How to Find Methods of a Python Object
-
Find Methods of a Python Object Using the
dir
Method -
Find Python Object Type Using the
type
Function -
Find Python Object Id Using the
id
Function -
Find Methods of a Python Object Using the
inspect
Module -
Find Python Objects Using the
hasattr()
Method -
Find Objects Using the
getattr()
Method
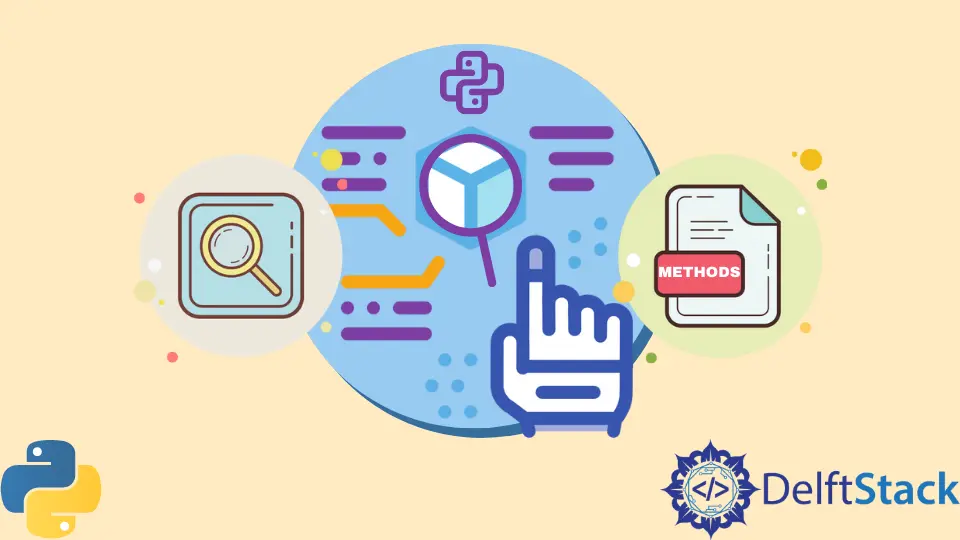
In Python programming, the ability to find methods of a Python object dynamically is called introspection. Since everything in Python is an object, we can easily find out its objects at runtime.
We can examine those using the built-in functions and modules. It is especially useful when we want to know the information without reading the source code.e
This article covers the six easy ways we can use to find the methods of a Python object. Let’s dive into it.
Find Methods of a Python Object Using the dir
Method
The first method to find the methods is to use the dir()
function. This function takes an object as an argument and returns a list of attributes and methods of that object.
The syntax for this function is:
# python 3.x
dir(object)
For example:
# python 3.x
my_object = ["a", "b", "c"]
dir(my_object)
Output:
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
From the output, we can observe that it has returned all of the methods of the object.
The functions starting with a double underscore are called dunder methods. These methods are called wrapper objects. For instance, the dict()
function would call the __dict__()
method.
We have created this basic Vehicle Python class:
# python 3.x
class Vehicle:
def __init__(self, wheels=4, colour="red"):
self.wheels = wheels
self.colour = colour
def repaint(self, colour=None):
self.colour = colour
If we make an object of this class and run the dir()
function, we can see the following output:
# python 3.x
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'colour', 'repaint', 'wheels']
We can see that it lists all the methods as well as their attributes. It shows the methods that we have created, but it also lists all the built-in methods of this class.
Furthermore, we can also check if the method is callable by using the callable()
function and passing the object as an argument.
Find Python Object Type Using the type
Function
The second method is to use the type()
function. The type()
function is used to return the type of an object.
We can pass any object or value in the argument of the type()
function. For example:
# python 3.x
print(type(my_object))
print(type(1))
print(type("hello"))
This will display the following output:
<class 'list'>
<class 'int'>
<class 'str'>
The type()
function has returned the type of an object.
Find Python Object Id Using the id
Function
To find out the id of an object in Python, we will use the id()
function.
This function returns a special id of any object that is passed as an argument. The id is similar to a special place in the memory for that particular object.
For example:
# python 3.x
print(id(my_object))
print(id(1))
print(id("Hello"))
We will get a similar output after executing these commands:
140234778692576
94174513879552
140234742627312
Find Methods of a Python Object Using the inspect
Module
The inspect
module is another method we can use to view information about live Python objects. The syntax for this module is:
# python 3.x
import inspect
print(inspect.getmembers(object))
The first step is to import the inspect
module. After that, we will call the getmembers()
function from the inspect
module and pass the object as an argument.
For example:
# python 3.x
print(inspect.getmembers(my_object))
print(inspect.getmembers(Vehicle))
In the above example, we have inspected two objects: a list and the object of Vehicle class. After executing the code, we are getting this Output:
# python 3.x
[('__add__', <method-wrapper '__add__' of list object at 0x7f8af42b4be0>), ('__class__', <class 'list'>), ('__contains__', <method-wrapper '__contains__' of list object at 0x7f8af42b4be0>), ('__delattr__', <method-wrapper '__delattr__' of list object at 0x7f8af42b4be0>), ('__delitem__', <method-wrapper '__delitem__' of list object at 0x7f8af42b4be0>), ('__dir__', <built-in method __dir__ of list object at 0x7f8af42b4be0>), ('__doc__', 'Built-in mutable sequence.\n\nIf no argument is given, the constructor creates a new empty list.\nThe argument must be an iterable if specified.'), ('__eq__', <method-wrapper '__eq__' of list object at 0x7f8af42b4be0>), ('__format__', <built-in method __format__ of list object at 0x7f8af42b4be0>), ('__ge__', <method-wrapper '__ge__' of list object at 0x7f8af42b4be0>), ('__getattribute__', <method-wrapper '__getattribute__' of list object at 0x7f8af42b4be0>), ('__getitem__', <built-in method __getitem__ of list object at 0x7f8af42b4be0>), ('__gt__', <method-wrapper '__gt__' of list object at 0x7f8af42b4be0>), ('__hash__', None), ('__iadd__', <method-wrapper '__iadd__' of list object at 0x7f8af42b4be0>), ('__imul__', <method-wrapper '__imul__' of list object at 0x7f8af42b4be0>), ('__init__', <method-wrapper '__init__' of list object at 0x7f8af42b4be0>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b668d5a0>), ('__iter__', <method-wrapper '__iter__' of list object at 0x7f8af42b4be0>), ('__le__', <method-wrapper '__le__' of list object at 0x7f8af42b4be0>), ('__len__', <method-wrapper '__len__' of list object at 0x7f8af42b4be0>), ('__lt__', <method-wrapper '__lt__' of list object at 0x7f8af42b4be0>), ('__mul__', <method-wrapper '__mul__' of list object at 0x7f8af42b4be0>), ('__ne__', <method-wrapper '__ne__' of list object at 0x7f8af42b4be0>), ('__new__', <built-in method __new__ of type object at 0x55a6b668d5a0>), ('__reduce__', <built-in method __reduce__ of list object at 0x7f8af42b4be0>), ('__reduce_ex__', <built-in method __reduce_ex__ of list object at 0x7f8af42b4be0>), ('__repr__', <method-wrapper '__repr__' of list object at 0x7f8af42b4be0>), ('__reversed__', <built-in method __reversed__ of list object at 0x7f8af42b4be0>), ('__rmul__', <method-wrapper '__rmul__' of list object at 0x7f8af42b4be0>), ('__setattr__', <method-wrapper '__setattr__' of list object at 0x7f8af42b4be0>), ('__setitem__', <method-wrapper '__setitem__' of list object at 0x7f8af42b4be0>), ('__sizeof__', <built-in method __sizeof__ of list object at 0x7f8af42b4be0>), ('__str__', <method-wrapper '__str__' of list object at 0x7f8af42b4be0>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b668d5a0>), ('append', <built-in method append of list object at 0x7f8af42b4be0>), ('clear', <built-in method clear of list object at 0x7f8af42b4be0>), ('copy', <built-in method copy of list object at 0x7f8af42b4be0>), ('count', <built-in method count of list object at 0x7f8af42b4be0>), ('extend', <built-in method extend of list object at 0x7f8af42b4be0>), ('index', <built-in