How to Convert List to Float in Python
-
Use the
for
Loop to Convert All Items in a List to Float in Python - Use the List Comprehension Method to Convert All Items in a List to Float in Python
-
Use the
numpy.float_()
Function to Convert Items in a List to Float in Python -
Use the
numpy.array()
Function to Convert Items in a List to Float in Python -
Use the
map()
Function to Convert Items in a List to Float in Python - Use the Generator Expression to Convert All Items in a List to Float in Python
- Conclusion
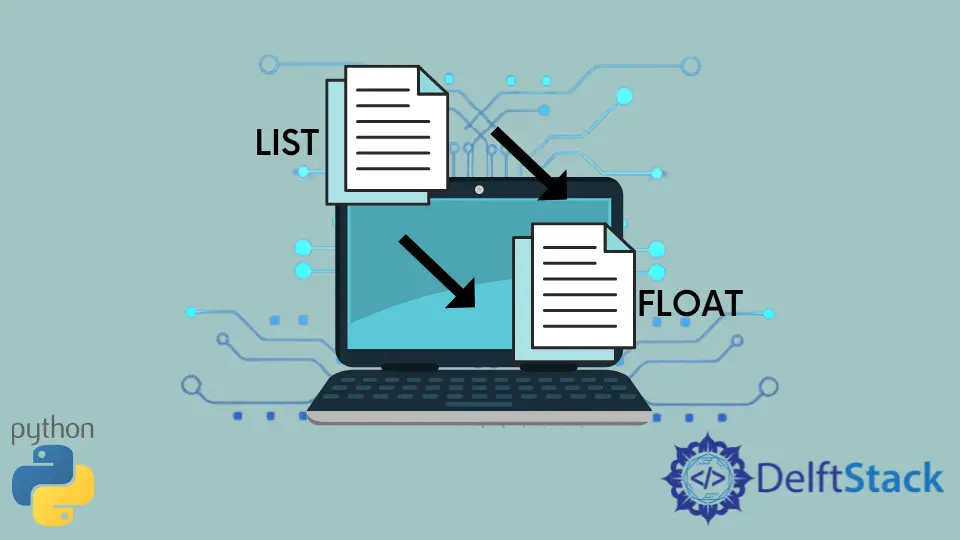
A list can store multiple elements of different data types. Due to this, we may encounter situations where we have to change the type of elements in the list.
For example, we may have a list of strings, where each string is in the form of a float value. In this tutorial, we will convert the elements of a list from a string to a float in Python.
Use the for
Loop to Convert All Items in a List to Float in Python
We can use the for
loop to iterate through the list and convert each element to float type using the float()
function. We can then add each element to a new list using the append()
function.
In the following syntax, the original_list
is the list containing the original string items. The for
loop iterates over each item
in original_list
.
Inside the loop, float(item)
is used to convert the current item
to a float, and the result is assigned to float_item
. We can perform further operations on float_item
or append it to another list if needed.
Basic Syntax:
for item in original_list:
float_item = float(item)
# Do something with float_item or append it to another list
Parameters:
item
: This is a variable that represents the current item in theoriginal_list
during each iteration of the loop.original_list
: This is the list that you are iterating through.
Code example:
lst = ["1.5", "2.0", "2.5"]
float_lst = []
for item in lst:
float_lst.append(float(item))
print(float_lst)
Output:
[1.5, 2.0, 2.5]
In this code block, we initialize a list called lst
containing string representations of floating-point numbers. We then create an empty list called float_lst
.
Using a for
loop, we iterate through each item in lst
. For each item, we convert it to a floating-point number using the float()
function and append it to the float_lst
.
Finally, we print the float_lst
, which results in the output: [1.5, 2.0, 2.5]
, representing the list of converted float values.
Use the List Comprehension Method to Convert All Items in a List to Float in Python
The list comprehension method creates a new list in a single line of code. It achieves the same result but more compactly and elegantly.
In the following syntax, the result_list
is the list that will store the converted float values. The float(item)
is the expression that converts each item
in the original_list
to a float.
Lastly, original_list
is the list containing the original string items.
Basic Syntax:
result_list = [float(item) for item in original_list]
Parameter:
original_list
: It represents the input list of string items that you want to convert to a list of floats.
Code example:
lst = ["1.2", "3.4", "5.6"]
float_lst = [float(item) for item in lst]
print(float_lst)
Output:
[1.2, 3.4, 5.6]
In this code, we begin with a list called lst
, containing string representations of floating-point numbers. We then create a new list, float_lst
, using a list comprehension.
With the list comprehension, we iterate through each item in lst
, converting them to floating-point numbers using the float()
function. Finally, we print float_lst
, which results in the output: [1.2, 3.4, 5.6]
, representing the list of the original string items converted to floats.
The output matches the input list since the original string items were already in a valid floating-point format.
Use the numpy.float_()
Function to Convert Items in a List to Float in Python
The numpy.float_()
function creates a NumPy
array with float values. We can pass the list to this function to create an array with float values and then convert this array to a list by using the list()
function.
It’s primarily used to ensure that the data types of the elements in the list are explicitly cast to floating-point numbers when working with NumPy
arrays.
Code example:
import numpy as np
lst = ["1.5", "2.0", "2.5"]
float_lst = list(np.float_(lst))
print(float_lst)
Output:
[1.5, 2.0, 2.5]
In this code, we first import the NumPy
library, denoting it as np
. We have a list, lst
, containing string representations of numbers.
