How to Fix ValueError: list.remove(x): X Not in List Error in Python
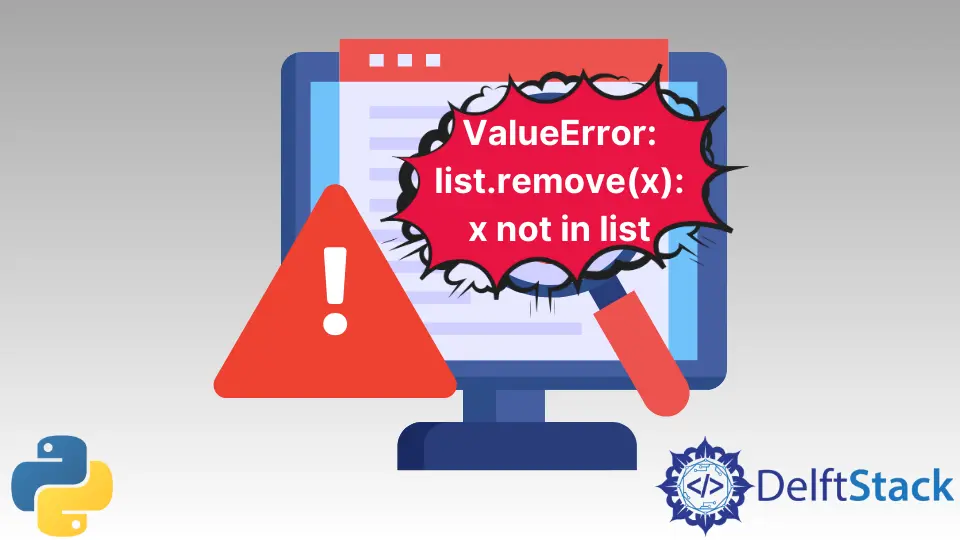
Python uses a list to store multiple items in a single variable. The items in a list are ordered and stored in the index number starting from zero.
The values can be duplicates and are changeable. You can add, change, and remove items in the list.
The remove()
method removes the specified item from a list. While removing the items, sometimes you may encounter an error saying list.remove(x): x not in list
.
The item you specify in the remove()
method is not present in a list. This tutorial will teach you the correct way to remove an item from the list in Python.
Fix the ValueError: list.remove(x): x not in list
Error in Python
The remove()
method takes only one argument. You can remove a single argument at a time.
The remove
method removes the first occurrence of an item in a list. It means the first instance of an item will only be removed when there are multiple instances of an item.
The following example creates a list mylist
.
mylist = ["science", "maths", "computer", "english"]
You can view the items in a list using the print()
method.
print(mylist)
Output:
['science', 'maths', 'computer', 'english']
You can use the command below to remove computer
from mylist
.
mylist.remove("computer")
Next, confirm the items in a list.
print(mylist)
Output:
['science', 'maths', 'english']
As you can see, the computer
element has been successfully removed from the list.
Now, if you try to remove computer
from a list, you will get ValueError: list.remove(x): x not in list
because the computer
element is not in a list.
mylist.remove("computer")
Output:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: list.remove(x): x not in list
Use if...in
to Check if an Item Exists in the List Before Removing
You can check whether the item exists in the list using the if...in
keywords.
if "computer" in mylist:
mylist.remove("computer")
else:
print("computer is not in the list.")
print(mylist)
The above example first checks if the computer
is in the list. If it exists, the remove()
method will remove the item from the list, and the last command prints the updated list.
The else
statement is executed and prints the list if it does not exist in the list.
Output:
computer is not in the list.
['science', 'maths', 'english']
It can be useful when you do not want to get an exception ValueError
in the output.
Remove Multiple List Items One-By-One
You can use two methods to remove multiple items from a list in Python. This error may also occur when two or more items or strings are removed at once.
Since the remove()
method takes only one argument, you must remove one item at a time in Python. Here is an example of removing two items in a list one by one.
mylist = ["science", "maths", "computer", "english"]
mylist.remove("maths")
mylist.remove("english")
print(mylist)
Output:
['science', 'computer']
Remove Multiple List Items Using the for
Loop
Another way is to use the for
loop for removing multiple items in a Python list. The following example uses a single remove
command with a for
loop to remove multiple items from a list.
mylist = ["science", "maths", "computer", "english"]
for item in ["maths", "english"]:
mylist.remove(item)
print(mylist)
Output:
['science', 'computer']
Now you know different ways to remove items in a list.
The ValueError: list.remove(x): x not in list
occurs if the item you specify is not found in a list. We hope now you understand the cause of the problem and how to handle it in Python.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python