Python オブジェクトのメソッドを 6つの方法で検索
-
dir
メソッドを使用して Python オブジェクトのメソッドを検索する -
type
関数を使用して Python オブジェクトタイプを検索する -
id
関数を使用して Python オブジェクト ID を検索する -
inspect
モジュールを使用して Python オブジェクトのメソッドを検索する -
hasattr()
メソッドを使用して Python オブジェクトを検索する -
getattr()
メソッドを使用してオブジェクトを検索する
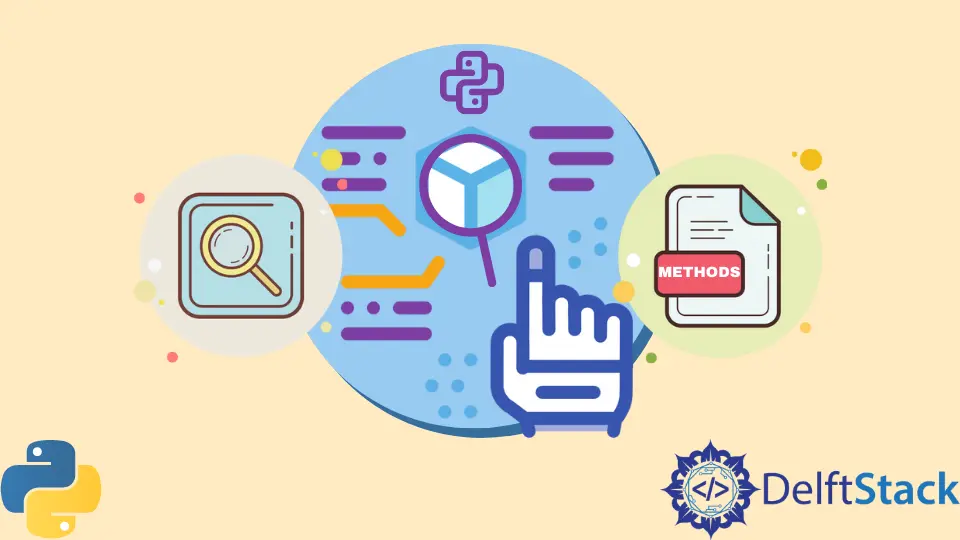
Python プログラミングでは、Python オブジェクトのメソッドを動的に検索する機能はイントロスペクションと呼ばれます。Python のすべてがオブジェクトであるため、実行時にそのオブジェクトを簡単に見つけることができます。
組み込みの関数やモジュールを使って調べることができます。ソースコードを読まずに情報を知りたいときに特に便利です。
この記事では、Python オブジェクトのメソッドを見つけるために使用できる 6つの簡単な方法について説明します。それに飛び込みましょう。
dir
メソッドを使用して Python オブジェクトのメソッドを検索する
メソッドを見つける最初のメソッドは、dir()
関数を使用することです。この関数は、オブジェクトを引数として受け取り、そのオブジェクトの属性とメソッドのリストを返します。
この関数の構文は次のとおりです。
# python 3.x
dir(object)
例えば:
# python 3.x
my_object = ["a", "b", "c"]
dir(my_object)
出力:
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
出力から、オブジェクトのすべてのメソッドが返されたことがわかります。
二重アンダースコアで始まる関数は、dunder メソッドと呼ばれます。これらのメソッドはラッパーオブジェクトと呼ばれます。たとえば、dict()
関数は __dict__()
メソッドを呼び出します。
この基本的な VehiclePython クラスを作成しました。
# python 3.x
class Vehicle:
def __init__(self, wheels=4, colour="red"):
self.wheels = wheels
self.colour = colour
def repaint(self, colour=None):
self.colour = colour
このクラスのオブジェクトを作成して dir()
関数を実行すると、次の出力が表示されます。
# python 3.x
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'colour', 'repaint', 'wheels']
すべてのメソッドとその属性がリストされていることがわかります。作成したメソッドが表示されますが、このクラスのすべての組み込みメソッドも一覧表示されます。
さらに、callable()
関数を使用し、オブジェクトを引数として渡すことで、メソッドが呼び出し可能かどうかを確認することもできます。
type
関数を使用して Python オブジェクトタイプを検索する
2 番目の方法は、type()
関数を使用することです。type()
関数は、オブジェクトの型を返すために使用されます。
type()
関数の引数に任意のオブジェクトまたは値を渡すことができます。例えば:
# python 3.x
print(type(my_object))
print(type(1))
print(type("hello"))
これにより、次の出力が表示されます。
<class 'list'>
<class 'int'>
<class 'str'>
type()
関数はオブジェクトの型を返しました。
id
関数を使用して Python オブジェクト ID を検索する
Python でオブジェクトの ID を見つけるには、id()
関数を使用します。
この関数は、引数として渡されるオブジェクトの特別な ID を返します。id は、その特定のオブジェクトのメモリ内の特別な場所に似ています。
例えば:
# python 3.x
print(id(my_object))
print(id(1))
print(id("Hello"))
これらのコマンドを実行すると、同様の出力が得られます。
140234778692576
94174513879552
140234742627312
inspect
モジュールを使用して Python オブジェクトのメソッドを検索する
inspect
モジュールは、ライブ Python オブジェクトに関する情報を表示するために使用できるもう 1つのメソッドです。このモジュールの構文は次のとおりです。
# python 3.x
import inspect
print(inspect.getmembers(object))
最初のステップは、inspect
モジュールをインポートすることです。その後、inspect
モジュールから getmembers()
関数を呼び出し、オブジェクトを引数として渡します。
例えば:
# python 3.x
print(inspect.getmembers(my_object))
print(inspect.getmembers(Vehicle))
上記の例では、リストと Vehicle クラスのオブジェクトの 2つのオブジェクトを検査しました。コードを実行した後、次の出力が得られます。
