Scientific Notation in Python
- Using the Built-in Format Function
- Using f-Strings for Formatting
- Using the NumPy Library for Scientific Notation
- Conclusion
- FAQ
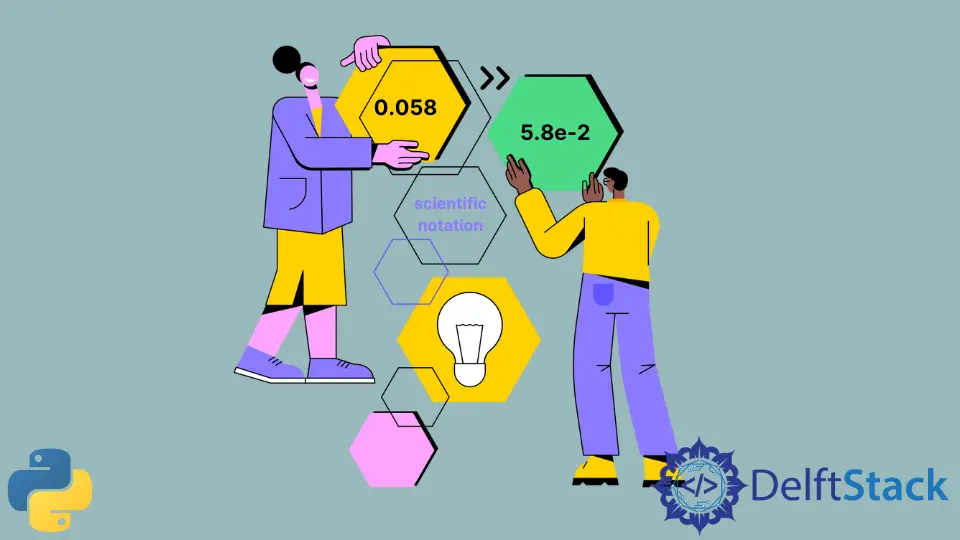
Understanding how to display numbers in scientific notation is essential for data analysis, scientific computing, and more. Python provides several methods to format numbers efficiently, making it easier to work with large or small values. Whether you’re a beginner or looking to refine your skills, this tutorial will guide you through various techniques for displaying numbers in scientific notation using Python. We’ll explore built-in functions, string formatting, and the NumPy library to help you master this important aspect of Python programming. By the end of this article, you’ll be able to present numbers in a clear and concise manner, enhancing the readability of your data.
Using the Built-in Format Function
Python’s built-in format()
function provides a straightforward way to display numbers in scientific notation. This method is beneficial for quick formatting without requiring additional libraries. You can specify the format using a string that includes the letter ’e’ or ‘E’ to indicate scientific notation.
Here’s a simple example:
number = 123456789
formatted_number = format(number, ".2e")
print(formatted_number)
Output:
1.23e+08
In this example, we first define a large integer, 123456789
. By using the format()
function, we specify that we want to format this number in scientific notation with two decimal places. The output shows the number as 1.23e+08
, which is a compact way to represent large numbers. The ’e’ indicates that the number is in scientific notation, with the exponent showing how many places to move the decimal point.
This method is particularly useful when presenting data in reports or visualizations, where space is limited, and clarity is paramount. You can adjust the number of decimal places by changing the precision in the format string. This flexibility allows you to tailor the output to your audience’s needs, making it an essential tool for any Python programmer.
Using f-Strings for Formatting
Starting from Python 3.6, f-strings offer a modern and efficient way to format strings, including numbers in scientific notation. This method is not only concise but also enhances code readability. You can easily embed expressions inside string literals, which allows for dynamic formatting.
Here’s how you can use f-strings to display a number in scientific notation:
number = 0.000123456
formatted_number = f"{number:.2e}"
print(formatted_number)
Output:
1.23e-04
In this snippet, we define a small floating-point number, 0.000123456
. By utilizing an f-string, we format this number to two decimal places in scientific notation. The output is 1.23e-04
, which clearly represents the small value. The :.2e
inside the curly braces specifies that we want two decimal places in scientific notation.
F-strings are not only efficient but also make your code cleaner and easier to understand at a glance. This method is particularly helpful when you’re working with variables that may change, allowing you to format them on the fly without cumbersome syntax. As a result, f-strings have become a preferred choice for many Python developers looking to streamline their code.
Using the NumPy Library for Scientific Notation
When dealing with large datasets or complex mathematical computations, the NumPy library becomes a powerful ally. NumPy offers a dedicated function for formatting numbers in scientific notation, making it especially useful for scientists and engineers.
Here’s how you can use NumPy to display numbers in scientific notation:
import numpy as np
number = np.array([123456789, 0.000123456])
formatted_numbers = np.format_float_scientific(number, precision=2)
print(formatted_numbers)
Output:
['1.23e+08' '1.23e-04']
In this example, we first import the NumPy library and create a NumPy array containing two numbers: a large integer and a small floating-point number. We then use the np.format_float_scientific()
function to format these numbers in scientific notation with a precision of two decimal places. The output shows both numbers formatted as strings in scientific notation.
Using NumPy for this purpose is particularly advantageous when working with large datasets, as it can handle arrays efficiently. This method also allows you to set the precision globally, making it easy to maintain consistency across your outputs. For anyone involved in scientific computing or data analysis, mastering NumPy’s formatting capabilities can significantly enhance your data presentation and interpretation.
Conclusion
In this tutorial, we’ve explored various methods for displaying numbers in scientific notation in Python. From using the built-in format()
function and f-strings to leveraging the power of the NumPy library, each method has its unique advantages. Understanding these techniques will not only improve your coding skills but also enhance the clarity and effectiveness of your data presentations. Whether you’re working on a small project or a large-scale data analysis, knowing how to format numbers in scientific notation is an invaluable skill that will serve you well in your programming journey.
FAQ
-
What is scientific notation?
Scientific notation is a way of expressing numbers that are too large or too small in a compact form, using powers of ten. -
How do I format a number in scientific notation in Python?
You can use the built-informat()
function, f-strings, or the NumPy library to format numbers in scientific notation. -
What is the difference between ’e’ and ‘E’ in scientific notation?
’e’ and ‘E’ are interchangeable in scientific notation; both represent the exponent part of the number.
-
Can I control the number of decimal places in scientific notation?
Yes, you can specify the precision by including it in the format string, such as:.2e
for two decimal places. -
Is NumPy necessary for formatting numbers in scientific notation?
No, NumPy is not necessary, but it is useful for handling arrays and large datasets efficiently.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn