Python 中的可选参数
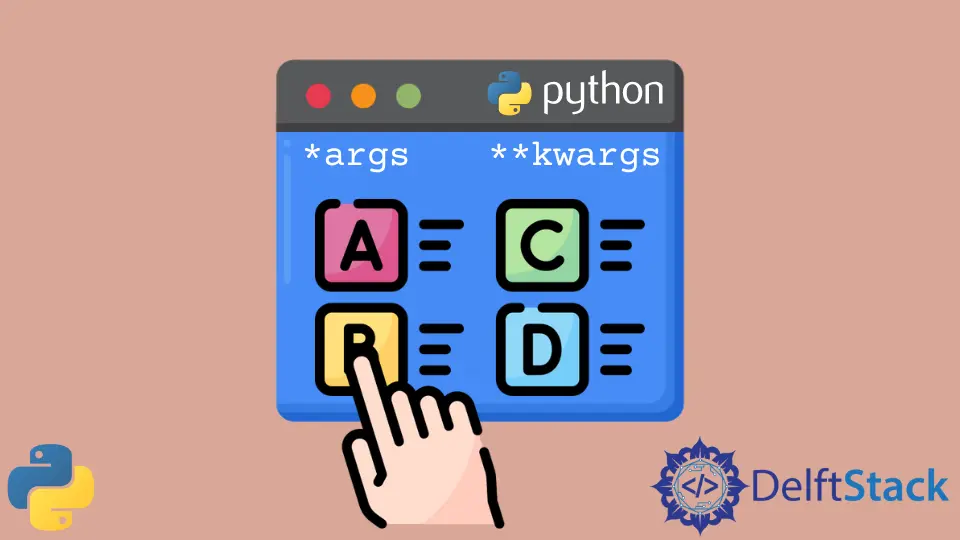
在 Python 中,有一种东西叫做默认参数。它在 python 中也被称为可选参数或可选参数。参数是指一个函数的输入。
Python 中有多个可选参数的函数
每当你调用一个函数的时候,你必须向该函数传递一些参数,这取决于函数是否接受任何参数。传递参数给一个函数并不是强制性的,一个函数可以不接受任何参数,也可以接受任何数量的参数(取决于函数如何使用)。一个函数可以不接受任何参数,也可以接受任何数量的参数(取决于函数是如何定义的)。我们有两种方式可以传递多个参数。
使用*args
(非关键字参数)
# Function Defination
def myfunction(first_name, last_name, *args):
print(first_name)
print(last_name)
for argument in args:
print(argument)
# Calling the Function
myfunction("Tim", "White", 999888999, 30)
输出:
Tim
White
999888999
30
使用**kargs
(关键字参数)
def myFunction(**kwargs):
# printing all values which are passed in as an argument
for key, value in kwargs.items():
print("%s = %s" % (key, value))
# Calling the Function
myFunction(first_name="Tim", last_name="White", mob=99999999, age="30")
输出:
first_name = Tim
last_name = White
mob = 99999999
age = 30
*args
和**kargs
都是可变长度的参数,用于向函数传递可变数量的参数。
当你创建一个函数时,你必须定义它将接受多少参数或参数作为输入。这些被称为形式参数。而每当你调用该函数时,你必须向该函数传递一些值,这些值被称为实际参数。
在 Python 中让参数成为可选项
比方说,你有一个函数,它接受三个参数 name
,number
和 age
作为输入。
# Function Defination
def personalDetails(name, number, age):
print("This function takes 3 parameters as input")
如果你想调用函数 personalDetails
,你必须把这 3 个参数作为输入传给该函数。
def personalDetails(name, number, age):
print("This function takes 3 parameters as input")
print("Name: ", name)
print("Number: ", number)
print("Age: ", age)
# Calling the function
personalDetails("Adam", 987654321, 18)
输出:
This function takes 3 parameters as input
Name: Adam
Number: 987654321
Age: 18
在这里,当调用一个函数时,如果你遗漏或忘记传递任何一个参数,你会得到一个错误(TypeError: personalDetails missing a required positional argument
)。
如果你不想把你的年龄作为参数传给上面的函数,你可以使用一个叫做可选参数的东西。在 Python 中,你可以使任何数量的参数或参数成为可选的。要使一个参数成为可选的,你必须给这个参数分配一些默认值。
在这里,为了使 age
参数成为可选的,你可以在函数定义中为 age
参数添加一个默认值,使其成为可选的。在这种情况下,让我们用 0
或任何其他你想要的值来初始化它。现在,Python 会将这个参数视为一个可选参数。所以,即使你不给 age
参数传递任何值,函数也会工作,它会使用默认值,在本例中是 0
。
def personalDetails(name, number, age=0):
print("Age is now an optional argument.")
print("Age is: ", age)
# Calling the function
personalDetails("Sam", 123456789)
输出:
Age is now an optional argument.
Age is: 0
即使你想在调用函数 personalDetails
时指定 age
参数的值,你也可以这样做。现在它将考虑你指定的新值,而不是默认值。
def personalDetails(name, number, age=0):
print("Age is now an optional argument.")
print("Age is: ", age)
# Calling the function
personalDetails("Sam", 123456789, 25)
输出:
Age is now an optional argument.
Age is: 25
结论
每当你用一个默认值初始化任何参数时,它就被称为默认参数。这最终使得该参数也变成了可选的,因为现在在调用函数的时候,并不是必须给该参数传入一个值。这在 Python 中被称为可选参数或可选参数。
但是如果你在调用函数的时候向可选参数中传递了一些值,那么函数就会取这个新的值而不是默认值。
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn