Optional Arguments in Python
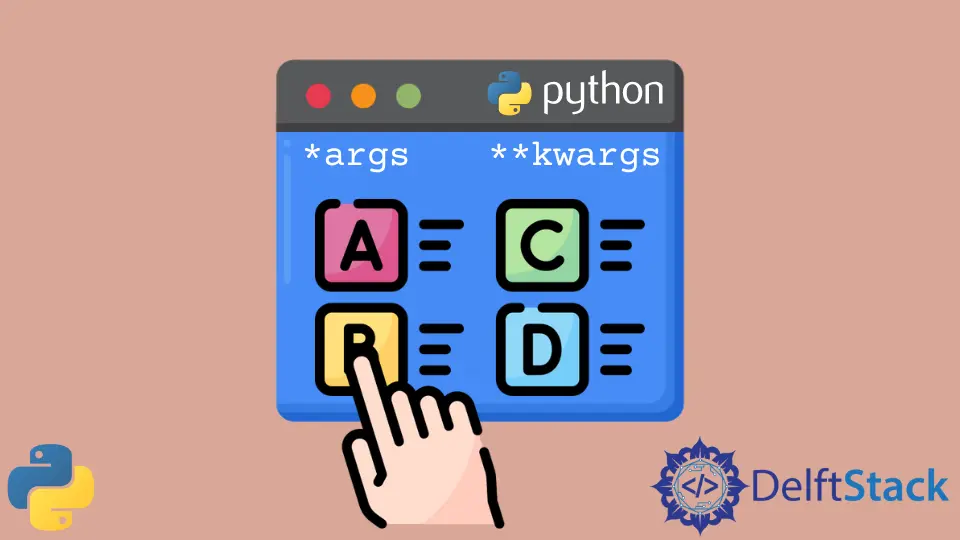
In python, there is something called a default argument. It is also known as optional argument or optional parameter in Python. An argument or a parameter both mean the same thing. You can use these words interchangeably. An argument or a parameter is an input that a function takes.
Python Function With Multiple Optional Arguments
Whenever you call a function, you have to pass some arguments to that function depending upon whether a function takes any arguments or not. It’s not mandatory to pass an argument to a function. A function can take no argument or can take any number of arguments (depending upon how the function is defined). There are two ways in which we can pass in multiple arguments.
Use *args
(Non-Keyword Arguments)
# Function Defination
def myfunction(first_name, last_name, *args):
print(first_name)
print(last_name)
for argument in args:
print(argument)
# Calling the Function
myfunction("Tim", "White", 999888999, 30)
Output:
Tim
White
999888999
30
Use **kargs
(Keyword Arguments)
def myFunction(**kwargs):
# printing all values which are passed in as an argument
for key, value in kwargs.items():
print("%s = %s" % (key, value))
# Calling the Function
myFunction(first_name="Tim", last_name="White", mob=99999999, age="30")
Output:
first_name = Tim
last_name = White
mob = 99999999
age = 30
The *args
and **kargs
both are variable-length arguments that are used to pass the variable number of arguments to a function.
When you create a function, you have to define how many parameters or arguments it will take as input. These are called formal arguments. And whenever you call that function, you have to pass some values to that function, and those values are called actual arguments.
Make Arguments Optional in Python
Let’s say you have a function that takes three arguments or parameters name
, number
, and age
as an input.
# Function Defination
def personalDetails(name, number, age):
print("This function takes 3 parameters as input")
If you want to call the function personalDetails
, you must pass all the 3 parameters as an input to that function.
def personalDetails(name, number, age):
print("This function takes 3 parameters as input")
print("Name: ", name)
print("Number: ", number)
print("Age: ", age)
# Calling the function
personalDetails("Adam", 987654321, 18)
Output:
This function takes 3 parameters as input
Name: Adam
Number: 987654321
Age: 18
Here, while calling a function, If you missed or forget to pass any of the parameters, you can get an error (TypeError: personalDetails missing a required positional argument
).
If you don’t want to pass your Age to the above function as an argument, you can use something called an optional argument. You can make any number of arguments or parameters optional in Python. To make an argument optional, you have to assign some default value to that argument.
Here, to make the age
argument optional, you can add a default value to the argument age
in the function definition to make it optional. In this case, let’s initialize it with 0
or any other value which you want. Now Python will consider this argument as an optional argument. So, Even though if you don’t pass any value to the age
parameter, the function will work, and it will use the default value, which in this case is 0
.
def personalDetails(name, number, age=0):
print("Age is now an optional argument.")
print("Age is: ", age)
# Calling the function
personalDetails("Sam", 123456789)
Output:
Age is now an optional argument.
Age is: 0
Even if you want to specify the value of the age
argument while calling the function personalDetails
, you can do that. And now it will consider the new value which you have specified instead of the default value.
def personalDetails(name, number, age=0):
print("Age is now an optional argument.")
print("Age is: ", age)
# Calling the function
personalDetails("Sam", 123456789, 25)
Output:
Age is now an optional argument.
Age is: 25
Conclusion
Whenever you initialize any argument with a default value, it is known as a default argument. This eventually makes that argument optional, too, as now it’s not mandatory to pass in a value to that argument while calling the function. This is known as an optional argument or optional parameter in Python.
But if you have passed some value into the optional argument while calling the function, then the function will take that new value instead of the default value.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn