How to Exit a Function in Python
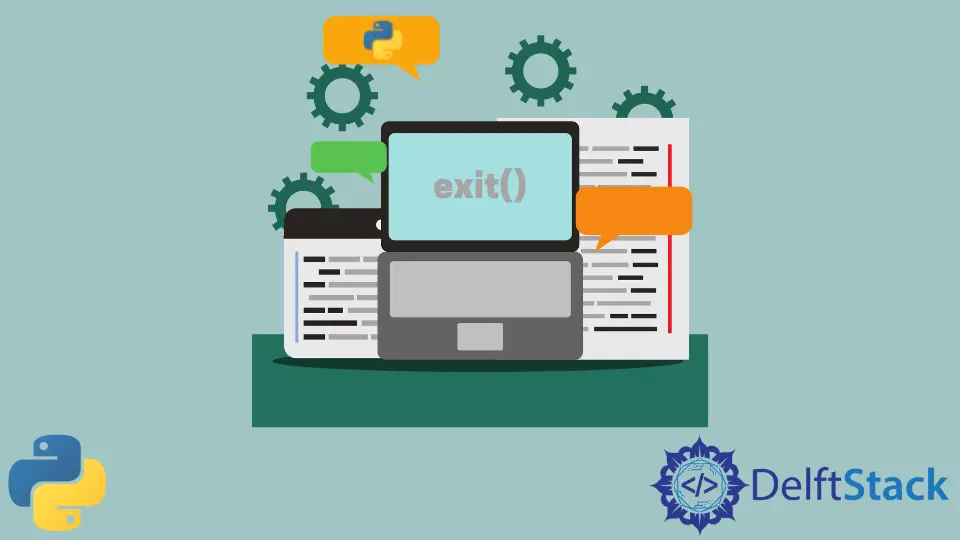
Every program has some flow of execution. A flow is nothing but how the program is executed. The return
statement is used to exit Python’s function, which can be used in many different cases inside the program. But the two most common ways where we use this statement are below.
- When we want to return a value from a function after it has exited or executed. And we will use the value later in the program.
def add(a, b):
return a + b
value = add(1, 2)
print(value)
Output:
3
Here, it returns the value computed by a+b
and then stores that value which is 3
, inside the value
variable.
- When we want to stop the execution of the function at a given moment.
def add(a, b):
if a == 0:
return
elif b == 0:
return
else:
sum = a + b
return sum
value = add(0, 2)
print(value)
Output:
None
Here, if the values of either a
or b
are 0
, it will directly return without calculating the numbers’ sum. If they are not 0
then only it will calculate and return the sum
.
Now, if you implement this statement in your program, then depending upon where you have added this statement in your program, the program execution will change. Let’s see how it works.
Implicit Return Type in Python
Suppose we have a function inside which we have written using an if
statement, then let’s see how the program behaves.
def solution():
name = "john"
if name == "john":
print("My name ", name)
solution()
Output:
My name john
The solution()
function takes no arguments. Inside it, we have a variable called name
and then check its value matches the string john
using the if
statement. If it matches, we print the value of the name
variable and then exit the function; otherwise, if the string doesn’t match, we will simply exit it without doing anything.
Here, you might think that since there is no return
statement written in the code, there is no return
statement present. Note that the return statement is not compulsory to write. Whenever you exit any Python function, it calls return
with the value of None
only if you have not specified the return
statement. The value None
means that the function has completed its execution and is returning nothing. If you have specified the return
statement without any parameter, it is also the same as return None
. If you don’t specify any return type inside a function, then that function will call a return
statement. It is called an implicit return type in Python.
Explicit Return Type in Python
Whenever you add a return
statement explicitly by yourself inside the code, the return type is called an explicit return type. There are many advantages of having an explicit return type, like you can pass a value computed by a function and store it inside a variable for later use or stop the execution of the function based on some conditions with the help of a return
statement and so on. Let’s see an example of the explicit type in Python.
def Fibonacci(n):
if n < 0:
print("Fibo of negative num does not exist")
elif n == 0:
return 0
elif n == 1 or n == 2:
return 1
else:
return Fibonacci(n - 1) + Fibonacci(n - 2)
print(Fibonacci(0))
Output:
0
This is a program for finding Fibonacci numbers. Notice how the code is return with the help of an explicit return
statement. Here, the main thing to note is that we will directly return some value if the number passed to this function is 2
or lesser than 2
and exit the function ignoring the code written below that. We will only execute our main code (present inside the else
block) only when the value passed to this function is greater than 2
.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn