How to Check if a String Is a Number in Python
-
Use the
float()
Function to Check if a String Is a Number in Python -
Use the
isdigit()
andpartition()
Functions to Check if a String Is a Number in Python
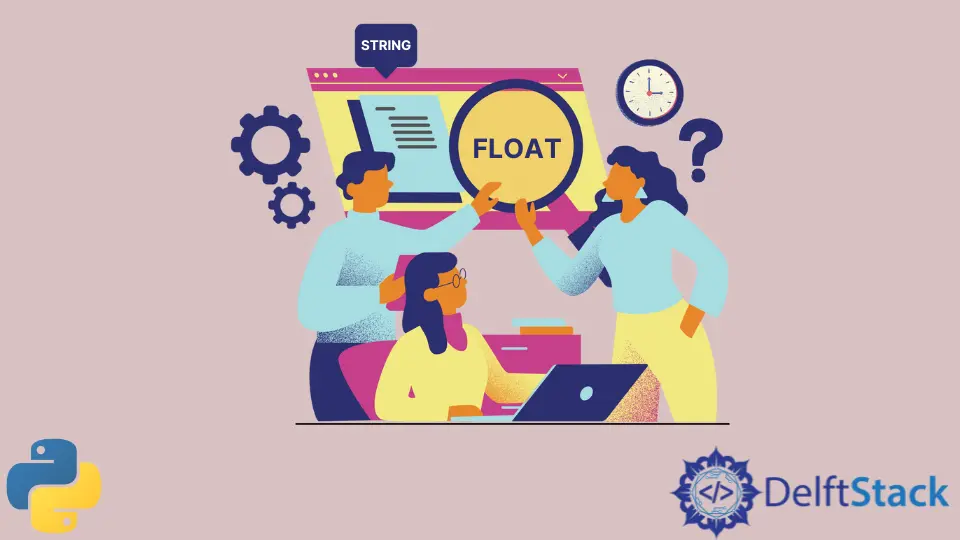
A string is a sequence of characters enclosed by double or single quote marks. Strings that span multiple lines, also known as multiline strings, are enclosed by triple quote marks.
On the other hand, floating-point numbers are one of Python’s most common built-in numeric data types. A float
or a floating-point number may be described as a number with decimal points.
print(type(3.445))
Output:
<class 'float'>
Use the float()
Function to Check if a String Is a Number in Python
Python provides predefined type conversion functions that convert one data type to another. These functions include the int()
, str()
, and float()
functions for converting data types to integer, string, and float data types respectively.
Besides creating a floating-point number by inserting a decimal point, floating-point numbers can also be created through the implicit or explicit conversion of a string to a floating-point number.
Implicit conversion is automatically done by Python without user involvement only if the values are compatible. Explicit conversion of string data type to a floating-point number is made possible using the float()
function as shown in the code below.
count = "22.23"
print(type(count))
r = float(count)
print(type(r))
Output:
<class 'str'>
<class 'float'>
Type conversion is an important concept in Python and programming in general. This is particularly evident when the need arises to use a particular data type in a program; in such an instance, type conversion can convert any data into the desired data type.
Such instances can often be experienced when performing file operations such as reading and writing files into various programs.
While in the code snippet above, we have effortlessly used the one-parameter function float()
to convert the string data type to a float numeric data type, this method is not without limitations. These limitations include the ValueError
, which often arises to convert a string with a sequence of characters to a floating data type.
Using the float function may result in an OverFlow
error if a number out of the range of the function is passed. The code snippet below illustrates how the ValueError
arises when the float function converts an invalid string.
name = "developer"
rslt = float(name)
print(rslt)
Output:
Traceback (most recent call last):
File "<string>", line 2, in <module>
ValueError: could not convert string to float: 'developer'
The ValueError
can be averted by ensuring that the string under consideration for conversion looks like a floating-point number.
Other instances may also lead to the ValueError
, which includes spaces, comma, or any other non-special character. Therefore, whenever working with files, there is a need to check beforehand if string values are valid and are convertible to floating-point numbers.
However, if the values are too many, it is inefficient to try and check every single value. Alternatively, we can create a function that checks if a string is a valid float that can be converted into a floating-point number.
If the string is not valid, the program should raise an exception instead of throwing an error.
def is_valid_float(element: str) -> bool:
try:
float(element)
return True
except ValueError:
return False
The function above accepts a string as an argument and checks if it is a valid string that can be converted to a floating-point number. The function returns false if the string is not a valid string that can be converted into a floating-point number and true if otherwise.
Use the isdigit()
and partition()
Functions to Check if a String Is a Number in Python
Alternatively, we can use the isdigit()
function instead of the float()
function. The isdigit()
function returns true if a string contains digits only and false if at least one character is not a digit.
However, this function returns false if a string contains a float number even though a floating-point number is a valid string.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("4546.4646")
Output:
String is not valid
We will use the partition
function to ensure that the isdigit()
function will not return a false statement even when a string contains a floating-point number.
This function allows us to separate the string containing a floating-point number into parts to check if they are digits. If both parts are digits, the function will let us know that this is a valid string.
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234.34")
Output:
234.34
String is also valid !
Please note that if the string contains a digit that is not a floating-point, the function will not need to partition the string.
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234")
Output:
string is valid
On the other hand, if the string contains a floating-point number that contains a character(s) that cannot be regarded as digits, then the floating-point will not be a valid string. A valid string, in this case, refers to a string that can be converted to a floating-point number using the float()
function.
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234.rt9")
Output:
string is not valid !
The isdigit()
function also returns true for integers and digits represented in exponential form and Unicode characters of any digit.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("4546")
Output:
String is valid
We have used the isdigit()
method alongside a function that returns a positive message if the string only contains numbers in the sample code block above. However, when the function is invoked with an argument containing no number, the function prints a negative message, as shown in the code below.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("Pal Alto")
Output:
String is not valid
Therefore using the isdigit()
function, we can easily determine if a string is valid and can be converted to floating-point numbers. A string that contains whitespace and symbols cannot be converted to a floating-point number.
Using the isdigit()
method, we can also determine if a string that contains whitespaces is valid, as shown in the code below.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("$4546.343")
Output:
String is not valid
A string containing any white space is equally not a valid string and cannot be converted into a floating-point number.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("45 8")
Output:
String is not valid
Complex numbers are equally among the three built-in numeric data types in Python. We can also use the float()
function alongside the complex()
function to determine if a string containing a complex number can also be converted to a floating-point data type.
The complex()
function converts integers and strings to complex numbers. Therefore using both functions, we can confirm that a string that contains a sequence of characters cannot be converted to a floating-point number, nor can it be converted to a complex number.
The functions can also check if a string containing a complex number can be converted to a floating-point number, as shown in the code below.
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("hello"))
Output:
False
On the contrary, a string consisting of an integer or floating-point number can be converted into a floating-point number and a complex number. Therefore, if we pass an argument with the above description, the function will likely return True
instead.
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("456.45"))
Output:
True
A string consisting of an integer is equally valid and can be converted into floating-point and complex numbers. Therefore, we will also get a True statement.
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("456"))
Output:
True
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python