Python 資料型別 - 集合
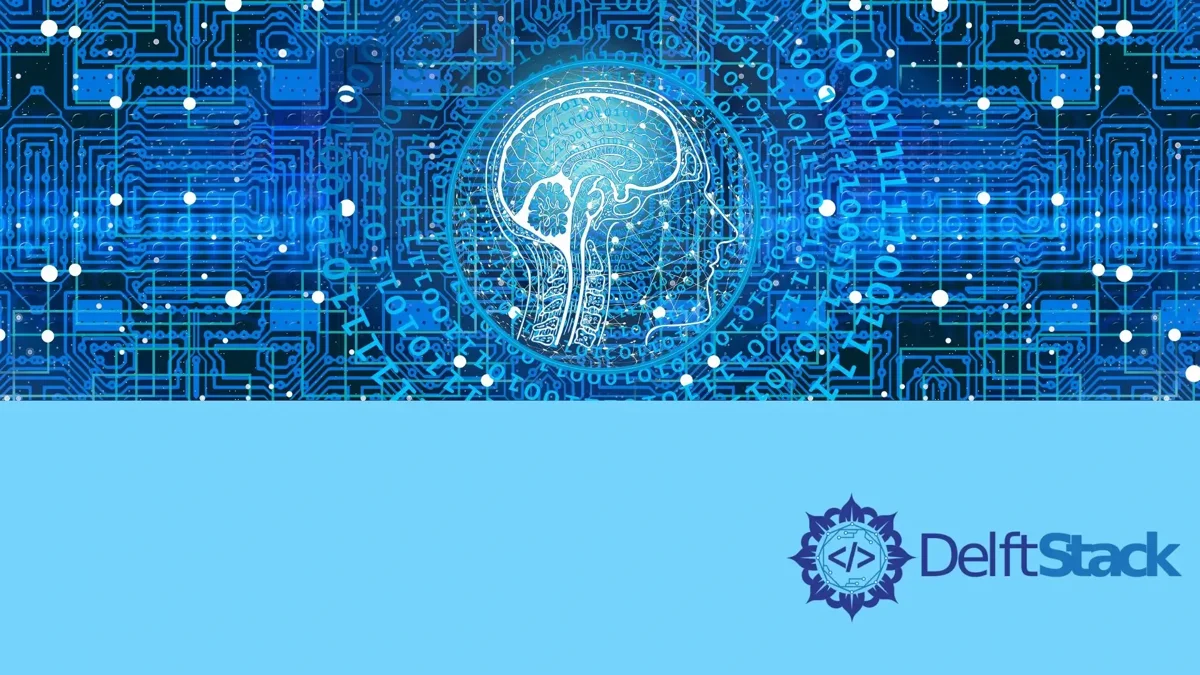
我們將來學習在 Python 中如何建立集合,以及如何增加和刪除其中的元素。
集合包含了不重複以及沒有排序的元素。
集合是可變資料型別,所以你可以增加或者刪除集合中的元素。
建立集合
集合可以通過大括號 {}
來建立,裡面的元素用逗號 ,
來分開。你也可以通過 Python 的內建函式 set()
來建立集合。
集合中的元素可以為不同的資料型別,而且元素必須是不可變資料型別,比如,元組可以作為集合的元素,但是集合、列表或者字典就不能是集合的元素。
>>> x = {3, 5, 7, 2, 4, 5}
>>> print(x) #prints the set variable
{2, 3, 4, 5, 7}
>>> print(type(x)) #checking type of x
<class 'set'>
你可以通過 set()
函式 with empty parameters to create an empty list. If you write {}
to create an empty object, it is interpreted as a dictionary:
>>> x = {}
>>> print(type(x))
<class 'dict'>
>>> x = set()
>>> print(type(x))
<class 'set'>
更新集合
索引和切片操作不能用來更新集合中的元素,因為集合不是一個有序的資料型別。可以通過 add()
方法來向集合中新增一個新元素,如果你想新增多個元素的話,可以使用 update()
方法。
>>> s = {2, 4}
>>> print(s)
{2, 4}
>>> s.add(6)
>>> print(s)
{2, 4, 6}
>>> s.update([2, 4, 6])
>>> print(s)
{2, 4, 6}
>>> s.update([5,6], {7, 8, 9})
>>> print(s)
{2, 4, 5, 6, 7, 8, 9}
刪除集合中的元素
集合可以通過 discard()
或 remove()
方法來刪除其中的某個元素。discard()
和 remove()
方法的差別在於當要去除的元素在集合中不存在時,discard()
不會報錯,而 remove()
會產生錯誤資訊。
>>> s = {2, 4, 5, 6, 7, 8, 9}
>>> print(s)
{2, 4, 5, 6, 7, 8, 9}
>>> s.discard(6)
>>> print(s)
{2, 4, 5, 7, 8, 9}
>>> s.remove(8)
>>> print(s)
{2, 4, 5, 7, 9}
>>> s.discard(6) #no error
>>> s.remove(8) #generated an error
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
s.remove(8)
KeyError: 8
你可以通過 pop()
方法來刪除集合中的一個元素,但 pop()
方法刪除的元素可能是集合中的任意的一個元素。
>>> s = set("Python")
>>> s
{'o', 'n', 'h', 'P', 'y', 't'}
>>> s.pop()
o
>>> s.pop()
n
>>> s
{'h', 'P', 'y', 't'}
>>> s.clear()
>>> print(s)
set()
clear()
方法是用來刪除集合中的所有元素。
>>> s = set("Python")
>>> s.clear()
>>> s
set()
集合的操作
集合的操作方法包括以下幾種,比如 union
、intersection
、difference
和 symmetric_difference
。
假設你有下面兩個集合 x
和 y
:
>>> x = {1, 2, 3, 4, 5, 6}
>>> y = {7, 8, 9, 10, 11, 12}
並集
兩個集合的並集是包含兩集合中所有元素的集合,Python 中可以使用 |
操作符或者 union()
方法來求兩集合的並集。
>>> print(x | y)
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12}
#using union() method on x
>>> x.union(y)
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12}
#union on y
>>> y.union(x)
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12}
交集
兩個集合的並集是在兩集合中都有的元素的集合,Python 中可以使用 &
操作符或者 intersection()
方法來求兩集合的交集。
>>> x = {1, 2, 3, 4, 5, 6}
>>> y = {7, 8, 9, 2, 6, 1}
