How to Convert List to Set in Python
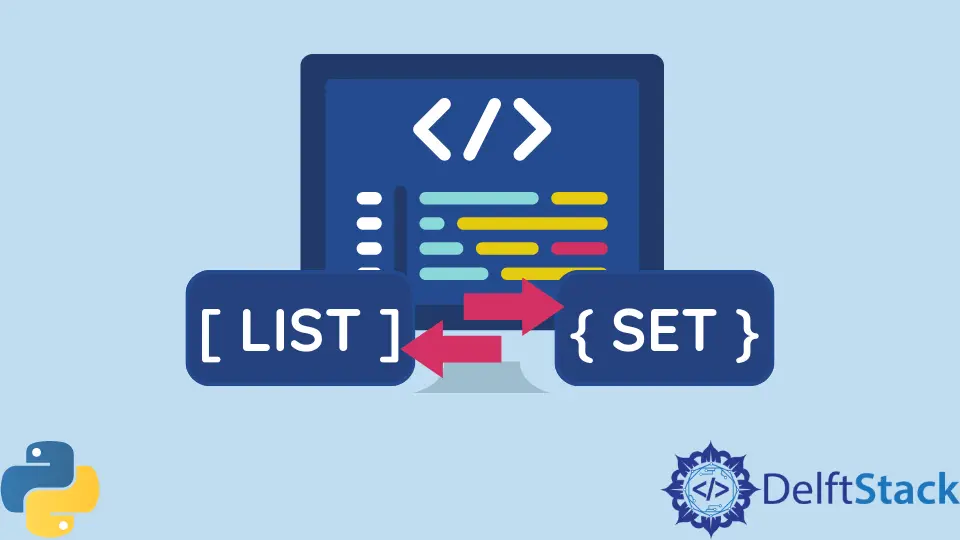
This tutorial demonstrates how to convert a list into a set in Python.
Difference Between a List and a Set
Lists and sets are standard Python data types that store values in sequence.
A Python List stores comma-separated values between square brackets. It’s mutable, which means that its items can be modified or changed. The thing about the Python List is that the items do not need to be of the same type.
Below is an example of what a Python List looks like:
list_1 = [1, 2, 3]
list_2 = ["Milk", "Bread", "Eggs"]
# List with multiple data types
list_3 = ["Chocolate", 15.25, 200]
Python sets are an unordered list of unique elements that may have different data types. The object stores comma-separated values and is encapsulated in curly braces {}
. It is iterable and mutable, yet it only stores immutable objects; this means that a set itself can be modified - we can add or remove elements; however, the elements inside the set must not change. We cannot modify or access the elements inside a set using slicing or indexing.
Here’s an example of what a Python Set looks like:
set_1 = {1, 2, 3}
set_2 = {"Milk", "Bread", "Eggs"}
# Sets with multiple data types
set_3 = {17.0, "Hello World", (10, 20, 30)}
Now, we know the differences between a Python List and a Set, let’s dive into converting a List into a Set.
Using the set()
Method to Convert the List to Set in Python
The simplest way to convert a list to a set is by using Python’s built-in method, the set()
method. Converting a List with duplicate values will be automatically removed by the set()
method and will retain the same unique elements as the initial List. The set()
method only accepts one parameter as an argument:
*iterable (optional) - a sequence (string, list, tuple, etc.) or collections of elements
Here’s the syntax:
set(iterable)
The set()
method returns:
- an empty set, if no arguments is passed.
- a constructed set
Example 1: Convert a Python List with Unique Values
# initialize a list
_list = [5, 4, 3, 1, 6, 2]
# convert list to set
converted = set(_list)
print(converted)
Output:
{1, 2, 3, 4, 5, 6}
Example 2: Convert List with Duplicate Values
# initialize a list
grocery_list = ["milk", "bread", "eggs", "bread", "hotdogs", "eggs"]
# convert list to set
converted = set(grocery_list)
print(converted)
Output:
{'eggs', 'bread', 'milk', 'hotdogs'}
As you can see, the converted
variable now only contains unique values.
Example 3: Convert List with Mixed Data Types
# initialize a list with mixed data types
grocery_list = ["milk", "bread", "eggs", ("Ham and Cheese", 2000), 19.43]
# convert the grocery_list to set
grocery_set = set(grocery_list)
# display the converted grocery_list
print(grocery_set)
Output:
{'milk', 'bread', 'eggs', ('Ham and Cheese', 2000), 19.43}
Python sets provide an array of methods you can choose from depending on your use-case, and not just convert a list into a set. There are set methods used to perform mathematical operations: union, intersection, difference, and symmetric difference. We can achieve this by using some defined Python set operators and methods.
Related Article - Python Set
- How to Join Two Sets in Python
- How to Add Values to a Set in Python
- How to Check if a Set Is a Subset of Another Set in Python
- How to Convert Set to String in Python
- Python Set Pop() Method
- How to Create a Set of Sets in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python