How to Find Maximum Float Value in Python
-
Find Maximum Float Value in Python Using
sys.float_info
-
Find Maximum Float Value in Python Using the
finfo()
Function of theNumpy
Library -
Find Maximum Float Value in Python Using
float('inf')
-
Find Maximum Float Value in Python Using
math.inf
- Conclusion
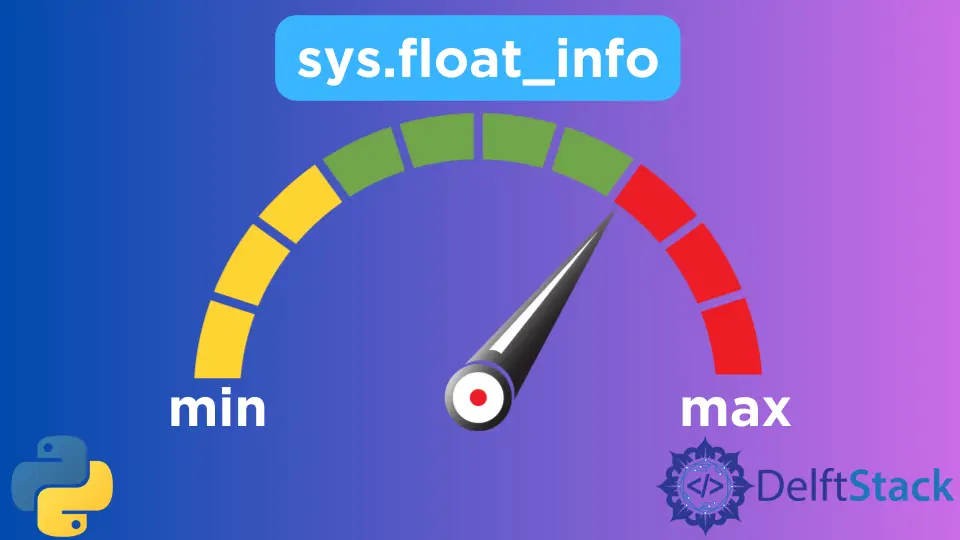
Python supports many data types, such as integer, float, string, etc. All of them have been assigned a minimum and a maximum value, between which the compiler can accept the values.
This article will discuss how to find out the maximum value that the float data type supports.
There are mainly two ways to find the maximum value of float data type in Python. One is the use of sys.float_info
that holds the information about the float data type in Python, and the next is to use the finfo()
function of the numpy
library to return machine limits of the floating-point numbers.
We will also discuss the methods that output infinity (inf)
, which is also referred to as the maximum value of a float. They are float('inf')
and math.inf
.
We will see each one of them in detail in this article.
Find Maximum Float Value in Python Using sys.float_info
The sys.float_info
is a named tuple that holds information such as the precision and internal representation of the float data type.
To use sys.float_info
in our code, we must first import the in-built sys
module defined in Python, which provides certain variables and functions used to manipulate various parts of the Python runtime environment.
However, the sys.float_info
will give us all the information about the float data type. It is demonstrated using the code below.
import sys
print(sys.float_info)
The code above intends to print all the information about the float data type.
Output:
sys.float_info(max=1.7976931348623157e+308, max_exp=1024, max_10_exp=308, min=2.2250738585072014e-308, min_exp=-1021, min_10_exp=-307, dig=15, mant_dig=53, epsilon=2.220446049250313e-16, radix=2, rounds=1)
However, we need to find the maximum value of the float data type. Therefore, we will use sys.float_info.max
, which will only provide the maximum float data type value in Python.
Let us see the code to demonstrate the use of sys.float_info.max
.
import sys
print(sys.float_info.max)
Output:
1.7976931348623157e+308
Find Maximum Float Value in Python Using the finfo()
Function of the Numpy
Library
If you are using the numpy
library of Python, you can easily find the maximum value of the float data type. It contains a function called finfo()
, which takes float
, dtype
or instance
as an argument and returns the machine limit for the floating-point types.
However, before using the finfo()
function, you first need to import the numpy
library in the code. After which, you need to call the finfo
function with np.float64
as the argument where np
is the alias name used for the numpy
library.
Let us see how to code the same.
import numpy as np
float64_info = np.finfo(np.float64)
# Customized print statement
print(
f"finfo(resolution={float64_info.resolution}, min={float64_info.min}, max={float64_info.max}, dtype=float64)"
)
The code above is the customized one to only print the resolution, minimum value, maximum value, and the dtype
. If you’re going to only put print(np.finfo(np.float64))
, expect a longer set of data will be printed.
Output:
finfo(resolution=1e-15, min=-1.7976931348623157e+308, max=1.7976931348623157e+308, dtype=float64)
However, the above function gives the minimum machine limit for the float data type. You can use np.finfo(np.float64).max
for just printing the maximum value of the float data type in Python.
Let us see the code for finding the maximum value of the float data type.
import numpy as np
print(np.finfo(np.float64).max)
Output:
1.7976931348623157e+308
Find Maximum Float Value in Python Using float('inf')
When dealing with very large numbers that exceed the representable range of finite floating-point numbers, Python uses a special notation called “infinity” (inf
).
Positive infinity (+inf
) is used to represent numbers greater than the maximum finite floating-point value, while negative infinity (-inf
) is used to represent numbers smaller than the minimum finite floating-point value.
Let’s see how Python interprets and shows it.
Example code:
max_float_value_inf = float("inf")
print("Maximum Float Value Using float('inf'):", max_float_value_inf)
The code, float('inf')
, creates a floating-point number representing positive infinity and assigns it to the variable max_float_value_inf
.
Then the print("Maximum Float Value Using float('inf'):", max_float_value_inf)
line prints a message indicating that the maximum float value is being displayed using the float('inf')
method, along with the actual value stored in max_float_value_inf
, which is positive infinity.
Output:
Maximum Float Value Using float('inf'): inf
It indicates that the maximum float value is positive infinity, which is used when the number is beyond the representable range of finite floating-point numbers.
The value 1.7976931348623157e+308
is the maximum representable finite floating-point number in Python, and it is often referred to as “infinity” (inf
) in Python because it is beyond the representable range of finite floating-point numbers.
Find Maximum Float Value in Python Using math.inf
The math.inf
constant represents positive infinity, and its counterpart, -math.inf
, represents negative infinity.
These values are used to signify that a number is beyond the representable range of finite floating-point numbers.
Let’s see how this method appears when printed.
Example code:
import math
max_float_value_math_inf = math.inf
print("Maximum Float Value Using math.inf:", max_float_value_math_inf)
The code snippet utilizes the math
module in Python to represent and print the maximum finite positive floating-point value.
It imports the math
module, assigns positive infinity (math.inf
) to a variable (max_float_value_math_inf
), and then prints a message indicating the use of math.inf
for displaying the maximum float value, along with the actual positive infinity value stored in the variable.
Output:
Maximum Float Value Using math.inf: inf
Same as the method above, inf
was printed, and it means that the value is treated as positive infinity.
This is expected behavior and does not mean that the maximum finite float value is not correctly represented. If you need to print the actual value 1.7976931348623157e+308
, you can use the methods involving sys.float_info
or numpy.finfo()
, as shown in the previous examples.
Conclusion
Understanding the limitations and precision of floating-point numbers is crucial in many scientific and computational applications.
In this article, we have seen two different methods to find the maximum value of the float data type in Python. One of them is to use the float_info.max
of the sys
module, and the second method is to use the finfo()
function of the numpy
library in Python.
By employing these tools, developers can make informed decisions when working with numerical data, ensuring accuracy and avoiding pitfalls associated with the limitations of floating-point precision.