How to Open All the Files in a Directory in Python
-
Open All Files in a Folder/Dictionary Using
os.walk()
in Python -
Open All the Files in a Folder/Directory With the
os.listdir()
Function in Python -
Open All the Files in a Folder/Directory With the
glob.glob()
Function in Python -
Open All the Files in a Folder/Directory With the
os.scandir()
Function in Python - Conclusion
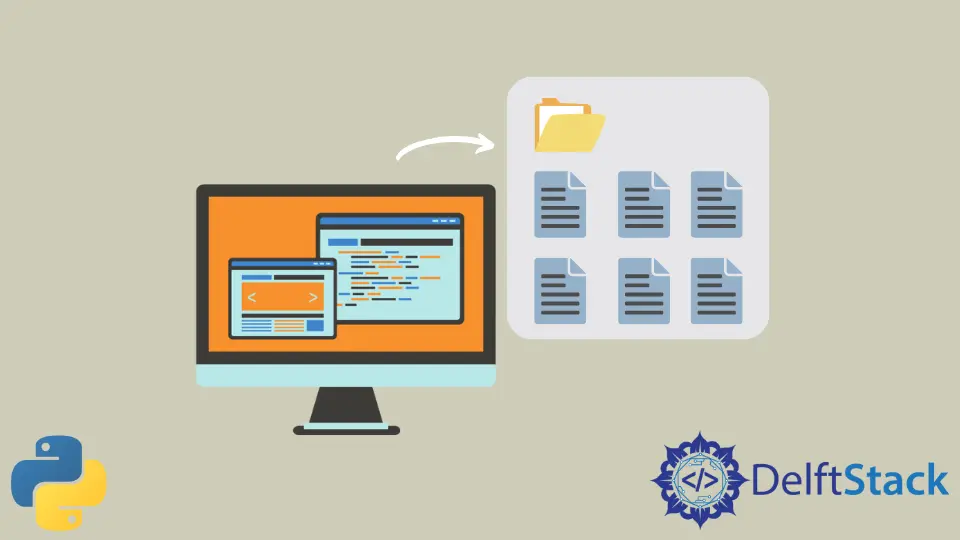
This comprehensive guide delves into various methods to open and read files in a directory, leveraging Python’s robust OS
module and its diverse functions. We will explore the use of os.walk()
, os.listdir()
, glob.glob()
, and the efficient os.scandir()
method.
Each of these functions offers unique advantages and approaches to file handling, addressing different requirements and scenarios. From traversing directory trees to pattern matching and efficient directory scanning, this article serves as an invaluable resource for Python developers.
Open All Files in a Folder/Dictionary Using os.walk()
in Python
Various OS
modules in Python programming allow multiple methods to interact with the file system. It has a walk()
function that will enable us to list all the files in a specific path by traversing the directory either bottom-up or top-down and returning three tuples - root
, dir
, and files
.
In the above syntax, r
is to read the root folder or directory, and the parameter pathname
is the path of the folder.
Example:
import os
def read_files(directory):
for root, dirs, files in os.walk(directory):
for filename in files:
file_path = os.path.join(root, filename)
with open(file_path, "r") as file:
print(f"Contents of {filename}:")
print(file.read())
print("----------")
# Replace '/path/to/your/directory' with the path of your directory
read_files("directory")
We begin by importing the os
module, as it is essential for file and directory operations.
We have designed the read_files
function to take a directory path as an argument.
Within the function, we use os.walk
to iterate over the directory tree. For each directory root
, we list its subdirectories and files.
We open each file, read its contents, and print them. We utilize the os.path.join
function to construct the full path to each file.
Output:
Open All the Files in a Folder/Directory With the os.listdir()
Function in Python
The listdir()
function inside the os
module is used to list all the files inside a specified directory. This function takes the specified directory path as an input parameter and returns the names of all the files inside that directory.
We can iterate through all the files inside a specific directory using the os.listdir()
function and open them with the open()
function in Python.
The following code example shows us how we can open all the files in a directory with the os.listdir()
and open()
functions.
import os
def read_files_in_directory(directory):
for entry in os.listdir(directory):
full_path = os.path.join(directory, entry)
if os.path.isfile(full_path):
with open(full_path, "r") as file:
print(f"Contents of {entry}:")
print(file.read())
print("----------")
# Replace '/path/to/your/directory' with your directory path
read_files_in_directory("directory")
We begin by importing os
, which provides the necessary functions for interacting with the file system.
