在 Python 中检查字符串是否为数字
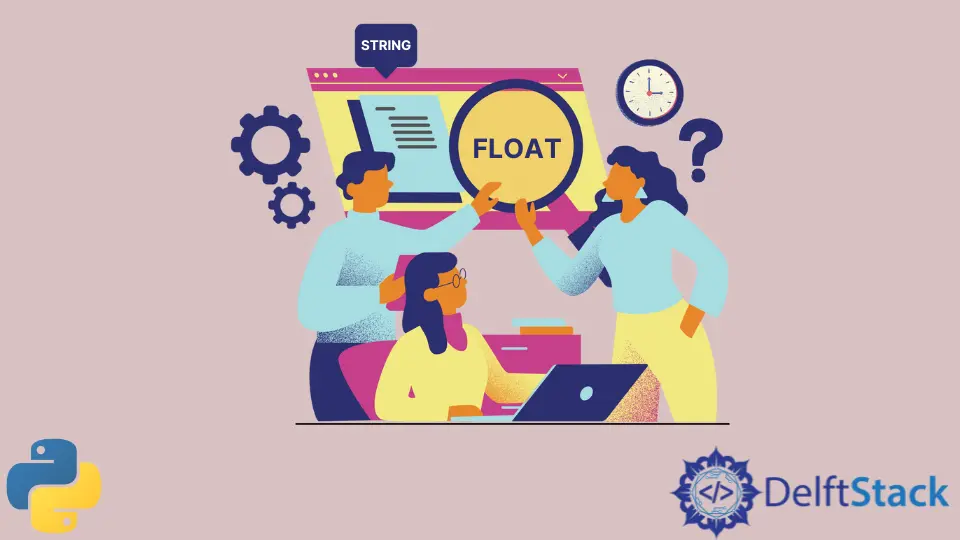
字符串是由双引号或单引号括起来的字符序列。跨越多行的字符串,也称为多行字符串,用三引号括起来。
另一方面,浮点数是 Python 最常见的内置数字数据类型之一。float
或浮点数可以描述为带小数点的数字。
print(type(3.445))
输出:
<class 'float'>
在 Python 中使用 float()
函数检查字符串是否为数字
Python 提供了预定义的类型转换函数,可以将一种数据类型转换为另一种数据类型。这些函数包括分别用于将数据类型转换为整数、字符串和浮点数据类型的 int()
、str()
和 float()
函数。
除了通过插入小数点创建浮点数外,还可以通过将字符串隐式或显式转换为浮点数来创建浮点数。
仅当值兼容时,隐式转换由 Python 自动完成,无需用户参与。使用 float()
函数可以将字符串数据类型显式转换为浮点数,如下面的代码所示。
count = "22.23"
print(type(count))
r = float(count)
print(type(r))
输出:
<class 'str'>
<class 'float'>
类型转换是 Python 和一般编程中的一个重要概念。当需要在程序中使用特定数据类型时,这一点尤为明显;在这种情况下,类型转换可以将任何数据转换为所需的数据类型。
在执行文件操作(例如将文件读取和写入各种程序)时,经常会遇到此类情况。
虽然在上面的代码片段中,我们毫不费力地使用了单参数函数 float()
将字符串数据类型转换为浮点数字数据类型,但这种方法并非没有限制。这些限制包括 ValueError
,它通常用于将具有字符序列的字符串转换为浮动数据类型。
如果传递了超出函数范围的数字,则使用 float 函数可能会导致 OverFlow
错误。下面的代码片段说明了当 float 函数转换无效字符串时如何产生 ValueError
。
name = "developer"
rslt = float(name)
print(rslt)
输出:
Traceback (most recent call last):
File "<string>", line 2, in <module>
ValueError: could not convert string to float: 'developer'
ValueError
可以通过确保考虑进行转换的字符串看起来像一个浮点数来避免。
其他实例也可能导致 ValueError
,其中包括空格、逗号或任何其他非特殊字符。因此,无论何时处理文件,都需要事先检查字符串值是否有效以及是否可以转换为浮点数。
但是,如果值太多,尝试检查每个值是低效的。或者,我们可以创建一个函数来检查字符串是否是可以转换为浮点数的有效浮点数。
如果字符串无效,程序应该引发异常而不是抛出错误。
def is_valid_float(element: str) -> bool:
try:
float(element)
return True
except ValueError:
return False
上面的函数接受一个字符串作为参数,并检查它是否是可以转换为浮点数的有效字符串。如果字符串不是可以转换为浮点数的有效字符串,则该函数返回 false,否则返回 true。
在 Python 中使用 isdigit()
和 partition()
函数检查字符串是否为数字
或者,我们可以使用 isdigit()
函数而不是 float()
函数。如果字符串仅包含数字,则 isdigit()
函数返回 true,如果至少有一个字符不是数字,则返回 false。
但是,如果字符串包含浮点数,即使浮点数是有效字符串,此函数也会返回 false。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("4546.4646")
输出:
String is not valid
我们将使用 partition
函数来确保 isdigit()
函数不会返回 false 语句,即使字符串包含浮点数。
这个函数允许我们将包含浮点数的字符串分成几部分来检查它们是否是数字。如果两个部分都是数字,该函数会让我们知道这是一个有效的字符串。
