How to Convert Letter to Number in Python
-
Method 1: Using the
ord()
Function - Method 2: Converting a String of Letters to Numbers
- Method 3: Creating a Custom Mapping
- Conclusion
- FAQ
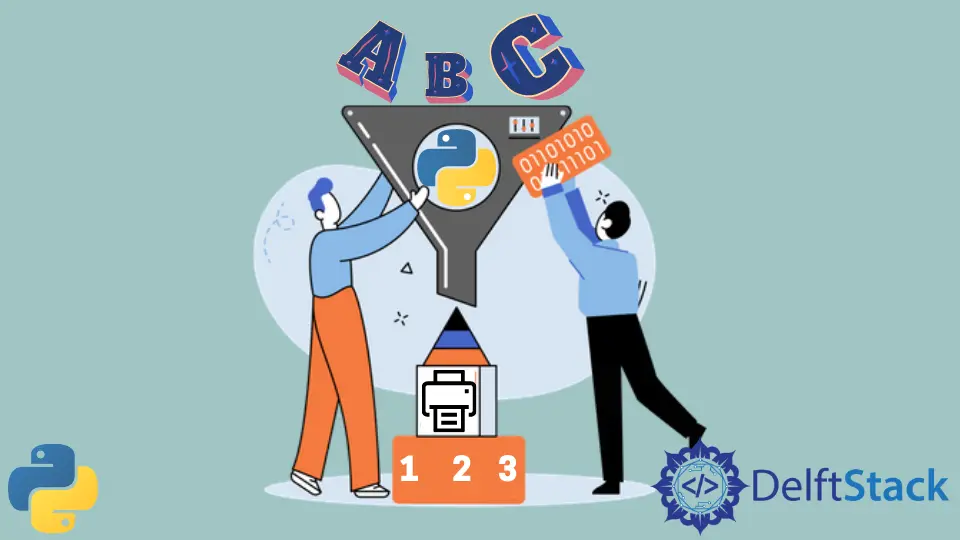
In the world of programming, converting letters to numbers is a common task that can be useful for various applications, such as encoding, decoding, or simply manipulating data. Python, with its rich set of libraries and straightforward syntax, makes this process quite simple. Whether you’re looking to convert a single character or an entire string, Python has you covered.
In this tutorial, we will explore different methods to convert letters to their corresponding numerical values in Python, including the use of the built-in ord()
function and some creative solutions. Let’s dive in and discover how to make these conversions seamlessly!
Method 1: Using the ord()
Function
One of the simplest ways to convert a letter to a number in Python is by using the built-in ord()
function. This function returns the Unicode code point for a given character, effectively converting letters into their numerical representations. For example, the letter ‘A’ corresponds to the number 65, while ‘a’ corresponds to 97.
Here’s how to use the ord()
function:
letter = 'A'
number = ord(letter)
print(number)
Output:
65
In this example, we start by defining a variable letter
and assigning it the value ‘A’. The ord()
function is then called with letter
as its argument. The result is stored in the variable number
, which is then printed to the console. This method is straightforward and efficient for converting individual characters.
If you want to convert lowercase letters, the same approach applies. For example, using ‘a’ will yield 97. This method can be extended to handle strings as well, allowing you to convert each character in a string to its corresponding number.
Method 2: Converting a String of Letters to Numbers
If you need to convert an entire string of letters into their respective numerical values, you can use a simple loop or a list comprehension. This method allows you to process each character in the string and convert it to its numerical representation using the ord()
function.
Here’s an example of how to do this:
input_string = "Hello"
numbers = [ord(char) for char in input_string]
print(numbers)
Output:
[72, 101, 108, 108, 111]
In this code, we define a string input_string
containing the word “Hello”. We then use a list comprehension to iterate over each character in the string, applying the ord()
function to convert each character to its numerical value. The result is stored in the list numbers
, which is then printed.
This method is not only concise but also highly readable. It efficiently transforms each character into its corresponding number, allowing for easy manipulation of the resulting list. You can use this approach for any string, regardless of its length or content.
Method 3: Creating a Custom Mapping
For more control over the conversion process, you might want to create a custom mapping of letters to numbers. This could be particularly useful if you have specific requirements, such as mapping ‘A’ to 1 instead of 65.
Here’s how you can implement a custom mapping:
def custom_mapping(letter):
return ord(letter.lower()) - 96
input_string = "abc"
numbers = [custom_mapping(char) for char in input_string]
print(numbers)
Output:
[1, 2, 3]
In this example, we define a function custom_mapping
that takes a letter as input and returns its corresponding number based on a custom mapping. Specifically, it converts ‘a’ to 1, ‘b’ to 2, and so on. The ord()
function is used to get the Unicode value, and we subtract 96 to achieve this mapping.
We then apply this function to each character in the string “abc” using a list comprehension. The resulting list of numbers is printed, showing the custom mapping in action. This method provides flexibility and allows you to define your own conversion logic tailored to your needs.
Conclusion
Converting letters to numbers in Python is a straightforward task that can be accomplished using various methods. Whether you choose to utilize the built-in ord()
function, process entire strings with list comprehensions, or implement a custom mapping, Python offers the tools necessary to achieve your goals efficiently. By mastering these techniques, you can enhance your programming skills and apply them in various projects. Keep experimenting and exploring the possibilities that Python provides for data manipulation!
FAQ
- How does the
ord()
function work in Python?
Theord()
function takes a single character as input and returns its corresponding Unicode code point as an integer.
-
Can I convert numbers back to letters in Python?
Yes, you can use thechr()
function to convert a number back to its corresponding character. -
What if I want to convert uppercase letters to a specific range?
You can create a custom mapping function to define how you want to convert letters, as shown in the examples. -
Is it possible to convert special characters using these methods?
Yes, theord()
function can handle any character, including special characters and symbols. -
Can I convert a string with mixed case letters?
Absolutely! You can use theord()
function in combination withlower()
orupper()
to handle mixed case strings effectively.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python