To convert these strings into actual floating-point numbers, we use the np.float_()
function within a list()
conversion. The np.float_()
function ensures that each element in the list is explicitly cast to a floating-point data type.
Finally, we print the float_lst
, which results in the output [1.5, 2.0, 2.5]
, representing the list of converted floating-point values. This output matches the original list, but with data types changed to float.
It’s important to note that in many cases, using np.array()
is a more common and intuitive way to create NumPy
arrays from lists of numbers. The numpy.float_()
function is less commonly used for this purpose.
Use the numpy.array()
Function to Convert Items in a List to Float in Python
This is similar to the previous method. Instead of using the numpy.float_()
function, we will use the numpy.array()
function and specify the dtype
parameter as float
.
In the following syntax, the import numpy as np
imports the NumPy
library with the alias np
. The input_list
is the list containing the original string items.
Next, the data_type
is the specified data type (e.g., "float"
). This line, np.array(input_list, dtype=data_type)
, creates a NumPy
array from input_list
with the specified data type.
Lastly, list()
is used to convert the NumPy
array back to a regular Python list, resulting in resulting_list
containing the converted float values.
Basic Syntax:
import numpy as np
resulting_list = list(np.array(input_list, dtype=data_type))
Parameters:
input_list
: This is the parameter that represents the input list containing the original string items that you want to convert to a list of floatsdata_type
: This parameter specifies the data type you want to assign to the elements in the resulting list (e.g.,"float"
). It indicates the data type to be used when creating theNumPy
array from theinput_list
.
Code example:
import numpy as np
lst = ["1.5", "2.0", "2.5"]
float_lst = list(np.array(lst, dtype="float"))
print(float_lst)
Output:
[1.5, 2.0, 2.5]
In this code, we begin by importing the NumPy
library as np
. We have a list, lst
, containing string representations of numbers.
Using NumPy
, we create a new list, float_lst
, by first converting lst
into a NumPy
array while specifying that the desired data type is "float"
. This ensures that each element in the list is explicitly cast to a floating-point number.
Finally, we print float_lst
, which results in the output: [1.5, 2.0, 2.5]
, representing the list of the converted floating-point values. The output reflects the original list but with data types changed to float, facilitating numerical operations.
Use the map()
Function to Convert Items in a List to Float in Python
Using the map()
function in Python allows us to apply a specific function to every item in an iterable (such as a list) and get a new iterable containing the transformed items. When we want to convert items in a list to float, we can use the float()
function as the mapping function.
In the following syntax, float_lst
is the list that will store the converted float values. The map(float, original_list)
applies the float()
function to each item
in the original_list
, converting them to floating-point numbers.
Lastly, original_list
is the list containing the original string items.
Basic syntax:
float_lst = list(map(float, original_list))
Parameters:
float
: This is the function you want to apply to each item in the iterable.original_list
: This is the list containing the string representations of numbers that you want to convert to floats.
Code example:
lst = ["1.5", "2.0", "2.5"]
float_lst = list(map(float, lst))
print(float_lst)
Output:
[1.5, 2.0, 2.5]
In this code, we utilize the map()
function to convert items in the lst
list to floating-point numbers in Python. We begin with a list, lst
, which contains string representations of numbers.
The map(float, lst)
function is applied to each item in the list, converting them to their corresponding floating-point values. We then use the list()
function to convert the map
object into a regular Python list, resulting in float_lst
.
Finally, we print float_lst
, which displays the converted list of floating-point values. The output will be: [1.5, 2.0, 2.5]
, representing the list with the original string items converted to float values.
This code provides a convenient way to cast string elements to numeric types for numerical operations.
Use the Generator Expression to Convert All Items in a List to Float in Python
In the following syntax, float_lst
is the list that will store the converted float values. The float(item)
is the expression that converts each item
in the original_list
to a float.
Lastly, original_list
is the list containing the original string items.
Basic syntax:
float_lst = list(float(item) for item in original_list)
Parameters:
float_lst
: This is the list that will store the converted float values.original_list
: This parameter represents the input list containing the original string items. The list comprehension processes each item in this input list and converts them to float values before populatingfloat_lst
with the results.
Code example:
lst = ["1.5", "2.0", "2.5"]
float_lst = list(float(item) for item in lst)
print(float_lst)
Output:
[1.5, 2.0, 2.5]
In this code, we use a generator expression to convert all items in the lst
list to floating-point numbers in Python. We begin with the list lst
, which contains string representations of numbers.
The generator expression float(item) for item in lst
iterates through each item in the list, converting them to their corresponding floating-point values. We then use the list()
function to convert the generator expression into a regular Python list, resulting in float_lst
.
Finally, we print float_lst
, displaying the list with the original string items converted to float values. The output will be: [1.5, 2.0, 2.5]
, representing the list with the original string items converted to floating-point values.
This approach provides an efficient way to perform the conversion while preserving the order of the items in the list.
Conclusion
In this article, we explored multiple methods for converting items within a Python list from strings to float values. Lists can hold diverse data types, leading to scenarios where you need to change the data type of elements in the list.
We discussed six approaches for this conversion, each with its advantages. The methods include using a for
loop, list comprehension, the numpy.float_()
function, the numpy.array()
function with a specified data type, applying the map()
function, and utilizing a generator expression.
These techniques offer flexibility and efficiency, enabling us to work effectively with numerical data in Python. We can choose the method that best aligns with our specific requirements and coding style.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python