How to Rotate Image in OpenCV
-
Use the
rotate()
Function of OpenCV to Rotate an Image in Python -
Use the
warpAffine()
Function of OpenCV to Rotate an Image in Python
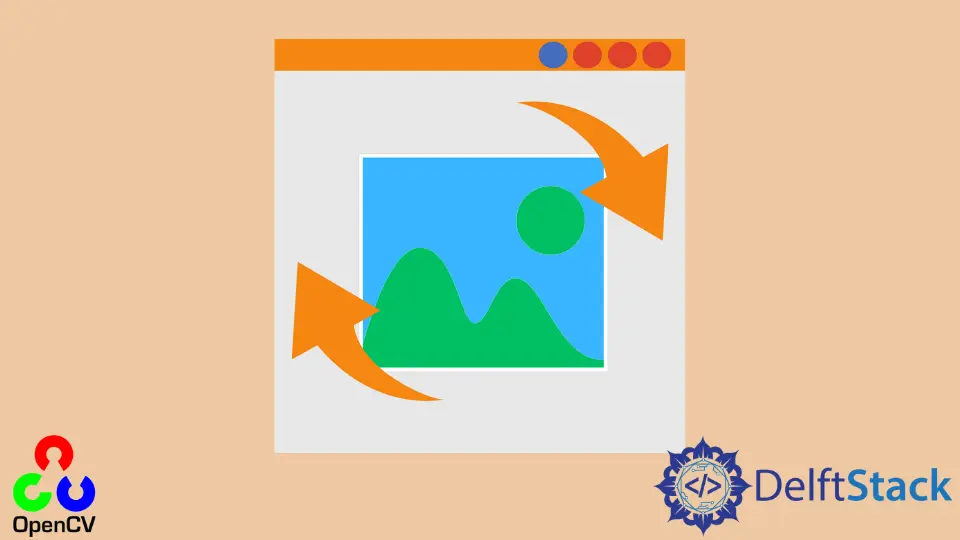
This tutorial will discuss rotating an image using the rotate()
and warpAffine()
function of OpenCV in Python.
Use the rotate()
Function of OpenCV to Rotate an Image in Python
We can use the rotate()
function of OpenCV to rotate an image. The first argument of the rotate()
function is the image we want to rotate. The second argument specifies how much the image will rotate and which direction.
There are only three ways to rotate an image using the rotate()
function. We can set the second argument to cv2.ROTATE_90_CLOKWISE
to rotate the image to 90 degrees clockwise.
We can use the cv2.ROTATE_180
to rotate the image 180 degrees or flip it. We can use the cv2.ROTATE_90_COUNTERCLOCKWISE
to rotate the image to 90 degrees counterclockwise or 270 degrees clockwise. These are the only three angles to rotate an image using the rotate()
function.
For example, let’s read an image using the imread()
function, rotate it to 90 degrees clockwise, and then show it along with the original image using the imshow()
function. See the code below.
import cv2
import numpy as np
image = cv2.imread("cat.jpg")
image_norm = cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE)
cv2.imshow("original Image", image)
cv2.imshow("Rotated Image", image_norm)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
Using rotate()
, we can only rotate an image on three-angle, but if we want to rotate an image on every angle, we can use the warpAffine()
function, discussed below.
Use the warpAffine()
Function of OpenCV to Rotate an Image in Python
We can use the warpAffine()
function of OpenCV to rotate an image at any angle. The warpAffine()
function transforms a matrix for another matrix.
To rotate an image, we have to find its rotation matrix using the getRotationMatrix2D()
function of OpenCV.
The first argument of the getRotationMatrix2D()
is the center of the image along which we want to rotate it. The second argument is the angle of rotation, and the third argument is the scale of the image.
If the scale is less than one, the image will become smaller in size than the original image, or in other words, it will be zoomed out. The image will be scaled or zoomed in if the scale is a positive number. If the scale is 1, the image will not be scaled.
The first argument of the warpAffine()
function is the image that we want to rotate. The second argument is the rotation matrix, and the third argument is the size of the output image.
For example, let’s rotate the above image to 45 degrees around its center. See the code below.
import cv2
import numpy as np
image = cv2.imread("cat.jpg")
(h, w) = image.shape[:2]
center = (w / 2, h / 2)
angle = 30
scale = 1
M = cv2.getRotationMatrix2D(center, angle, scale)
rotated = cv2.warpAffine(image, M, (w, h))
cv2.imshow("original Image", image)
cv2.imshow("Rotated Image", rotated)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
In the code above, we used the shape method to find the length and width of the given image, and then we calculated the center of the image by taking half of the length and width. We can change the center, angle, and scale value to get the desired result.
We can also set the border mode of the output image using the borderMode
argument. By default, the border mode is set to cv2.BORDER_CONSTANT
, and as you can see, there is no border at the corners of the output image.
We can set the border mode to cv2.BORDER_TRANSPARENT
. The output image will have the same borders as the original image, or the corners will remain the same.
We can set the border mode to cv2.BORDER_REPLICATE
. The output image border or corners will be drawn using the nearby pixels.
Click this link for more details about the border modes.
Related Article - Python OpenCV
- How to Fix No Module Named CV2 in Mac in Python
- Image Masking in OpenCV
- How to Utilize Bitwise_AND on an Image Using OpenCV
- OpenCV Package Configuration
- OpenCV ArUco Markers
- How to SIFT Using OpenCV in Python