How to Display a Number With Leading Zeros in Python
-
Use the
rjust()
Function to Display a Number With Leading Zeros in Python -
Use the
str.zfill()
Function to Display a Number With Leading Zeros in Python -
Use String Formatting With the Modulus
(%)
Operator to Display a Number With Leading Zeros in Python -
Use String Formatting With the
str.format()
Function to Display a Number With Leading Zeros in Python -
Use String Formatting With
f-strings
to Display a Number With Leading Zeros in Python
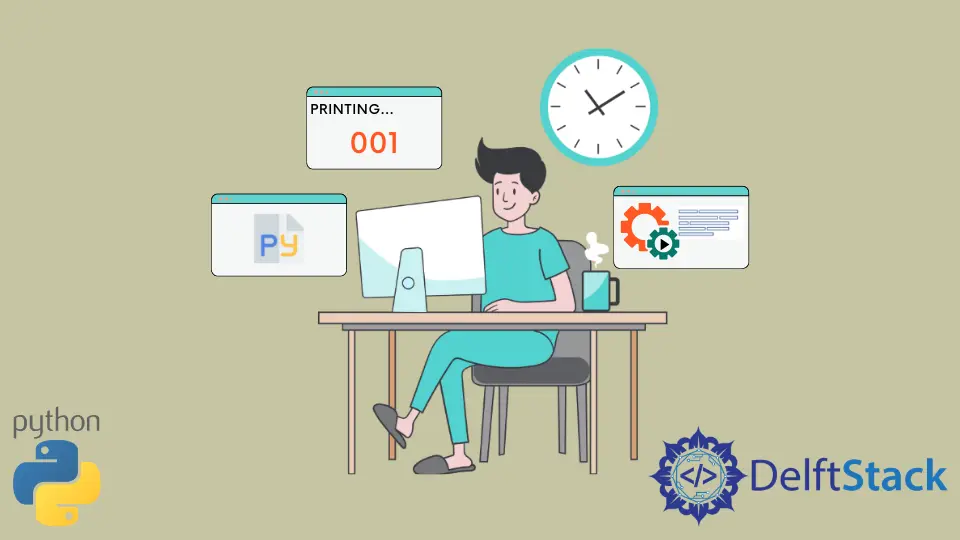
Storing and displaying numbers with leading zeros is a crucial aspect as it significantly helps in accurately converting a number from one number system to another.
This tutorial discusses the different ways you can execute to display a number with leading zeros in Python.
Use the rjust()
Function to Display a Number With Leading Zeros in Python
The rjust()
function adds padding to the left side of a string. Padding, in simple terms, is the injection of non-informative characters to the left or the right side of a given string, which adds no additional value to the initial string. We can use string padding to display leading zeros in a Python program.
The rjust()
function contains two parameters: width
and fillchar
. Out of these two, the width
parameter is mandatory and is utilized to specify the length of the given string after the padding process is completed. On the other hand, the fillchar
parameter is optional and represents the character that we want to pad the string with.
Here, we have an example program where we need to display the numbers, 1
and 10
, with leading zeros in each of the explained methods of this tutorial. The amount of padding required can be manually specified with the rjust()
function.
The following code uses the rjust()
function to display a number with leading zeros in Python.
a = [1, 10]
for num in a:
print(str(num).rjust(2, "0"))
The code above provides the following output.
01
10
Use the str.zfill()
Function to Display a Number With Leading Zeros in Python
The str.zfill(width)
function is utilized to return the numeric string; its zeros are automatically filled at the left side of the given width
, which is the sole attribute that the function takes. If the value specified of this width
parameter is less than the length of the string, the filling process does not occur.
The following code uses the str.zfill()
function to display a number with leading zeros in Python.
print(str(1).zfill(2))
print(str(10).zfill(2))
The code above provides the following output.
01
10
The str.zfill()
function is exclusively designed to pad a string with zeros towards its left side. It is an excellent choice and a highly recommended method in this case.
Use String Formatting With the Modulus (%)
Operator to Display a Number With Leading Zeros in Python
The modulus %
sign, sometimes even referred to as the string formatting or interpolation operator, is one of the few ways to implement string formatting in Python. Being the oldest of the ways you can implement string formatting, it works without any problems on almost all the versions of Python that are currently available for use on the internet.
The %
sign and a letter representing the conversion type are marked as a placeholder for the variable.
The following code uses the string formatting with the modulus (%)
operator to display a number with leading zeros in Python.
print("%02d" % (1,))
print("%02d" % (10,))
The code above provides the following output.
01
10
In the code above, the number 02
written after the %
sign sets the text width to be displayed and adjusted for the leading zeros, and the d
variable denotes the conversion type.
This method makes it easy to conduct the operation for displaying a number with leading zeros. Still, it affects the readability of the code and sometimes makes it more difficult to understand.
Use String Formatting With the str.format()
Function to Display a Number With Leading Zeros in Python
Yet another way to implement string formatting in Python is by using the str.format()
function. It utilizes the curly {}
brackets to mark the place where the variables need to be substituted in the print
statement.
The str.format()
function was introduced in Python 2.6 and can be used in all Python versions released after that up to Python 3.5. This function is incredibly popular among coders, and the programmers recommend this way to implement string formatting due to its very efficient handling in formatting complex strings.
The following code uses string formatting with the str.format()
function to display a number with leading zeros in Python.
print("{:02d}".format(1))
print("{:02d}".format(10))
The code above provides the following output.
01
10
In the program above, the content after the colon sign specifies the width and data type conversion; the curly brackets {}
act as a placeholder.
Use String Formatting With f-strings
to Display a Number With Leading Zeros in Python
Being introduced with Python 3.6, it is relatively the newest method in Python to implement string formatting. Additionally, it can be used in the latest versions of Python.
It is more efficient for implementing string formatting than the other two previous methods, the %
operator and the str.format()
function, as this one is quicker and simpler to understand. It also helps in implementing string formatting in Python at a faster rate than the other two.
The following code uses string formatting with f-strings
to display a number with leading zeros in Python.
print(f"{1:02d}")
print(f"{10:02d}")
The code above provides the following output:
01
10
In the program above, the string width and data conversion type are specified after the colon sign within the placeholder.
When it comes to using string formatting to pad the string with leading zeros, the use of f-strings
is recommended by professionals more than its other two methods. It is more concise, less prone to errors, and certainly improves the readability of the program.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python