Python で文字列を浮動小数点値に変換する
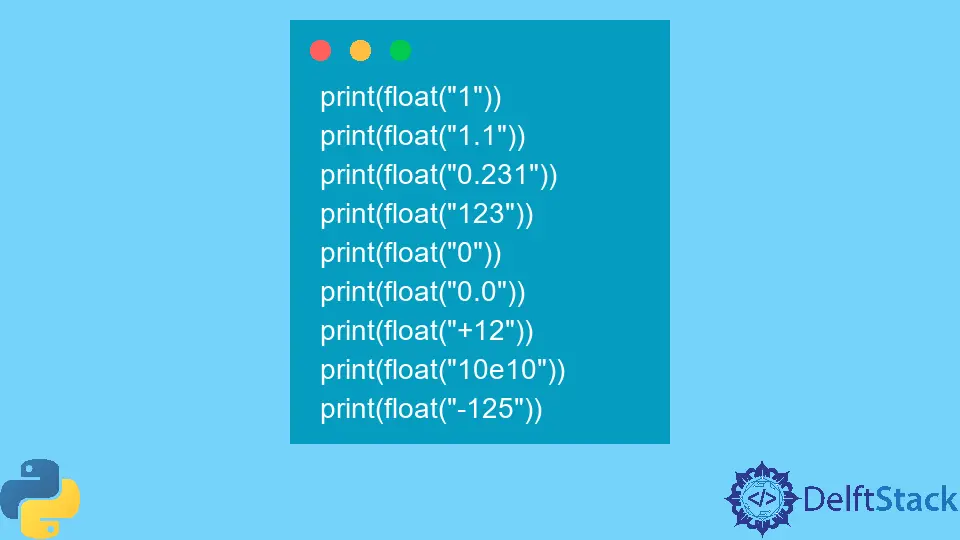
プログラミングでは、データは変数に格納され、これらの変数には特定のデータ型があります。これらのデータ型には、整数、浮動小数点値、文字列、およびブール値が含まれます。
あるデータ型の値を別のデータ型に変換しなければならない状況に遭遇することがあります。たとえば、integer
を float
に、integer
を long
に、integer
を boolean
に、string
を boolean
に変換します。
この記事では、文字列値を浮動小数点値に変換する方法を学習します。
Python で文字列を浮動小数点値に変換する
文字列を float 値に変換するときは、文字列が数値を表すことを確認する必要があります。たとえば、"1"
と"1.0"
は 1.0
に変換できますが、"hello"
と"python is amazing"
を浮動小数点値に変換することはできません。
実際に変換を実行する方法を見てみましょう。これについては、次の Python コードを参照してください。
print(float("1"))
print(float("1.1"))
print(float("0.231"))
print(float("123"))
print(float("0"))
print(float("0.0"))
print(float("+12"))
print(float("10e10"))
print(float("-125"))
出力:
1.0
1.1
0.231
123.0
0.0
0.0
12.0
100000000000.0
-125.0
Python には、文字列を浮動小数点値に変換できる float()
関数があります。文字列だけでなく、この組み込みメソッドを使用して整数を浮動小数点値に変換することもできます。
前述のように、文や単語を表す文字列を浮動小数点値に変換することはできません。float()
メソッドは、そのようなシナリオに対して ValueError
例外をスローします。
次の Python コードはこれを表しています。
print(float("hello"))
出力:
Traceback (most recent call last):
File "<string>", line 1, in <module>
ValueError: could not convert string to float: 'hello'
float()
メソッドに渡す文字列値がわからない場合は、try
および except
ブロックを使用して例外をキャッチし、プログラムの実行を続行できます。これについては、次のコードを参照してください。
strings = ["1.1", "-123.44", "+33.0000", "hello", "python", "112e34", "0"]
for s in strings:
try:
print(float(s))
except ValueError:
print("Conversion failed!")
出力:
1.1
-123.44
33.0
Conversion failed!
Conversion failed!
1.12e+36
0.0
ご覧のとおり、try...except
ブロックは、"hello"
と"python"
の例外をキャッチするのに役立ちました。他の要素については、アルゴリズムはシームレスに実行されました。
関連記事 - Python String
- Python で文字列からコンマを削除する
- Python で文字列を変数名に変換する
- Python 文字列の空白を削除する方法
- Python で文字列から数字を抽出する
- Python が文字列を日時 datetime に変換する方法
- Python 2 および 3 で文字列を小文字に変換する方法
関連記事 - Python Float
- Python で最大フロート値を求める
- Python で Float オブジェクトが呼び出せない問題の修正
- Python で文字列が数値かどうかを調べる
- Python で文字列を 10 進数に変換する
- Python でリストを Float に変換する