Python Functools Partial Function
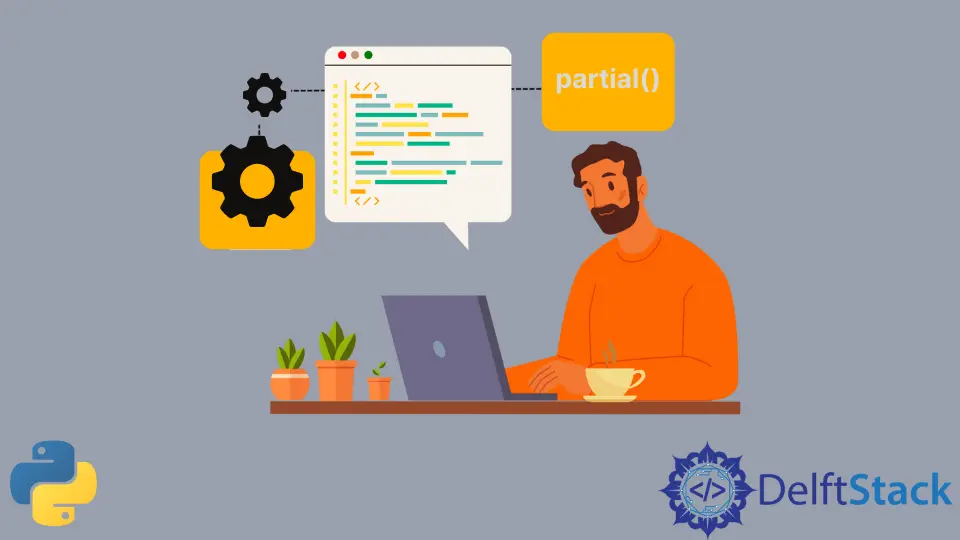
Functools are the standard framework used in Python when working with high-order functions that act on or return other functions, and it helps to extend or use the functions without rewriting them. Using Functools makes the code readable and easy maintenance with high efficiency.
This library contains two main functions: partial
and partialmethod
. The partialmethod
is a non-callable method descriptor of an already defined function for specific arguments and returns a new partialmethod
descriptor.
Let’s have a look at the partial()
function.
Use the Functools partial()
Function in Python
The partial()
function is a higher-order function that takes a function as input and returns a function that can use in a program like other functions. When there is a function with lots of arguments, but we need to change only one or two arguments every time we use that function, the partial()
function, which comes along with the Functools library, aids in such situations.
With the help of the partial()
function, we can freeze a specific number of arguments from a function and create a new simplified one to return a new partial()
object with positional arguments and keywords. Therefore, it prevents us from rewriting the original function.
Overall, partial()
is a handy tool, especially in applying various inputs to a single object or wrapping one of the function’s arguments to be constant. So, the final result of using the partial()
function will print out an elegant, readable, and reusable code.
Before using the partial()
function, we must import it from the Functools library. Let’s see how we implement the function.
from functools import partial
As we have imported the partial
library using the above code, we can use the partial
command as below. Then we can call over the partial()
function as we want, and it outputs the function similar to the defined function along with a fixed value.
Below is the standardized partial()
function and its attributes.
partial(func, /, *args, **keywords)
The partial
function consists of three main attributes: function, arguments, and keywords.
partial.func
(function name) - A callable object or a function that returns the name and hexadecimal address of the parent function.partial.args
(positional arguments) - This attribute returns the pre-assigned arguments to thepartial()
function.partial.keyword
(keyword arguments) - This attribute returns the pre-assigned keywords to thepartial()
function.
Let’s define an example using the partial()
function and its attributes.
Full Code:
from functools import partial
# original function
def multiply(a, b):
return a * b
# partial function to multiply a as 2
multiplication1 = partial(multiply, 2)
print(multiplication1(4))
# partial function to multiply b as 7
multiplication2 = partial(multiply, b=7)
print(multiplication2(3))
# simple multiply function with two variables
print(multiply(5, 2))
# partial function with two values and multiply function
multiplication3 = partial(multiply, 2, 5)
print(multiplication3())
# defining some attributes in multiplication1, multiplication2 multiplication3
print("Function used in multiplication1 :", multiplication1.func)
print("Keywords for multiplication2 :", multiplication2.keywords)
print("Arguments for multiplication3 :", multiplication3.args)
Output:
At first, we imported the partial()
function from functools
and defined a function named multiply()
with two variables as a
and b
. This multiply
function multiplies two numbers (a,b)
together.
from functools import partial
def multiply(a, b):
return a * b
In the below code chunk, the partial()
function is called and outputs a function object multiply()
along with a fixed value of 2
. Here, the partial()
function calls the multiply()
with a
as 2
.
When printing, it assigns 4
for b
and prints the result as 8
.
multiplication1 = partial(multiply, 2)
print(multiplication1(4))
Here, when the partial()
function gets called, it prints out the multiply()
function and the value assigned for b
as 3.
It has specified the value for the variable b
by skipping the parameter of the original function.
When printing, it has defined a value for a
as 3
and displays the output as 21
.
multiplication2 = partial(multiply, b=7)
print(multiplication2(3))
The partial()
function is not essential when calling the function with values for two variables. As usual, it takes 5
for a
, 2
for b
, and prints out the result as 10
.
print(multiply(5, 2))
As the original function has two parameters when calling the partial()
function, it will not be an issue, and it takes multiply()
with a
as 2
and b
as 5
. Then, it displays the result as 10
.
multiplication3 = partial(multiply, 2, 5)
print(multiplication3())
The below code chunk displays the function used in the function multiplication1
, keywords in multiplication2
, and arguments in multiplication3
, respectively. So, the function used in multiplication1
is multiply()
, and its hexadecimal value is 000001E523A6E4C0
.
In multiplication2
, we have used {'b': 7}
as keywords while 2, 5
are the arguments used in multiplication3
.
print("Function used in multiplication1 :", multiplication1.func)
print("Keywords for multiplication2 :", multiplication2.keywords)
print("Arguments for multiplication3 :", multiplication3.args)
Conclusion
This article discussed the partial()
function of the functools
library. As you saw in the previous examples, using the partial()
function makes our code more readable, elegant, and fast.
If you find a function that freezes one or more arguments, the partial()
function will be the best for that situation.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.