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234.34")
输出:
234.34
String is also valid !
请注意,如果字符串包含不是浮点数的数字,则该函数不需要对字符串进行分区。
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234")
输出:
string is valid
另一方面,如果字符串包含一个浮点数,其中包含一个不能被视为数字的字符,那么该浮点数将不是一个有效的字符串。在这种情况下,有效字符串是指可以使用 float()
函数转换为浮点数的字符串。
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234.rt9")
输出:
string is not valid !
对于以指数形式表示的整数和数字以及任何数字的 Unicode 字符,isdigit()
函数也返回 true。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("4546")
输出:
String is valid
如果字符串只包含上面示例代码块中的数字,我们将 isdigit()
方法与一个函数一起使用,该函数返回一个肯定的消息。但是,当使用不包含数字的参数调用该函数时,该函数会打印一条否定消息,如下面的代码所示。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("Pal Alto")
输出:
String is not valid
因此,使用 isdigit()
函数,我们可以轻松确定字符串是否有效以及是否可以转换为浮点数。包含空格和符号的字符串不能转换为浮点数。
使用 isdigit()
方法,我们还可以确定包含空格的字符串是否有效,如下面的代码所示。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("$4546.343")
输出:
String is not valid
包含任何空格的字符串同样不是有效字符串,不能转换为浮点数。
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("45 8")
输出:
String is not valid
复数在 Python 中的三种内置数字数据类型中同等重要。我们还可以在 complex()
函数旁边使用 float()
函数来确定包含复数的字符串是否也可以转换为浮点数据类型。
complex()
函数将整数和字符串转换为复数。因此使用这两个函数,我们可以确认包含字符序列的字符串不能转换为浮点数,也不能转换为复数。
这些函数还可以检查包含复数的字符串是否可以转换为浮点数,如下面的代码所示。
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("hello"))
输出:
False
相反,由整数或浮点数组成的字符串可以转换为浮点数和复数。因此,如果我们传递具有上述描述的参数,该函数可能会返回 True
。
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("456.45"))
输出:
True
由整数组成的字符串同样有效,可以转换为浮点数和复数。因此,我们也会得到一个 True 语句。
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("456"))
输出:
True
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn相关文章 - Python String
- 在 Python 中从字符串中删除逗号
- 在 Python 中将字符串转换为变量名
- Python 如何去掉字符串中的空格/空白符
- 如何在 Python 中从字符串中提取数字
- Python 如何将字符串转换为时间日期 datetime 格式
- Python2 和 3 中如何将(Unicode)字符串转换为小写