Python Tutorial - Data Type-Set
- Create Python Sets
- Python Set Update
- Remove Elements From a Python Set
- Python Set Operations
- Python Set Methods
- Other Python Set Operations
- Python Set Built-In Functions
- Python Frozenset
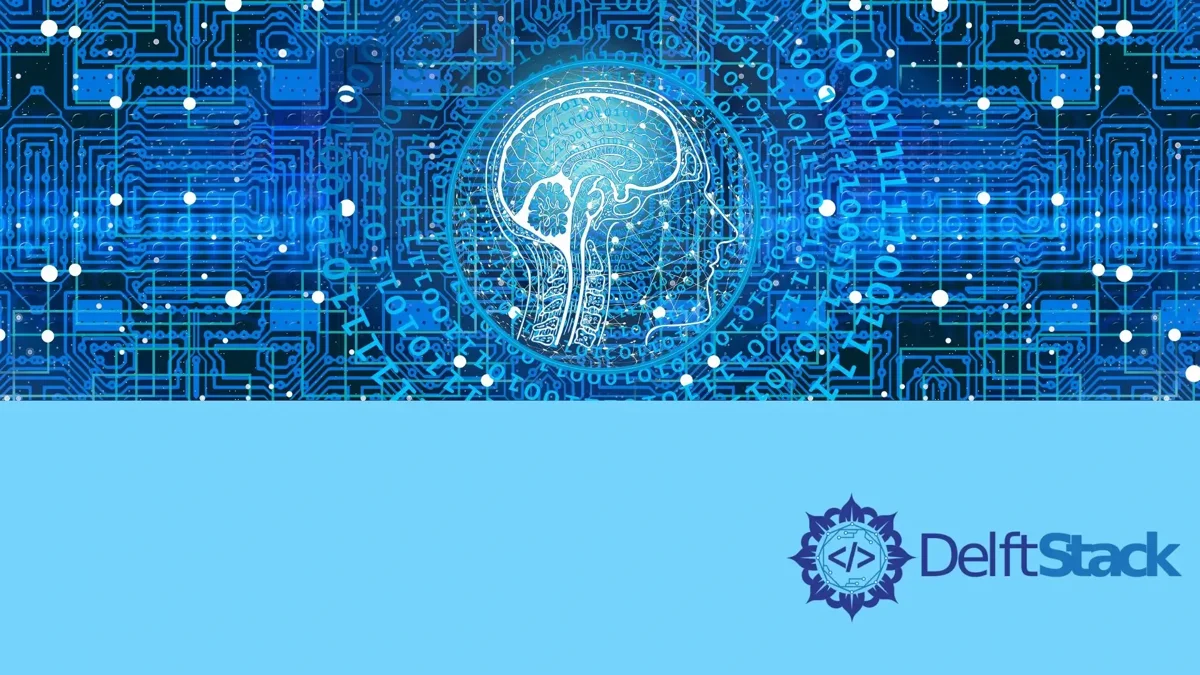
In this section, you will learn how to create sets in Python and how to add and remove elements from sets.
Set contains unique items and is an unordered list of elements.
set
is a mutable object so that you can add and delete items from a set.Create Python Sets
A set in Python can be created using curly braces {}
and the items are separated by commas. You can also create a set using the built-in method set()
.
The elements of a set can be of different data types. The elements of a set should be immutable. For example, you can have a tuple as an element of the set but you cannot have a set or dictionary or list as elements of the set.
>>> x = {3, 5, 7, 2, 4, 5}
>>> print(x) #prints the set variable
{2, 3, 4, 5, 7}
>>> print(type(x)) #checking type of x
<class 'set'>
You could use the set()
method with empty parameters to create an empty list. If you write {}
to create an empty object, it is interpreted as a dictionary:
>>> x = {}
>>> print(type(x))
<class 'dict'>
>>> x = set()
>>> print(type(x))
<class 'set'>
Python Set Update
Indexing and slicing cannot be used to change elements of a set because set
is not a ordered data type.
A single element can be added in a set using the add()
method. If you want to add more than one element in the set then you have to use the update()
method.
>>> s = {2, 4}
>>> print(s)
{2, 4}
>>> s.add(6)
>>> print(s)
{2, 4, 6}
>>> s.update([2, 4, 6])
>>> print(s)
{2, 4, 6}
>>> s.update([5,6], {7, 8, 9})
>>> print(s)
{2, 4, 5, 6, 7, 8, 9}
Remove Elements From a Python Set
To remove an element from a set you can either use discard()
method or remove()
method.
The difference between these methods is that discard()
method will not prompt anything if the element to be deleted is not in the set whereas, remove()
will generate an error if the element to be deleted is not found.
>>> s = {2, 4, 5, 6, 7, 8, 9}
>>> print(s)
{2, 4, 5, 6, 7, 8, 9}
>>> s.discard(6)
>>> print(s)
{2, 4, 5, 7, 8, 9}
>>> s.remove(8)
>>> print(s)
{2, 4, 5, 7, 9}
>>> s.discard(6) #no error
>>> s.remove(8) #generated an error
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
s.remove(8)
KeyError: 8
You can also use pop()
to remove an item from a set. pop()
removes and returns one arbitrary set element.