>>> print(x & y)
{1, 2, 6}
#using intersection() method on x
>>> x.intersection(y)
{1, 2, 6}
#intersection on y
>>> y.intersection(x)
{1, 2, 6}
差集
集合 x
和 y
的差集是元素在 x
中存在,而在 y
中不存在的元素的集合,比如,x - y
中的元素在集合 x
而不在集合 y
中。Python 可以使用操作符 -
或者 difference()
方法來求差集。
>>> x = {1, 2, 3, 4, 5, 6}
>>> y = {7, 8, 9, 2, 6, 1}
>>> print(x - y)
{3, 4, 5}
#using difference() method on x
>>> x.difference(y)
{3, 4, 5}
#diference on y
>>> y.difference(x)
{8, 9, 7}
對稱差集
對稱差集是其中的元素不同時在兩個集合中出現的集合,Python 求對稱差集可以使用^
操作符或者 symmetric_difference()
方法。
>>> x = {1, 2, 3, 4, 5, 6}
>>> y = {7, 8, 9, 2, 6, 1}
>>> print(x ^ y)
{3, 4, 5, 7, 8, 9}
#using symmetric_difference() method on x
>>> x.symmetric_difference(y)
{3, 4, 5, 7, 8, 9}
#symmetric_diference on y
>>> y.symmetric_difference(x)
{3, 4, 5, 7, 8, 9}
集合方法
方法 | 描述 |
---|---|
add() |
增加一個元素到集合 |
clear() |
清空整個集合 |
copy() |
返回集合的拷貝(淺拷貝) |
difference() |
求差集 |
difference_update() |
求差集並且將集合更新為該差集 |
discard() |
從集合中刪除某一元素 |
intersection() |
求交集 |
intersection_update() |
求交集並且將集合更新為該交集 |
isdisjoint() |
當兩個集合沒有交集時,返回 True |
issubset() |
當另外一個集合包含此集合時,返回 True |
issuperset() |
當該集合包含另外一個集合時,返回 True |
pop() |
從集合彈出一任意元素 |
remove() |
從集合中刪除某一元素,假如集合中不存在該元素,報錯 |
symmetric_difference() |
求對稱交集 |
symmetric_difference_update() |
求對稱交集,並將該集合更新為此對稱交集 |
union() |
求並集 |
update() |
將集合更新為此集合和引數集合的並集 |
集合其他操作
集合成員檢查
可以使用 in
關鍵字來檢查某元素是否存在在集合中。
>>> s = set("Blue")
>>> print('o' in s)
False
>>> print('l' in s)
True
遍歷集合
你可以通過 for
迴圈來遍歷集合。
>>> for i in set("Blue"):
print(i)
B
l
u
e
適用於集合的內建函式
以下是 Python 中適用於集合的內建函式的列表
函式 | 描述 |
---|---|
all() |
如果集合中有所有元素為 True 返回 True ,當集合為空時,返回 True |
any() |
如果集合中有一個元素為 True 返回 True ,當集合為空時,返回 False |
enumerate() |
返回所有元素的索引和元素本身,索引和元素組成一個元組;最終返回的是一個 enumerate 型別 |
len() |
返回集合中的元素數目或者集合的長度 |
set() |
定義一個集合 |
max() |
返回集合中元素的最大值 |
min() |
返回集合中元素的最小值 |
sorted() |
返回一個包含集合所有元素的排序列表 |
sum() |
返回集合中所有元素的總和 |
Python 不可變集合
不可變集合是一種特殊集合,它在被賦值後元素不能被更新和修改。不可變集合是不可變型別的集合。
集合是可變型別的,所以你無法把它作為詞典的鍵,但因為不可變集合是不可變型別的,所以它是可以作為字典的鍵的。
不可變集合可以用 frozenset()
來建立,fronzenset
支援以下的方法:
copy()
difference()
intersection()
isdisjoint()
issubset()
issuperset()
symmetric_difference()
union()
>>> x = frozenset([2,6,3,9])
>>> y = frozenset([6,1,2,4])
>>> x.difference(y)
frozenset({9, 3})