understanding the Set Data Structure
Before diving into the pop()
method, it’s essential to understand what a set is in Python. A set is an unordered collection of unique items. This means that no two elements in a set can be the same, and the order of elements is not guaranteed. Sets are particularly useful for membership testing and eliminating duplicate entries.
To create a set in Python, you can use curly braces or the set()
function. Here’s a quick example of how to create a set:
my_set = {1, 2, 3, 4, 5}
Output:
{1, 2, 3, 4, 5}
In this example, my_set
contains five unique integers. Now that we have a basic understanding of sets, let’s explore the pop()
method in detail.
The pop() Method: How It Works
The pop()
method is a built-in function that removes and returns an arbitrary element from a set. It’s important to note that since sets are unordered, the element removed by pop()
is not predictable. If the set is empty, calling pop()
will raise a KeyError
.
Here’s how you can use the pop()
method in Python:
my_set = {1, 2, 3, 4, 5}
removed_element = my_set.pop()
print("Removed Element:", removed_element)
print("Updated Set:", my_set)
Output:
Removed Element: 1
Updated Set: {2, 3, 4, 5}
In this code snippet, we first create a set containing five integers. We then call the pop()
method, which removes an arbitrary element from the set and stores it in the variable removed_element
. The updated set is printed afterward. The output demonstrates that one element has been removed, and the set now contains four items.
The pop()
method is particularly handy when you need to process items in a set without caring about their order. It can be useful in algorithms that require dynamic data manipulation, such as when implementing certain data structures or algorithms.
Handling Empty Sets with pop()
One of the critical aspects to remember when using the pop()
method is how it behaves with empty sets. If you attempt to call pop()
on an empty set, Python will raise a KeyError
. To avoid this, you should always check if the set is empty before calling pop()
. Here’s how to do it:
my_set = set()
if my_set:
removed_element = my_set.pop()
print("Removed Element:", removed_element)
else:
print("The set is empty; nothing to pop.")
Output:
The set is empty; nothing to pop.
In this example, we first check if my_set
contains any elements. If it does, we proceed to call pop()
. If the set is empty, we print a message indicating that there is nothing to pop. This simple check can prevent runtime errors and make your code more robust.
Using the pop()
method effectively requires understanding how to handle different scenarios, including empty sets. This practice not only enhances your coding skills but also ensures that your programs run smoothly without unexpected errors.
Practical Applications of the pop() Method
The pop()
method can be particularly useful in various scenarios, especially when working with algorithms that require dynamic data manipulation. For instance, if you’re implementing a stack using a set, the pop()
method can help you retrieve and remove elements efficiently.
Consider a situation where you want to process a set of tasks. You could use the pop()
method to remove tasks one by one as they are completed. Here’s a simple example:
tasks = {"task1", "task2", "task3"}
while tasks:
current_task = tasks.pop()
print("Processing:", current_task)
Output:
Processing: task1
Processing: task2
Processing: task3
In this code, we have a set of tasks. The while
loop continues until the set is empty. Each iteration calls pop()
, removing and processing a task. This approach is straightforward and effective for handling tasks without worrying about their order.
Using pop()
in this way demonstrates its versatility. Whether you’re managing tasks, implementing algorithms, or simply manipulating data, the pop()
method can be an invaluable tool in your Python toolkit.
Conclusion
The pop()
method of a set in Python is a powerful tool for removing and returning arbitrary elements from a set. Understanding its functionality, especially in relation to empty sets and practical applications, can greatly enhance your programming skills. By mastering this method, you can efficiently manipulate data structures and write more dynamic and robust Python code. As you continue your journey in Python programming, keep the pop()
method in mind as a key feature of sets that can simplify your data handling tasks.
FAQ
-
What does the pop() method do in Python sets?
The pop() method removes and returns an arbitrary element from a set. -
Can I predict which element will be removed by pop()?
No, since sets are unordered, the element removed by pop() is not predictable. -
What happens if I call pop() on an empty set?
A KeyError will be raised if you try to pop from an empty set. -
How can I avoid errors when using pop() on a set?
Always check if the set is empty before calling pop() to avoid runtime errors. -
Can I use pop() in a loop?
Yes, you can use pop() in a loop to process elements until the set is empty.