在 Python 中将列表转换为浮点数
Hemank Mehtani
2023年1月30日
-
使用
for
循环将列表中的所有项目转换为 Python 中的浮点数 - 在 Python 中使用列表推导方法将列表中的所有项目转换为浮点数
-
Python 中使用
numpy.float_()
函数将列表中的项目转换浮点数 -
在 Python 中使用
numpy.array()
函数将列表中的项目转换为浮点数
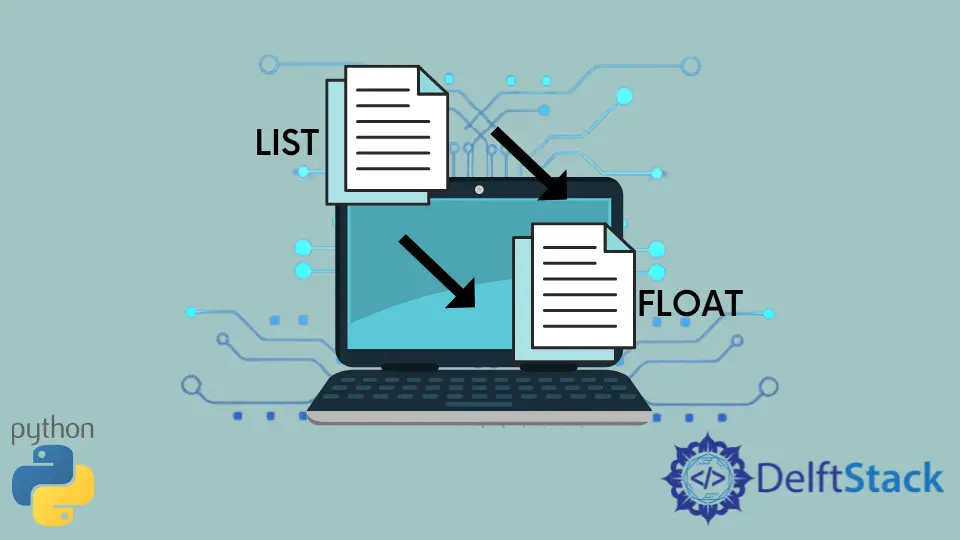
一个列表可以存储多个不同数据类型的元素。因此,我们可能会遇到必须更改列表中元素类型的情况。例如,我们可能有一个字符串列表,其中每个字符串都是浮点值的形式。
在本教程中,我们将在 Python 中将列表的元素从字符串转换为浮点数。
使用 for
循环将列表中的所有项目转换为 Python 中的浮点数
我们可以使用 for
循环遍历列表并使用 float()
函数将每个元素转换为浮点类型。
然后我们可以使用 append()
函数将每个元素添加到一个新列表中。
例如,
lst = ["1.5", "2.0", "2.5"]
float_lst = []
for item in lst:
float_lst.append(float(item))
print(float_lst)
输出:
[1.5, 2.0, 2.5]
在 Python 中使用列表推导方法将列表中的所有项目转换为浮点数
列表推导方法在一行代码中创建一个新列表。它实现了相同的结果,但更加紧凑和优雅。
例如,
lst = ["1.2", "3.4", "5.6"]
float_lst = [float(item) for item in lst]
print(float_lst)
输出:
[1.5, 2.0, 2.5]
Python 中使用 numpy.float_()
函数将列表中的项目转换浮点数
numpy.float_()
函数创建一个带有浮点数的 NumPy
数组。我们可以将列表传递给这个函数来创建一个带有浮点数的数组。然后,我们可以使用 list()
函数将此数组转换为列表。
例如,
import numpy as np
lst = ["1.5", "2.0", "2.5"]
float_lst = list(np.float_(lst))
print(float_lst)
输出:
[1.5, 2.0, 2.5]
在 Python 中使用 numpy.array()
函数将列表中的项目转换为浮点数
这与之前的方法类似。我们将使用 numpy.array()
函数并将 dtype
参数指定为 float
,而不是使用 numpy.float_()
函数。
请参考下面的代码。
import numpy as np
lst = ["1.5", "2.0", "2.5"]
float_lst = list(np.array(lst, dtype="float"))
print(float_lst)
输出:
[1.5, 2.0, 2.5]
相关文章 - Python List
- 从 Python 列表中删除某元素的所有出现
- 在 Python 中将字典转换为列表
- 在 Python 中从列表中删除重复项
- 如何在 Python 中获取一个列表的平均值
- Python 列表方法 append 和 extend 之间有什么区别
- 如何在 Python 中将列表转换为字符串