How to Make List of Even Numbers in Python
- What is an Even Number
-
Use a
for
Loop to Make a List of Even Numbers in Python -
Use a
while
Loop to Make a List of Even Numbers in Python - Use List Comprehension to Make a List of Even Numbers in Python
- Use Lambda Expression to Make a List of Even Numbers in Python
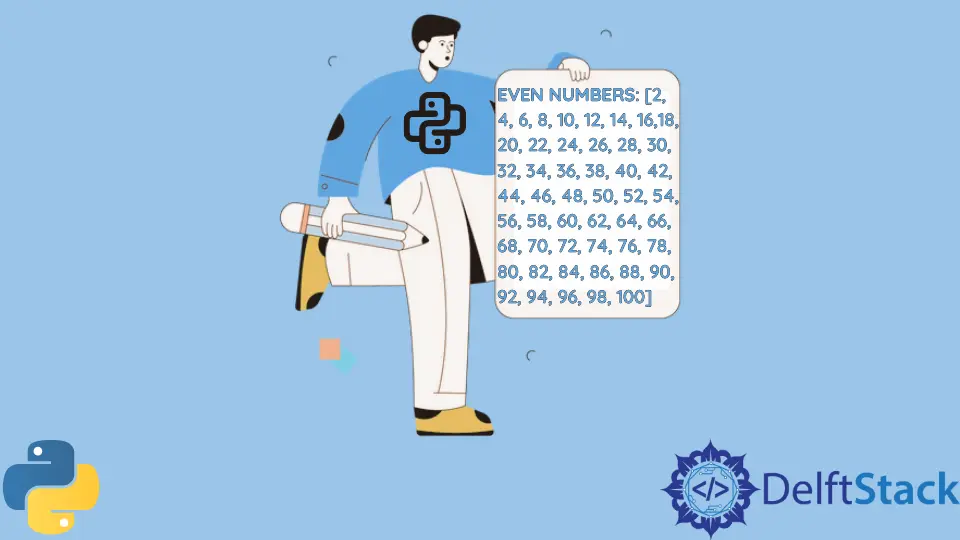
We will introduce a list of even numbers and different ways to create a list of even numbers in Python with examples.
What is an Even Number
This tutorial shows how to make a list of even numbers in Python. These questions are mostly asked in interview questions to test your programming skills.
This type of problem can also boost your knowledge and thinking process. You may all know what even numbers are, but let’s discuss them before getting started.
There are two ways to define an even number; the first is when a whole number that can be divided by 2 is known as an even number.
Another way of looking at it is if there is no remainder when we are trying to divide a whole number by 2, it is an even number. For example, 2, 4, 6, 8, 10, 12, 46, 80, and so on are all even numbers.
There are many methods to get even numbers from a list, but we will discuss only a few. Firstly, we will discuss how we can create a list of even numbers using the for
loop in Python.
Use a for
Loop to Make a List of Even Numbers in Python
As we all know, when we try to divide an even number by 2, there is no remainder.
We will use this concept to create a list of even numbers using the for
loop.
We will define a function EVEN_NUMBERS
that will take a num
and use it as a range to get the even numbers from that range and skip the zero values from being added to the list of even numbers shown below.
# python
def EVEN_NUMBERS(num):
EVEN = []
for i in range(num):
if i != 0:
if i % 2 == 0:
EVEN.append(i)
return EVEN
num = 101
print("Even Number: ", EVEN_NUMBERS(num))
Output:
The above result shows that it is easy to get a list of even numbers from a range using the for
loop.
We will go through another example in which we will use a while
loop to create a list of even numbers.
Use a while
Loop to Make a List of Even Numbers in Python
We will use the same concept but a while
loop to create a list of even numbers. First, we will define a function EVEN_NUMBERS
that will take the max
value of the range.
And we will define an array EVEN
that will store all the even numbers. We will create another variable, number
, that will increment after every while
loop.
# python
def EVEN_NUMBERS(max):
EVEN = []
number = 1
while number <= max:
if number % 2 == 0:
EVEN.append(number)
number = number + 1
print("Even Numbers: ", EVEN)
max = 10
EVEN_NUMBERS(max)
Output:
The above result shows that creating a list of even numbers is easy using the while
loop. We used a range to get all the even numbers in that range.
We will use another “list comprehension” method to create a list of even numbers in Python.
Use List Comprehension to Make a List of Even Numbers in Python
We can make a list of even numbers using an easy and compact syntax that can be used to make a list from a string or another list. List comprehension is a compact way of creating a new list by performing a certain function on all the elements of an existing list.
This method is a lot faster than the for
loop. As shown below, we will use the same concept to create a list of even numbers.
# python
even_list = [x for x in range(1, 100) if x % 2 == 0]
print("Even Numbers :", even_list)
Output:
The above result shows that it is quite easy and faster than the for
loop. We just wrote one line of code to create a list of even numbers inside a range of 1 to 100 using list comprehension.
We will go through our last tutorial method, lambda
, to create a list of even numbers in Python.
Use Lambda Expression to Make a List of Even Numbers in Python
We can use the lambda
function to get the even numbers from the given range in Python. The lambda
function is a single line function with no name and can take any number of arguments, but it only consists of a single-line expression.
Let’s use the same concept as shown below, but we will use a list of whole numbers, identify the even numbers, and save them in a new list.
# python
list1 = [20, 23, 48, 85, 96, 33, 51]
even_number = list(filter(lambda x: (x % 2 == 0), list1))
print("Even numbers in the list: ", even_number)
Output:
The above result shows that we can get the even numbers from a list of whole numbers containing both odd and even numbers using the lambda
function.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python