How to Convert a String to a Float Value in Python
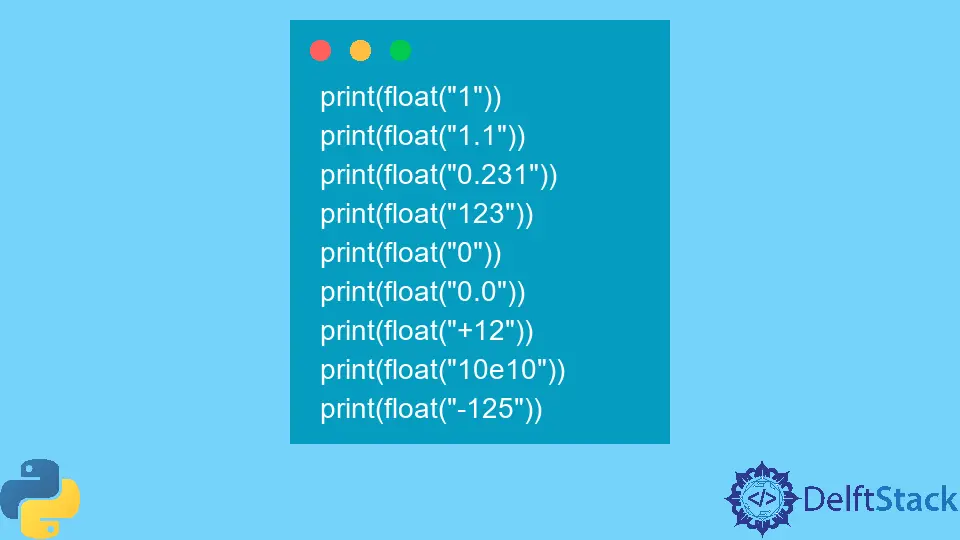
In programming, data is stored in variables, and these variables have certain data types. These data types include integer, floating value, string, and boolean values.
We sometimes run into a situation wherein we have to convert values of some data type to another data type. For example, converting integer
to float
, integer
to long
, integer
to boolean
, string
to boolean
, etc.
In this article, we will learn how to convert a string value to a floating value.
Converting a String to a Float Value in Python
When converting a string to a float value, we must ensure that the string represents a number. For example, "1"
and "1.0"
can be converted to 1.0
, but we can not convert "hello"
and "python is amazing"
to a floating value.
Let us look into how to perform the conversion practically. Refer to the following Python code for this.
print(float("1"))
print(float("1.1"))
print(float("0.231"))
print(float("123"))
print(float("0"))
print(float("0.0"))
print(float("+12"))
print(float("10e10"))
print(float("-125"))
Output:
1.0
1.1
0.231
123.0
0.0
0.0
12.0
100000000000.0
-125.0
Python has a float()
function that can convert a string to a floating value. Not only a string, but we can also convert an integer to a floating value using this in-built method.
As mentioned above, we can not convert a string representing a sentence or a word to a floating value. The float()
method will throw a ValueError
exception for such a scenario.
The following Python code depicts this.
print(float("hello"))
Output:
Traceback (most recent call last):
File "<string>", line 1, in <module>
ValueError: could not convert string to float: 'hello'
If we are unsure about the string values that we pass to the float()
method, we can use try
and except
blocks to catch exceptions and continue with the program’s execution. Refer to the following code for this.
strings = ["1.1", "-123.44", "+33.0000", "hello", "python", "112e34", "0"]
for s in strings:
try:
print(float(s))
except ValueError:
print("Conversion failed!")
Output:
1.1
-123.44
33.0
Conversion failed!
Conversion failed!
1.12e+36
0.0
As we can see, the try...except
block helped us catch exceptions for "hello"
and "python"
. For other elements, the algorithm ran seamlessly.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python
Related Article - Python Float
- How to Find Maximum Float Value in Python
- How to Fix Float Object Is Not Callable in Python
- How to Check if a String Is a Number in Python
- How to Convert String to Decimal in Python
- How to Convert List to Float in Python