method index of list object at 0x7f8af42b4be0>), ('insert', <built-in method insert of list object at 0x7f8af42b4be0>), ('pop', <built-in method pop of list object at 0x7f8af42b4be0>), ('remove', <built-in method remove of list object at 0x7f8af42b4be0>), ('reverse', <built-in method reverse of list object at 0x7f8af42b4be0>), ('sort', <built-in method sort of list object at 0x7f8af42b4be0>)] [('__class__', <class '__main__.Vehicle'>), ('__delattr__', <method-wrapper '__delattr__' of Vehicle object at 0x7f8af813a350>), ('__dict__', {'wheels': 4, 'colour': 'red'}), ('__dir__', <built-in method __dir__ of Vehicle object at 0x7f8af813a350>), ('__doc__', None), ('__eq__', <method-wrapper '__eq__' of Vehicle object at 0x7f8af813a350>), ('__format__', <built-in method __format__ of Vehicle object at 0x7f8af813a350>), ('__ge__', <method-wrapper '__ge__' of Vehicle object at 0x7f8af813a350>), ('__getattribute__', <method-wrapper '__getattribute__' of Vehicle object at 0x7f8af813a350>), ('__gt__', <method-wrapper '__gt__' of Vehicle object at 0x7f8af813a350>), ('__hash__', <method-wrapper '__hash__' of Vehicle object at 0x7f8af813a350>), ('__init__', <bound method Vehicle.__init__ of <__main__.Vehicle object at 0x7f8af813a350>>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b9617e20>), ('__le__', <method-wrapper '__le__' of Vehicle object at 0x7f8af813a350>), ('__lt__', <method-wrapper '__lt__' of Vehicle object at 0x7f8af813a350>), ('__module__', '__main__'), ('__ne__', <method-wrapper '__ne__' of Vehicle object at 0x7f8af813a350>), ('__new__', <built-in method __new__ of type object at 0x55a6b6698ba0>), ('__reduce__', <built-in method __reduce__ of Vehicle object at 0x7f8af813a350>), ('__reduce_ex__', <built-in method __reduce_ex__ of Vehicle object at 0x7f8af813a350>), ('__repr__', <method-wrapper '__repr__' of Vehicle object at 0x7f8af813a350>), ('__setattr__', <method-wrapper '__setattr__' of Vehicle object at 0x7f8af813a350>), ('__sizeof__', <built-in method __sizeof__ of Vehicle object at 0x7f8af813a350>), ('__str__', <method-wrapper '__str__' of Vehicle object at 0x7f8af813a350>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b9617e20>), ('__weakref__', None), ('colour', 'red'), ('repaint', <bound method Vehicle.repaint of <__main__.Vehicle object at 0x7f8af813a350>>), ('wheels', 4)]
Find Python Objects Using the hasattr()
Method
Lastly, we can also use the hasattr()
method to find out the methods of a Python object. This function checks if an object has an attribute.
The syntax for this method is:
# python 3.x
hasattr(object, attribute)
The function takes two arguments: object and attribute. It checks if the attribute is present in that particular object.
For example:
# python 3.x
print(hasattr(my_object, "__doc__"))
This function will return True
if the attribute exists. Otherwise, it will return False
. Furthermore, once we have found the method, we can use the help()
function to view its documentation.
For example:
# python 3.x
help(object.method)
Find Objects Using the getattr()
Method
In contrast to hasattr()
method, the getattr()
method returns the contents of an attribute if it exists for that particular Python object.
The syntax for this function is:
# python 3.x
getattr(object, attribute)
For example:
# python 3.x
print(getattr(my_object, "__doc__"))
Output:
Built-in mutable sequence.
If no argument is given, the constructor creates a new empty list.
The argument must be an iterable if specified.
From the output, it is clear that the attribute exists. Hence, it has returned its contents with details on how this method works.
So far, we have looked at several methods to perform object introspection. In other words, we have listed the methods and attributes of a Python object in 5 different ways.
By following this article, we should be able to assess Python objects and perform introspection.