We define read_files_in_directory
to take a directory path as its argument.
Using the os.listdir
function, we list all entries in the specified directory, processing each entry in a for
loop.
For each entry, we construct the full path using os.path.join
. This step is necessary because os.listdir
returns only the names, not the full paths.
Before attempting to open an entry, we check if it is a file using os.path.isfile
. This check is crucial as the directory may contain subdirectories, and attempting to open a directory as a file would cause an error.
If the entry is a file, we open it in read mode.
Finally, we read and print the contents of each file, providing a clear view of what each file contains.
Output:
Open All the Files in a Folder/Directory With the glob.glob()
Function in Python
The glob
module is used for listing files inside a specific directory. The glob()
function inside the glob
module is used to get a list of files or subdirectories matching a specified pattern inside a specified directory.
The glob.glob()
function takes the pattern as an input parameter and returns a list of files and subdirectories inside the specified directory.
We can iterate through all the text files inside a specific directory using the glob.glob()
function and open them with the open()
function in Python. The following code example shows us how we can open all files in a directory with the glob.glob()
and open()
functions.
import glob
def read_files(pattern):
for file_path in glob.glob(pattern):
with open(file_path, "r") as file:
print(f"Contents of {file_path}:")
print(file.read())
print("----------")
# Replace '/path/to/your/directory/*.txt' with your pattern
read_files("directory/*.txt")
We start by importing the glob
module, an essential tool for pattern matching in file paths.
In the read_files
function, we design it to accept a pattern as its argument, specifying the criteria for file selection.
Using the glob.glob
function, we retrieve a list of file paths that match the specified pattern. The loop then iterates over each file path that satisfies the criteria.
For each file path returned by glob.glob
, we open the file in read mode and read its contents.
Finally, we print out the contents of each file, along with its path. This approach aids in identifying the contents of each specific file that matches the specified pattern.
Output:
Open All the Files in a Folder/Directory With the os.scandir()
Function in Python
The scandir
method, introduced in Python 3.5, is part of the os
module and provides a more efficient way to list entries in a directory. It’s a high-level and more performant alternative to older functions like os.listdir
, especially when you need additional file information like file type or attributes.
Here’s how you can use scandir
to open and read all files in a specific directory.
import os
def read_files_in_directory(directory):
with os.scandir(directory) as entries:
for entry in entries:
if entry.is_file():
with open(entry.path, "r") as file:
print(f"Contents of {entry.name}:")
print(file.read())
print("----------")
# Replace '/path/to/your/directory' with your directory path
read_files_in_directory("directory")
We start our script by importing the os
module, which includes the useful scandir
function.
In defining our function, read_files_in_directory
, we set it to take a single argument, directory, specifying the directory we wish to scan.
We utilize scandir
by opening the directory and initializing an iterator, entries, with the statement with os.scandir(directory) as entries
. This context manager is efficient as it ensures the directory is closed after processing, thereby reducing resource usage.
Our function then iterates over each entry in the directory, where an entry is an object representing a file or directory in the specified path.
For file checking, we use if entry.is_file():
to determine if the current entry is a file. This distinction is crucial because scandir
lists both files and directories.
When an entry is a file, we open it in read mode, read its contents, and print them. We use entry.name
to get the name of the file and entry.path
for the full path necessary to open the file.
Output:
Conclusion
Throughout this exploration of Python’s file-handling capabilities, we have uncovered the versatility and power of the OS
module and its various functions. From the depth-first search approach of os.walk()
to the straightforward listing capabilities of os.listdir()
, the pattern-matching utility of glob.glob()
, and the efficiency of os.scandir()
, we have navigated through a range of methods suited for different file-processing needs.
Each technique presents unique advantages, whether in handling large numbers of files, dealing with complex directory structures, or optimizing performance. This guide not only equips Python programmers with the tools to effectively manage file operations but also deepens the understanding of how Python interacts with the operating system.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python File
- How to Get All the Files of a Directory
- How to Append Text to a File in Python
- How to Check if a File Exists in Python
- How to Find Files With a Certain Extension Only in Python
- How to Read Specific Lines From a File in Python
- How to Check if File Is Empty in Python
Related Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python