How to Fix Python Return Outside Function Error
- Understanding the Error
- Method 1: Correcting Indentation
- Method 2: Ensuring Proper Function Definition
- Method 3: Avoiding Return Statements in Global Scope
- Conclusion
- FAQ
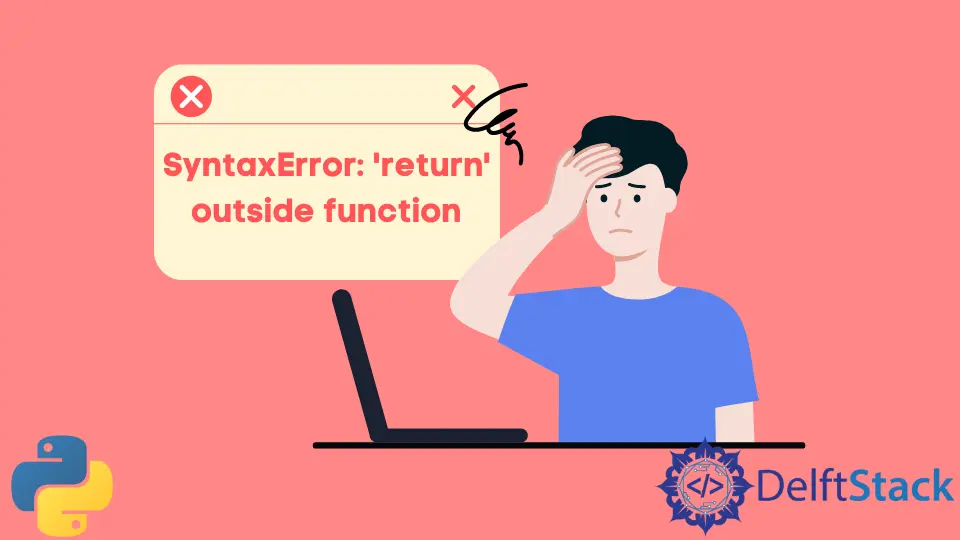
When working with Python, encountering the “return outside function” error can be frustrating, especially for beginners. This error typically arises when the return
statement is used outside of a function context, leading to confusion. Understanding how to resolve this issue is crucial for writing effective Python code.
In this article, we will explore practical methods to fix the “return outside function” error, providing clear code examples along the way. Whether you’re debugging your own code or helping a friend, these insights will help you grasp the concept better and enhance your Python programming skills.
Understanding the Error
Before diving into solutions, it’s essential to understand why this error occurs. The return
statement is designed to send a value back to the caller of a function. If you attempt to use it outside of a function, Python will raise a SyntaxError
. This usually happens when the indentation is off or when the return
statement is mistakenly placed in the wrong part of your code.
Let’s take a look at a common scenario that leads to this error.
def my_function():
print("Hello, World!")
return my_function()
Output:
SyntaxError: 'return' outside function
In this example, the return
statement is incorrectly placed outside the function definition, leading to the error.
Method 1: Correcting Indentation
One of the most common causes of the “return outside function” error is improper indentation. Python relies heavily on indentation to define the structure of the code, including which statements belong to which functions. To fix this error, ensure that your return
statement is correctly indented within the function block.
Here’s an example of how to correct the indentation.
def my_function():
print("Hello, World!")
return "Function finished"
result = my_function()
print(result)
Output:
Hello, World!
Function finished
In this corrected version, the return
statement is properly indented within the my_function
definition. This allows the function to execute without error, returning the specified string when called.
Indentation is crucial in Python, as it defines the scope of your functions. Always check your indentation levels if you encounter this error, as it’s often the simplest fix.
Method 2: Ensuring Proper Function Definition
Another common mistake that leads to this error is failing to define a function correctly. If you forget to include the def
keyword or accidentally misspell the function name, Python will not recognize the intended function context for the return
statement.
Here’s an example of a function defined incorrectly.
my_function():
print("Hello, World!")
return "Function finished"
Output:
SyntaxError: invalid syntax
To fix this, make sure to include the def
keyword before the function name.
def my_function():
print("Hello, World!")
return "Function finished"
result = my_function()
print(result)
Output:
Hello, World!
Function finished
In this corrected version, the function is properly defined with the def
keyword. This allows the return
statement to work as intended, providing a seamless execution of the function. Always double-check your function definitions to ensure they follow the correct syntax.
Method 3: Avoiding Return Statements in Global Scope
Using a return
statement in the global scope will also trigger the “return outside function” error. The return
statement must be contained within a function, and attempting to use it outside will lead to a syntax error.
Here’s an example that demonstrates this error.
print("Hello, World!")
return "Function finished"
Output:
SyntaxError: 'return' outside function
To resolve this, encapsulate the return
statement within a function.
def my_function():
print("Hello, World!")
return "Function finished"
result = my_function()
print(result)
Output:
Hello, World!
Function finished
In this example, the return
statement is now properly placed within the my_function
definition. By doing so, we avoid the syntax error and allow the function to execute correctly. Always remember that return
statements should only be used inside functions.
Conclusion
Fixing the “return outside function” error in Python is a straightforward process once you understand the underlying causes. By ensuring proper indentation, correctly defining your functions, and avoiding the use of return
statements in the global scope, you can prevent this error from disrupting your coding experience. As you continue to practice and refine your Python skills, these tips will help you write cleaner, more efficient code. Remember, programming is a journey, and every error is an opportunity to learn and grow.
FAQ
-
What causes the “return outside function” error in Python?
The error occurs when a return statement is used outside of a function context, usually due to improper indentation or incorrect function definitions. -
How can I avoid this error in my Python code?
Ensure that your return statements are always placed within function definitions and check your indentation levels to confirm that they are correct. -
Is there a way to debug this error easily?
Yes, reviewing your code for proper function definitions and indentation can help you quickly identify the source of the error. -
Can I use return statements in global scope?
No, return statements must always be used within a function. Attempting to use them in the global scope will lead to a syntax error. -
What should I do if I encounter other syntax errors?
Carefully read the error message and review your code for common mistakes, such as missing colons, parentheses, or incorrect indentation.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Function
- How to Exit a Function in Python
- Optional Arguments in Python
- How to Fit a Step Function in Python
- Built-In Identity Function in Python
- Arguments in the main() Function in Python
- Python Functools Partial Function
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python