How to Add Values to a Set in Python
-
Add Only a Single Value to a Set With the
Set.add()
Function in Python -
Add Multiple Values to a Set With the
Set.update()
Function in Python
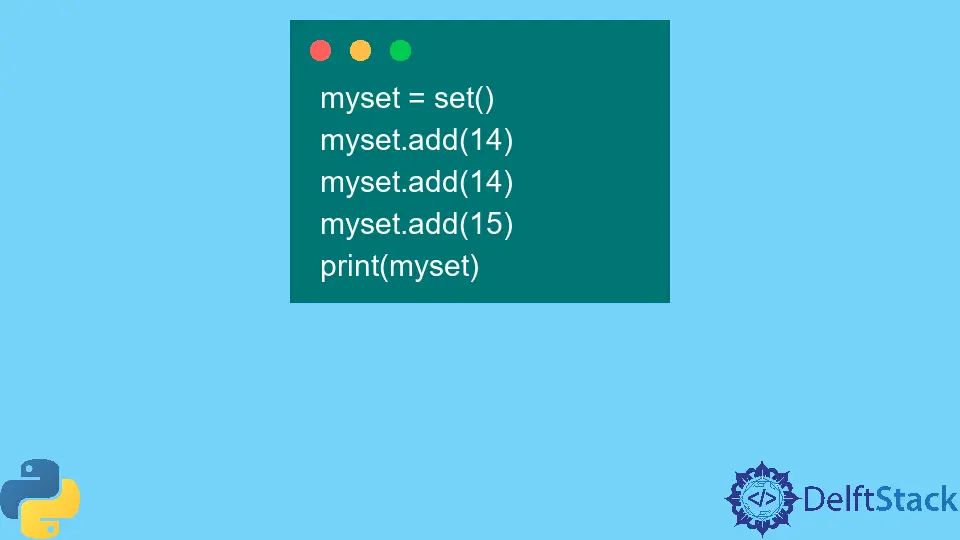
This tutorial will discuss the methods we can use to append values to a set in Python.
Add Only a Single Value to a Set With the Set.add()
Function in Python
Mathematically, a set is a collection of distinct objects that are logically related. In Python, the set is a built-in data type that is unindexed and immutable.
This means that we can access set items with some specific index, and we cannot modify existing data inside a set.
We can declare a set by creating a set
class object in Python. We can use the built-in add()
method to append values into our newly created set.
The following code snippet demonstrates how we can append values to a set with the add()
method in Python.
myset = set()
myset.add(14)
myset.add(14)
myset.add(15)
print(myset)
Output:
{14, 15}
The above output demonstrates another property of sets that was not mentioned earlier. A set only contains distinct values, so we can’t have duplicate values inside a set.
The only drawback of the add()
method is that it can only add a single value to our set.
Add Multiple Values to a Set With the Set.update()
Function in Python
The add()
method works fine, but it only takes one input, making our task very tedious if we want to add thousands of values inside a single set. In this type of situation, we can utilize the built-in update()
method that can add multiple values to our set at a single time.
The update()
method takes an iterable object as input, iterates over it, and adds each item into our set. Our iterable doesn’t need to be a list at all times.
The following code snippet shows us how to add multiple values to our set with the update()
method in Python.
myset.update([1110, 3, 4])
print(myset)
Output:
{1, 3, 4, 14, 15, 1110}
We added new values to our set from the previous example with the update()
method in Python.
Although it is the clear winner in saving our time, the update()
method has flaws. For example, if we want to add a string to our set, the update()
function will iterate through the whole string and add each unique character to our set, as shown in the code example below.
myset.update("this is my value")
print(myset)
Output:
{1, 3, 4, 's', 'm', 'a', 'h', 14, 15, 'l', 'y', 'u', 'e', 1110, 'v', 't', ' ', 'i'}
This is because the string is an iterable object in Python. We must enclose our string inside another iterable (like a list or a set) to solve this problem.
This step is shown in the following code example.
myset.update(["this is my value"])
print(myset)
Output:
{1, 3, 4, 'm', 14, 15, 'i', 's', 'l', 'y', ' ', 'u', 'h', 1110, 'v', 'this is my value', 't', 'a', 'e'}
This time, the whole string gets added inside our set instead of each character.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn