How to Check if a Character Is a Number in Python
-
Use the
if-else
Statement to Check if a Given Character Is a Number in Python - Use ASCII Values to Check if a Given Character Is a Number in Python
-
Use the
isdigit()
Method to Check if a Given Character Is a Number in Python -
Use the
isnumeric()
Function to Check if a Given Character Is a Number in Python
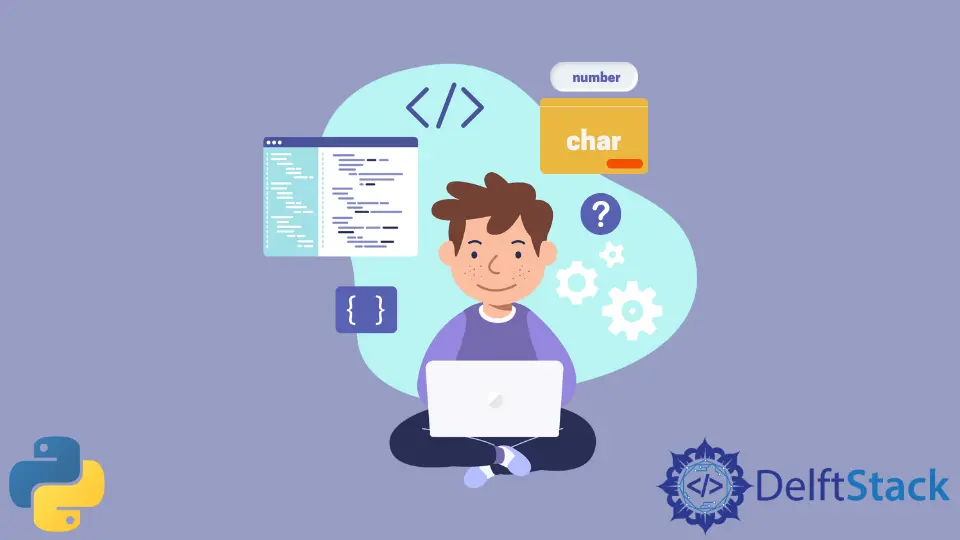
In Python, a string is capable of storing numeric values within double quotes provided that the characters are digits between (0-9).
This tutorial demonstrates methods how to check if a given character is a number in Python.
Use the if-else
Statement to Check if a Given Character Is a Number in Python
You can simply use the if-else
conditional statement in this case to check whether the given character is a number or not. The following code uses the if-else
statement to check if a given character is a number in Python.
x = input("Enter The character that you want to check for int:")
if x >= "0" and x <= "9":
print("It is a Number")
else:
print("It is Not a Number")
Output:
Enter The character that you want to check for int:6
It is a Number
Use ASCII Values to Check if a Given Character Is a Number in Python
ASCII is an abbreviation for American Standard Code for Information Interchange. It can be defined as a standard that can assign numbers, letters, and some other characters in an 8-bit code that contains a maximum of 256 available slots.
Every character, whether it is a digit (0-9) or a letter (a-z) or (A-Z), has a unique ASCII value; this can be used to figure out if a given character is a number.
We also need to use the if-else
conditional statement and the knowledge of ASCII values in this method.
The following code uses ASCII values to check if a given character is a number in Python.
x = input("Enter The character that you want to check for int:")
if ord(x) >= 48 and ord(x) <= 57:
print("It is a Number")
else:
print("It is Not a Number")
Output:
Enter The character that you want to check for int:7
It is a Number
Here, we use the ord()
function to return the ASCII values of the given data. The ASCII values of the digits are between 48 and 57. Hence, that is used as a comparison in the conditional statement.
Use the isdigit()
Method to Check if a Given Character Is a Number in Python
The isdigit()
function is used to check whether all the characters in a particular string are digits. It returns a True
value if all the characters are digits. Exponents are also confined in the scope of digits.
The following code uses the isdigit()
method to check if a given character is a number in Python.
x = "666"
y = x.isdigit()
print(y)
Output:
True
Use the isnumeric()
Function to Check if a Given Character Is a Number in Python
The isnumeric()
function works in a similar manner as the isdigit()
function and provides a True
value if all the characters in a given string are numbers.
Negative numbers like -4
and decimals with the dot .
sign are not considered numeric values in the isnumeric()
function. The following code uses the isnumeric()
function to check if a given character is a number in Python.
x = "666"
y = x.isnumeric()
print(y)
Output:
True
Both the isdigit()
and the isnumeric()
functions have the same working process and provide the same output. The only difference between the two is that the isdigit()
function returns the True
value only for the digits (0-9), while the isnumeric()
function returns True
if it contains any numeric characters; it could be another language that is used instead of the original digits 0-9
.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python