How to Convert List of Strings to Integer in Python
- Method 1: Using List Comprehension
- Method 2: Using the map() Function
- Method 3: Using a Traditional For Loop
- Method 4: Using NumPy for Large Datasets
- Conclusion
- FAQ
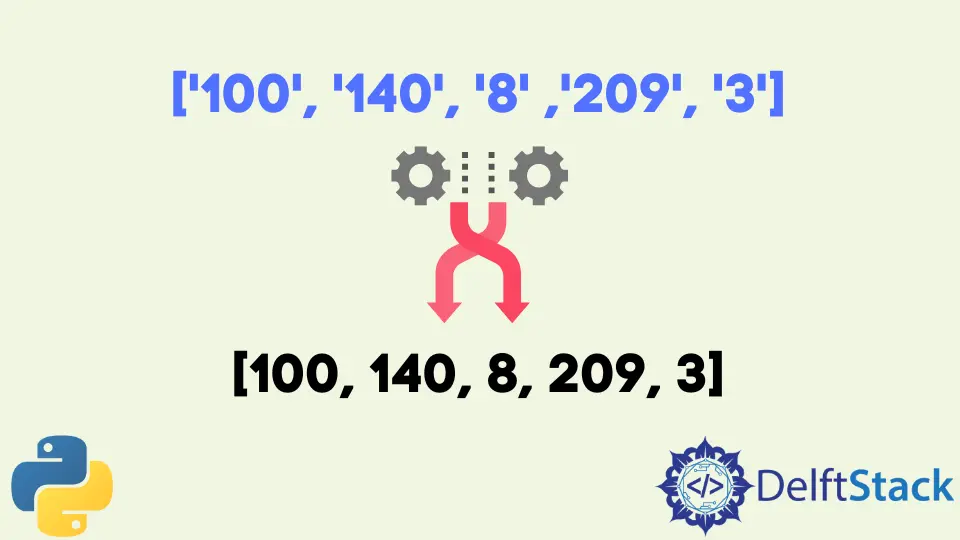
Converting a list of strings to integers is a common task in Python programming, especially when dealing with data input from users or files. Whether you’re processing numerical data from a CSV file or simply taking user input, transforming these strings into integers allows for more efficient calculations and operations.
In this tutorial, we will explore various methods to convert a list of strings to integers in Python. Each method will be explained with clear examples, making it easy for you to follow along. By the end of this article, you will have a solid understanding of how to perform this conversion effectively.
Method 1: Using List Comprehension
One of the most Pythonic ways to convert a list of strings to integers is by using list comprehension. This method is not only concise but also highly readable. Here’s how you can do it:
string_list = ["1", "2", "3", "4", "5"]
int_list = [int(num) for num in string_list]
print(int_list)
Output:
[1, 2, 3, 4, 5]
In this example, we start with a list of strings called string_list
. Using list comprehension, we iterate over each string in the list and apply the int()
function to convert it into an integer. The result is a new list called int_list
, which contains the converted integers. This method is efficient and leverages Python’s powerful list comprehension feature, making it a favorite among developers for its simplicity and elegance.
Method 2: Using the map() Function
Another effective way to convert a list of strings to integers is by using the map()
function. This built-in function applies a specified function to every item of the iterable (in this case, our list of strings). Here’s how it works:
string_list = ["10", "20", "30", "40", "50"]
int_list = list(map(int, string_list))
print(int_list)
Output:
[10, 20, 30, 40, 50]
In this example, the map()
function takes two arguments: the first is the conversion function int
, and the second is the iterable string_list
. It applies the int()
function to each element of the list. The result of map()
is a map object, which we convert to a list using the list()
constructor. This method is particularly useful when you want to apply a function to all elements in an iterable without explicitly writing a loop.
Method 3: Using a Traditional For Loop
While list comprehension and map()
are often preferred for their brevity, using a traditional for loop can sometimes be clearer, especially for beginners. Here’s how to do it using a for loop:
string_list = ["100", "200", "300", "400", "500"]
int_list = []
for num in string_list:
int_list.append(int(num))
print(int_list)
Output:
[100, 200, 300, 400, 500]
In this approach, we initialize an empty list called int_list
. We then iterate over each string in string_list
, convert it to an integer using int()
, and append the result to int_list
. This method is straightforward and easy to understand, making it a great choice for those who are just starting with Python programming. It also allows for more complex operations if needed, giving you the flexibility to customize the conversion process.
Method 4: Using NumPy for Large Datasets
If you are working with large datasets, using the NumPy library can enhance performance significantly. NumPy is optimized for numerical computations and can handle large arrays efficiently. Here’s an example of how to convert a list of strings to integers using NumPy:
import numpy as np
string_list = ["1000", "2000", "3000", "4000", "5000"]
int_array = np.array(string_list, dtype=int)
print(int_array)
Output:
[1000 2000 3000 4000 5000]
In this example, we first import the NumPy library. We then create a NumPy array from string_list
and specify the data type as int
. This approach is particularly beneficial when dealing with large datasets, as NumPy arrays consume less memory and provide faster computations compared to standard Python lists. If you’re working on data analysis or scientific computing projects, leveraging NumPy can greatly improve your code’s performance and efficiency.
Conclusion
Converting a list of strings to integers in Python can be accomplished through various methods, each with its own advantages. Whether you prefer the elegance of list comprehension, the simplicity of map()
, the clarity of a for loop, or the efficiency of NumPy, the choice ultimately depends on your specific needs and preferences. By mastering these techniques, you can handle numerical data more effectively in your Python programs. So go ahead, try these methods in your next project, and see which one works best for you!
FAQ
-
What is the best method to convert a list of strings to integers in Python?
The best method depends on your needs. List comprehension is concise, while a for loop is more explicit. For large datasets, consider using NumPy. -
Can I convert strings with decimal points to integers?
Yes, but you must first convert them to floats and then to integers. Useint(float(num))
for each string. -
What happens if the list contains non-numeric strings?
If you try to convert non-numeric strings to integers, Python will raise a ValueError. You may want to handle exceptions or filter the list first. -
Is it possible to convert a mixed list of strings and integers?
Yes, you can use a combination ofisinstance()
to check types and convert accordingly. -
How can I handle errors during conversion?
You can use a try-except block to catch ValueErrors during conversion and handle them gracefully.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python