How to Round A Number to Significant Digits in Python
-
Use the
round()
Function to Round a Number to the Given Significant Digit in Python -
Use the
math
Module to Round a Number to the Given Significant Digit in Python - Use String Formatting to Round a Number to the Given Significant Digit in Python
-
Use the
sigfig
Module to Round a Number to the Given Significant Digit in Python -
Use the
decimal
Module to Round a Number to the Given Significant Digit in Python - Use NumPy to Round a Number to the Given Significant Digit in Python
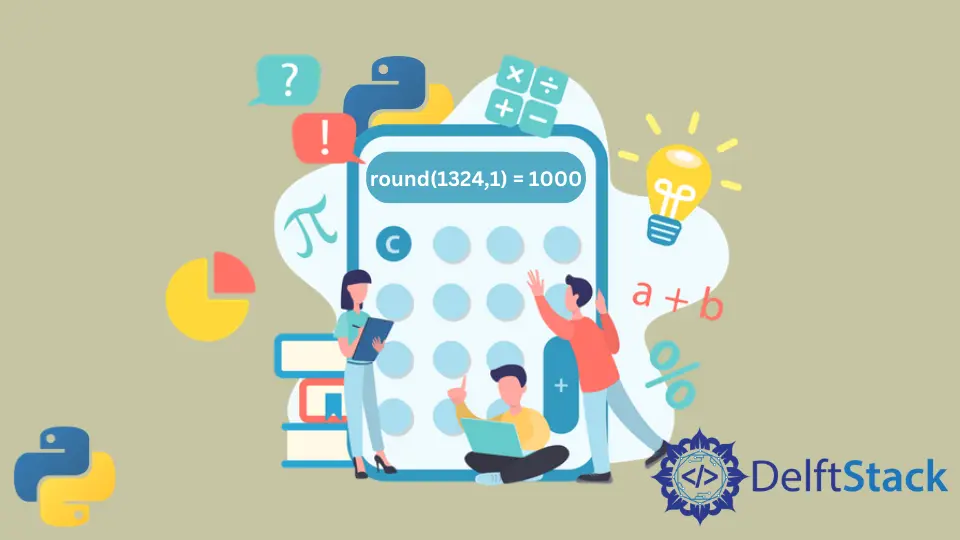
Rounding the number means replacing a number with an approximate value that is close to it. We can also round numbers to the required significant figure.
In this article, we will round a number to a specific significant digit in Python.
Use the round()
Function to Round a Number to the Given Significant Digit in Python
The round()
function rounds the given floating values to the nearest integer or specified decimal position.
We can use a negative number to round the integer to the significant floor value by assigning it to the round()
function. This negative number to be specified depends on the required significant digits.
For example, if we wish to round to one significant digit, we specify the value as (1-total digits) in the round()
function.
See the following code.
x = round(1342, -3)
print(x)
Output:
1000
Use the math
Module to Round a Number to the Given Significant Digit in Python
This method is an improvement on the previously discussed function. We can create a simple user-defined function to make this more convenient. We won’t have to specify the negative number in this method’s round()
function. We will replace this using a combination of the math.floor()
function and the math.log10()
function.
See the code below.
from math import log10, floor
def round_it(x, sig):
return round(x, sig - int(floor(log10(abs(x)))) - 1)
print(round_it(1324, 1))
Output:
1000
Use String Formatting to Round a Number to the Given Significant Digit in Python
In Python, the %g
specifier in string formats a float rounded to a specified significant figure. If the magnitude of the final number is huge, then it shows it in scientific notation.
This method returns a string. So, we have to convert the string number back to a float or integer value after formatting it.
For example,
x = float("%.1g" % 1324)
print(x)
Output:
1000.0
Use the sigfig
Module to Round a Number to the Given Significant Digit in Python
The sigfig
module is the best fit for rounding the numbers to a significant digit as it minimizes the hassle of creating a user-defined function and simplifies the code.
We can specify the number of significant digits in this module’s round()
function.
See the following example.
from sigfig import round
print(round(1324, sigfigs=1))
Output:
1000
Use the decimal
Module to Round a Number to the Given Significant Digit in Python
The decimal
module introduces a robust set of tools for high-precision decimal arithmetic and intricate rounding options. To round a number to a specific significant digit, we can leverage the scaleb()
method from the decimal
module.
Example Codes
from decimal import Decimal, ROUND_HALF_UP
import math
def round_to_significant_digit(number, digits):
scaled_number = Decimal(str(number)).scaleb(
-int(math.floor(math.log10(abs(number)))) + (digits - 1)
)
rounded_number = scaled_number.quantize(Decimal("1"), rounding=ROUND_HALF_UP)
return float(rounded_number)
rounded_number = round_to_significant_digit(1324, 1)
print(rounded_number)
Output:
1000.0
In the provided example:
Decimal(str(number))
converts the inputnumber
to aDecimal
object, ensuring precision.scaleb()
method is used to scale the number to the desired significant digit. The scaling factor is calculated using the formula-int(math.floor(math.log10(abs(number)))) + (digits - 1)
.quantize()
method is employed to round the scaled number to the nearest integer while adhering to rounding rules specified byROUND_HALF_UP
.
Use NumPy to Round a Number to the Given Significant Digit in Python
NumPy, a powerful numerical computing library, offers a versatile function for rounding numbers to a specified number of significant digits. The numpy.around()
function serves as a valuable tool for this purpose.
Example
import numpy as np
def round_to_significant_digit(number, digits):
rounded_number = np.around(
number, digits - int(np.floor(np.log10(abs(number)))) - 1
)
return rounded_number
rounded_number = round_to_significant_digit(1324, 1)
print(rounded_number)
Output:
1000.0
In the provided example:
np.around()
function is used to round thenumber
to the specified significant digit. The rounding precision is calculated usingdigits - int(np.floor(np.log10(abs(number)))) - 1
.