>>> s = set("Python")
>>> s
{'o', 'n', 'h', 'P', 'y', 't'}
>>> s.pop()
o
>>> s.pop()
n
>>> s
{'h', 'P', 'y', 't'}
>>> s.clear()
>>> print(s)
set()
You could use set clear()
method to clear all the set elements.
>>> s = set("Python")
>>> s.clear()
>>> s
set()
Python Set Operations
You can perform operations such as union
, intersection
, difference
, and symmetric_difference
.
Suppose you have two sets x
and y
:
>>> x = {1, 2, 3, 4, 5, 6}
>>> y = {7, 8, 9, 10, 11, 12}
Union
The Union of two sets results in a new set containing all elements of both the sets.
To perform union operation, you can use either |
operator or the union()
method.
>>> print(x | y)
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12}
#using union() method on x
>>> x.union(y)
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12}
#union on y
>>> y.union(x)
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12}
Python Set Intersection
The Intersection of two sets results in a new set containing the items that are common in both of the sets.
To perform intersection operation, you can use either &
operator or the intersection()
method.
>>> x = {1, 2, 3, 4, 5, 6}
>>> y = {7, 8, 9, 2, 6, 1}
>>> print(x & y)
{1, 2, 6}
#using intersection() method on x
>>> x.intersection(y)
{1, 2, 6}
#intersection on y
>>> y.intersection(x)
{1, 2, 6}
Python Sets Difference
The difference of two sets results in a new set that contains elements of the first set that are not present in the second set.
For example, x - y
is elements of x
not in y
. Similarly, y - x
is elements of y
not in x
.
To perform difference, you can use either -
operator or the difference()
method.
>>> x = {1, 2, 3, 4, 5, 6}
>>> y = {7, 8, 9, 2, 6, 1}
>>> print(x - y)
{3, 4, 5}
#using difference() method on x
>>> x.difference(y)
{3, 4, 5}
#diference on y
>>> y.difference(x)
{8, 9, 7}
Python Sets Symmetric Difference
The symmetric difference of two sets results in a new set that contains elements that are not common in both sets.
To perform symmetric difference, you can use either ^
operator or the symmetric_difference()
method.
>>> x = {1, 2, 3, 4, 5, 6}
>>> y = {7, 8, 9, 2, 6, 1}
>>> print(x ^ y)
{3, 4, 5, 7, 8, 9}
#using symmetric_difference() method on x
>>> x.symmetric_difference(y)
{3, 4, 5, 7, 8, 9}
#symmetric_diference on y
>>> y.symmetric_difference(x)
{3, 4, 5, 7, 8, 9}
Python Set Methods
The following is the list of methods that can be applied on sets in Python:
Method | Description |
---|---|
add() |
add an element to a set |
clear() |
clear the elements of the set (clears entire set) |
copy() |
return the copy of a set |
difference() |
return a new set that contains elements of the first set that are not present in the second set. |
difference_update() |
remove all elements of another set from the current set |
discard() |
remove a specific element from a set |
intersection() |
return a new set containing the items that are common in both of the sets. |
intersection_update() |
update a set with the intersection of this set with another set |
isdisjoint() |
return a True when two sets results in a null intersection |
issubset() |
return a True when another set has the current set |
issuperset() |
return a True when the current set has another set |
pop() |
return an element from a set |
remove() |
remove a specific element from a set. If the element is not in the set an error will be generated. |
symmetric_difference() |
return a new set that contains elements that are not common in both sets. |
symmetric_difference_update() |
update a set with the symmetric difference of this set with another set |
union() |
return a new set containing all elements of both the sets. |
update() |
update a set with the union of this set with another set |
Other Python Set Operations
Set Membership Check
The in
keyword tests if an item is a member of the set or not.
>>> s = set("Blue")
>>> print('o' in s)
False
>>> print('l' in s)
True
Iterate Through a Set
You can iterate through a set by using for
loop:
for i in set("Blue"):
print(i)
Result:
B
l
u
e
Python Set Built-In Functions
The following are some of the built-in functions that can be used with sets to perform different tasks:
Functions | Description |
---|---|
all() |
return True when all the elements of the set are True . It also returns True when the set is empty. |
any() |
return True when any of the element of the set is True . It returns False when the set is empty. |
enumerate() |
return the index and the value of all the elements of the set as a tuple. It returns an enumerate object. |
len() |
return the number of items in a set or the length of the set. |
set() |
define a set. |
max() |
return the maximum item in the set. |
min() |
return the minimum item in the set. |
sorted() |
return a sorted list of elements of a set. |
sum() |
return the sum of all elements of the set. |
Python Frozenset
Frozenset is a special set whose elements cannot be updated when assigned once. Frozensets are immutable sets.
As sets are mutable so you cannot use them as a key for in dictionary but frozen sets are immutable, hence, you can use frozen sets as dictionary keys.
A frozenset can be created using the frozenset()
method.
The following are some of the methods supported by frozenset:
copy()
difference()
intersection()
isdisjoint()
issubset()
issuperset()
symmetric_difference()
union()
>>> x = frozenset([2,6,3,9])
>>> y = frozenset([6,1,2,4])
>>> x.difference(y)
frozenset({9, 3})
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook