How to Create a List of Odd Numbers in Python
- Odd Numbers in Python
-
Use a
for
Loop to Get Odd Numbers in Python -
Use a
while
Loop to Get Odd Numbers in Python - Using List Comprehension to Get Odd Numbers in Python
- Use Lambda Expression to Get Odd Numbers in Python in Python
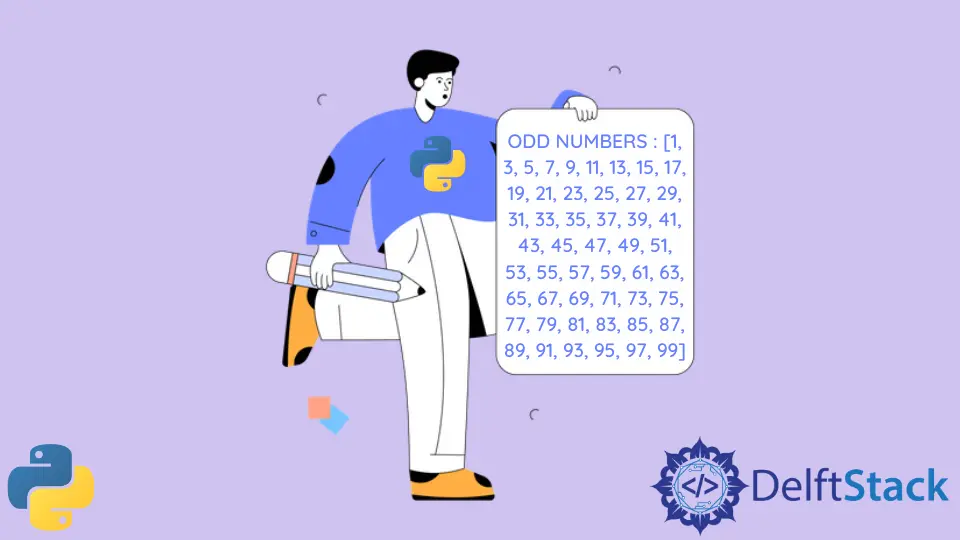
We will introduce in this article the different ways to create a list of odd numbers in Python.
Odd Numbers in Python
There are two ways to define an odd number, and the first one is when a whole number cannot be divided by 2. Another way is if there is a remainder of 1 when we try to divide a whole number by 2.
For example, 1, 5, 9, 11, 45, and so on are all odd numbers. There are many methods to get odd numbers from a list, but we will discuss only a few.
Use a for
Loop to Get Odd Numbers in Python
When we try to divide an odd number by 2, the remainder is 1. When we try to divide an even number by 2, the remainder is 0.
We will use this concept to create a list of odd numbers using the for
loop. In the below example, we’ll define a function ODD_NUMBERS
that will take a num
and use it as a range to get the odd numbers from that range.
Code:
# python
def ODD_NUMBERS(num):
ODD = []
for i in range(num):
if i % 2 == 1:
ODD.append(i)
return ODD
num = 101
print("ODD Number: ", ODD_NUMBERS(num))
Output:
Use a while
Loop to Get Odd Numbers in Python
We will use While Loop
to create a list of odd numbers. First, we define a function ODD_NUMBERS
that will take the max
value of the range, and we define an array ODD
that stores all the odd numbers.
As shown below, we create another variable called number
that will increment after every while
loop.
Code:
# python
def ODD_NUMBERS(max):
ODD = []
number = 1
while number <= max:
if number % 2 != 0:
ODD.append(number)
number = number + 1
print("ODD Number: ", ODD)
max = 10
ODD_NUMBERS(max)
Output:
Using List Comprehension to Get Odd Numbers in Python
We can use an easy and compact syntax to make a list from a string or another list. List comprehension is another way of creating a new list by performing a certain function on all the elements of an existing list.
Using list comprehension is a lot faster than the for
loop. We will use the same concept to create a list of odd numbers.
Code:
# python
odd_list = [x for x in range(100) if x % 2 != 0]
print("ODD_NUMBERS :", odd_list)
Output:
We only wrote one line of code to create a list of odd numbers inside a range of 100 using list comprehension.
Use Lambda Expression to Get Odd Numbers in Python in Python
In Python, we can use the lambda function
to get the odd numbers from the given range. The lambda function
is a single line function with no name and can take any number of arguments, but it only consists of a single-line expression.
Let’s use the same concept but using a list of whole numbers. Identify the odd numbers, and save them in a new list.
Code:
# python
list1 = [20, 23, 48, 85, 96, 33, 51]
odd_number = list(filter(lambda x: (x % 2 != 0), list1))
print("Odd numbers in the list: ", odd_number)
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn