Python에서 문자열이 숫자인지 확인
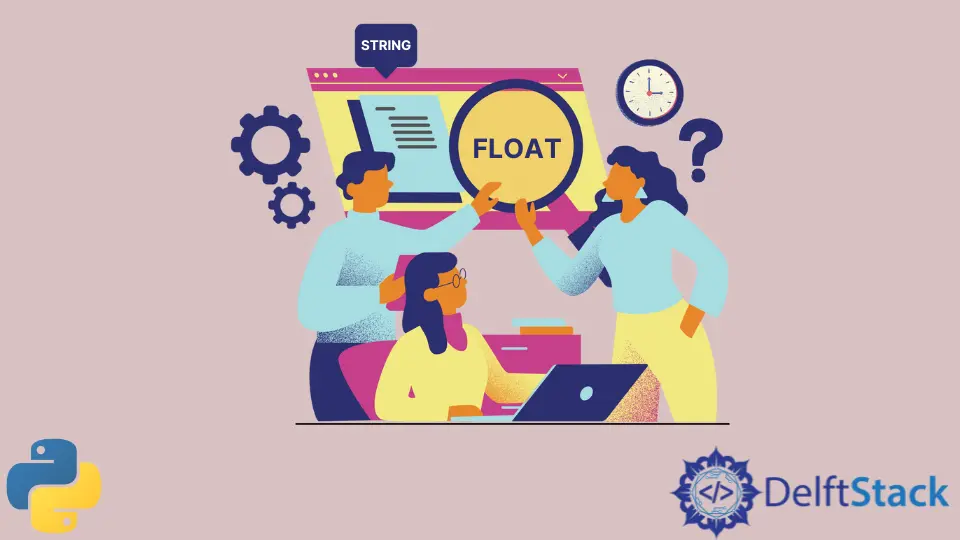
문자열은 큰따옴표나 작은따옴표로 묶인 일련의 문자입니다. 여러 줄에 걸쳐 있는 문자열(여러 줄 문자열이라고도 함)은 삼중 따옴표로 묶습니다.
반면에 부동 소수점 숫자는 Python의 가장 일반적인 내장 숫자 데이터 유형 중 하나입니다. float
또는 부동 소수점 숫자는 소수점이 있는 숫자로 설명될 수 있습니다.
print(type(3.445))
출력:
<class 'float'>
float()
함수를 사용하여 Python에서 문자열이 숫자인지 확인
Python은 한 데이터 유형을 다른 데이터 유형으로 변환하는 미리 정의된 유형 변환 함수를 제공합니다. 이러한 함수에는 데이터 유형을 정수, 문자열 및 부동 소수점 데이터 유형으로 각각 변환하기 위한 int()
, str()
및 float()
함수가 포함됩니다.
소수점을 삽입하여 부동 소수점 숫자를 만드는 것 외에도 부동 소수점 숫자는 문자열을 부동 소수점 숫자로 암시적 또는 명시적으로 변환하여 만들 수도 있습니다.
암시적 변환은 값이 호환되는 경우에만 사용자 개입 없이 Python에서 자동으로 수행됩니다. 아래 코드와 같이 float()
함수를 사용하여 문자열 데이터 유형을 부동 소수점 숫자로 명시적으로 변환할 수 있습니다.
count = "22.23"
print(type(count))
r = float(count)
print(type(r))
출력:
<class 'str'>
<class 'float'>
유형 변환은 일반적으로 Python 및 프로그래밍에서 중요한 개념입니다. 이것은 프로그램에서 특정 데이터 유형을 사용해야 할 때 특히 분명합니다. 이러한 경우 유형 변환은 모든 데이터를 원하는 데이터 유형으로 변환할 수 있습니다.
이러한 인스턴스는 다양한 프로그램에 파일을 읽고 쓰는 것과 같은 파일 작업을 수행할 때 종종 경험할 수 있습니다.
위의 코드 조각에서 문자열 데이터 유형을 부동 숫자 데이터 유형으로 변환하기 위해 단일 매개변수 함수 float()
를 쉽게 사용했지만 이 방법에도 제한이 없습니다. 이러한 제한 사항에는 문자 시퀀스가 있는 문자열을 부동 데이터 유형으로 변환할 때 종종 발생하는 ValueError
가 포함됩니다.
float 함수를 사용하면 함수 범위를 벗어난 숫자가 전달되는 경우 OverFlow
오류가 발생할 수 있습니다. 아래 코드 조각은 float 함수가 잘못된 문자열을 변환할 때 ValueError
가 어떻게 발생하는지 보여줍니다.
name = "developer"
rslt = float(name)
print(rslt)
출력:
Traceback (most recent call last):
File "<string>", line 2, in <module>
ValueError: could not convert string to float: 'developer'
ValueError
는 변환을 고려 중인 문자열이 부동 소수점 숫자처럼 보이도록 하여 방지할 수 있습니다.
다른 인스턴스는 공백, 쉼표 또는 기타 비특수 문자를 포함하는 ValueError
로 이어질 수도 있습니다. 따라서 파일 작업을 할 때마다 문자열 값이 유효하고 부동 소수점 숫자로 변환 가능한지 여부를 미리 확인할 필요가 있습니다.
그러나 값이 너무 많으면 모든 값을 확인하려고 시도하는 것은 비효율적입니다. 또는 문자열이 부동 소수점 숫자로 변환할 수 있는 유효한 부동 소수점인지 확인하는 함수를 만들 수 있습니다.
문자열이 유효하지 않으면 프로그램은 오류를 발생시키는 대신 예외를 발생시켜야 합니다.
def is_valid_float(element: str) -> bool:
try:
float(element)
return True
except ValueError:
return False
위의 함수는 문자열을 인수로 받아 부동 소수점 숫자로 변환할 수 있는 유효한 문자열인지 확인합니다. 이 함수는 문자열이 부동 소수점 숫자로 변환할 수 있는 유효한 문자열이 아니면 false를 반환하고 그렇지 않으면 true를 반환합니다.
isdigit()
및 partition()
함수를 사용하여 Python에서 문자열이 숫자인지 확인
또는 float()
함수 대신 isdigit()
함수를 사용할 수 있습니다. isdigit()
함수는 문자열에 숫자만 포함된 경우 true를 반환하고 하나 이상의 문자가 숫자가 아닌 경우 false를 반환합니다.
그러나 이 함수는 부동 소수점 숫자가 유효한 문자열이더라도 문자열에 부동 소수점 숫자가 포함되어 있으면 false를 반환합니다.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("4546.4646")
출력:
String is not valid
partition
함수를 사용하여 문자열에 부동 소수점 숫자가 포함된 경우에도 isdigit()
함수가 거짓 명령문을 반환하지 않도록 할 것입니다.
이 함수를 사용하면 부동 소수점 숫자가 포함된 문자열을 부분으로 분리하여 숫자인지 확인할 수 있습니다. 두 부분이 모두 숫자인 경우 함수는 이것이 유효한 문자열임을 알려줍니다.
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234.34")
출력:
234.34
String is also valid !
문자열에 부동 소수점이 아닌 숫자가 포함된 경우 함수는 문자열을 분할할 필요가 없습니다.
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234")
출력:
string is valid
반면에 문자열에 숫자로 간주할 수 없는 문자가 포함된 부동 소수점 숫자가 포함된 경우 부동 소수점은 유효한 문자열이 아닙니다. 이 경우 유효한 문자열은 float()
함수를 사용하여 부동 소수점 숫자로 변환할 수 있는 문자열을 나타냅니다.
def check_float(element):
partition = element.partition(".")
if element.isdigit():
newelement = float(element)
print("string is valid")
elif (
(partition[0].isdigit() and partition[1] == "." and partition[2].isdigit())
or (partition[0] == "" and partition[1] == "." and partition[2].isdigit())
or (partition[0].isdigit() and partition[1] == "." and partition[2] == "")
):
newelement = float(element)
print(newelement)
print("String is also valid !")
else:
print("string is not valid !")
check_float("234.rt9")
출력:
string is not valid !
isdigit()
함수는 지수 형식으로 표현된 정수 및 숫자와 모든 숫자의 유니코드 문자에 대해서도 true를 반환합니다.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("4546")
출력:
String is valid
위의 샘플 코드 블록에서 문자열에 숫자만 포함된 경우 긍정적인 메시지를 반환하는 함수와 함께 isdigit()
메서드를 사용했습니다. 그러나 숫자가 포함되지 않은 인수로 함수를 호출하면 아래 코드와 같이 함수가 음수 메시지를 인쇄합니다.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("Pal Alto")
출력:
String is not valid
따라서 isdigit()
함수를 사용하면 문자열이 유효하고 부동 소수점 숫자로 변환될 수 있는지 쉽게 결정할 수 있습니다. 공백과 기호가 포함된 문자열은 부동 소수점 숫자로 변환할 수 없습니다.
isdigit()
메서드를 사용하여 아래 코드와 같이 공백이 포함된 문자열이 유효한지 확인할 수도 있습니다.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("$4546.343")
출력:
String is not valid
공백을 포함하는 문자열은 마찬가지로 유효한 문자열이 아니며 부동 소수점 숫자로 변환할 수 없습니다.
def is_valid_string(element):
if element.isdigit():
print("String is valid")
else:
print("String is not valid")
is_valid_string("45 8")
출력:
String is not valid
복소수는 Python의 세 가지 기본 제공 숫자 데이터 유형에서 동일합니다. 또한 complex()
함수와 함께 float()
함수를 사용하여 복소수가 포함된 문자열도 부동 소수점 데이터 유형으로 변환할 수 있는지 확인할 수 있습니다.
complex()
함수는 정수와 문자열을 복소수로 변환합니다. 따라서 두 함수를 모두 사용하여 일련의 문자를 포함하는 문자열을 부동 소수점 숫자로 변환할 수 없으며 복소수로 변환할 수도 없음을 확인할 수 있습니다.
이 함수는 아래 코드와 같이 복소수가 포함된 문자열을 부동 소수점 숫자로 변환할 수 있는지 여부도 확인할 수 있습니다.
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("hello"))
출력:
False
반대로 정수 또는 부동 소수점 숫자로 구성된 문자열은 부동 소수점 숫자와 복소수로 변환될 수 있습니다. 따라서 위의 설명과 함께 인수를 전달하면 함수가 대신 True
를 반환할 가능성이 높습니다.
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("456.45"))
출력:
True
정수로 구성된 문자열은 동일하게 유효하며 부동 소수점 및 복소수로 변환될 수 있습니다. 따라서 우리는 또한 True 진술을 얻을 것입니다.
def is_valid_string(str):
try:
float(str)
return True
except ValueError:
try:
complex(str)
except ValueError:
return False
print(is_valid_string("456"))
출력:
True
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn관련 문장 - Python String
- Python의 문자열에서 쉼표 제거
- 파이썬 방식으로 문자열이 비어 있는지 확인하는 방법
- Python에서 문자열을 변수 이름으로 변환
- 파이썬에서 문자열에서 공백을 제거하는 방법
- Python의 문자열에서 숫자 추출
- 파이썬에서 문자열을 날짜 / 시간으로 변환하는 방법