How to Fix Float Object Is Not Callable in Python
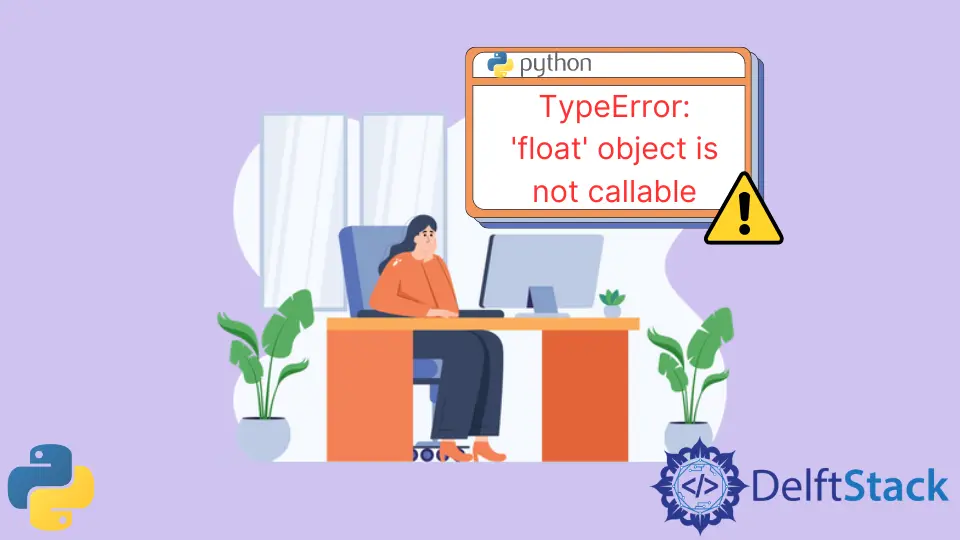
Functions can be thought of as reusable code that can be called and used anywhere in the program. We can only call functions in Python.
To call functions, we use the parentheses with the function name. Any arguments or parameters to be provided for the function are specified within these parentheses.
the float object is not callable
Error in Python and How to Solve It
This tutorial will discuss Python’s float object is not callable
error.
This is a TypeError
, which indicates that some invalid operation is associated with a given object. In Python, we can only call functions. This error indicates that a float
object is being called.
For example,
a = 1.5
a()
Output:
TypeError: 'float' object is not callable
In the above example, we get the error because we created a float
variable a
and tried to call it. We will now discuss various scenarios where such an error may occur.
In Python, we sometimes perform complex complications and may use parentheses to separate operators and operands. Sometimes, one may pace the parentheses in the wrong position where it may seem to represent a function call statement.
For example,
a = 1.5
b = 5
c = 8 * 10 / 5 * a(2) * 5
print(c)
Output:
TypeError: 'float' object is not callable
We need to be careful of the parentheses and place the operands accordingly to fix this. It is a simple fix for the previous example, as shown below.
a = 1.5
b = 5
c = 8 * 10 / 5 * (a * 2) * 5
print(c)
Output:
240.0
Let us now discuss another situation. See the code below.
def mul(a, b):
return a * b
mul = mul(7, 4.2)
print(mul)
mul = mul(13, 8.2)
print(mul)
Output:
29.400000000000002
TypeError: 'float' object is not callable
In the above example, we created a function and then assigned it to the same name variable twice.
This works for the first call but returns the float object is not callable
is caused due to the second function call. This happens because the function gets overridden with the variable name in the second function call statement.
It also has a simple fix. We should change the function’s name or the variable to solve this error.
See the code below.
def mul_cal(a, b):
return a * b
mul = mul_cal(7, 4.2)
print(mul)
mul = mul_cal(13, 8.2)
print(mul)
Output:
29.400000000000002
106.6
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Float
- How to Find Maximum Float Value in Python
- How to Convert a String to a Float Value in Python
- How to Check if a String Is a Number in Python
- How to Convert String to Decimal in Python
- How to Convert List to Float in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python