# python 3.x
[('__add__', <method-wrapper '__add__' of list object at 0x7f8af42b4be0>), ('__class__', <class 'list'>), ('__contains__', <method-wrapper '__contains__' of list object at 0x7f8af42b4be0>), ('__delattr__', <method-wrapper '__delattr__' of list object at 0x7f8af42b4be0>), ('__delitem__', <method-wrapper '__delitem__' of list object at 0x7f8af42b4be0>), ('__dir__', <built-in method __dir__ of list object at 0x7f8af42b4be0>), ('__doc__', 'Built-in mutable sequence.\n\nIf no argument is given, the constructor creates a new empty list.\nThe argument must be an iterable if specified.'), ('__eq__', <method-wrapper '__eq__' of list object at 0x7f8af42b4be0>), ('__format__', <built-in method __format__ of list object at 0x7f8af42b4be0>), ('__ge__', <method-wrapper '__ge__' of list object at 0x7f8af42b4be0>), ('__getattribute__', <method-wrapper '__getattribute__' of list object at 0x7f8af42b4be0>), ('__getitem__', <built-in method __getitem__ of list object at 0x7f8af42b4be0>), ('__gt__', <method-wrapper '__gt__' of list object at 0x7f8af42b4be0>), ('__hash__', None), ('__iadd__', <method-wrapper '__iadd__' of list object at 0x7f8af42b4be0>), ('__imul__', <method-wrapper '__imul__' of list object at 0x7f8af42b4be0>), ('__init__', <method-wrapper '__init__' of list object at 0x7f8af42b4be0>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b668d5a0>), ('__iter__', <method-wrapper '__iter__' of list object at 0x7f8af42b4be0>), ('__le__', <method-wrapper '__le__' of list object at 0x7f8af42b4be0>), ('__len__', <method-wrapper '__len__' of list object at 0x7f8af42b4be0>), ('__lt__', <method-wrapper '__lt__' of list object at 0x7f8af42b4be0>), ('__mul__', <method-wrapper '__mul__' of list object at 0x7f8af42b4be0>), ('__ne__', <method-wrapper '__ne__' of list object at 0x7f8af42b4be0>), ('__new__', <built-in method __new__ of type object at 0x55a6b668d5a0>), ('__reduce__', <built-in method __reduce__ of list object at 0x7f8af42b4be0>), ('__reduce_ex__', <built-in method __reduce_ex__ of list object at 0x7f8af42b4be0>), ('__repr__', <method-wrapper '__repr__' of list object at 0x7f8af42b4be0>), ('__reversed__', <built-in method __reversed__ of list object at 0x7f8af42b4be0>), ('__rmul__', <method-wrapper '__rmul__' of list object at 0x7f8af42b4be0>), ('__setattr__', <method-wrapper '__setattr__' of list object at 0x7f8af42b4be0>), ('__setitem__', <method-wrapper '__setitem__' of list object at 0x7f8af42b4be0>), ('__sizeof__', <built-in method __sizeof__ of list object at 0x7f8af42b4be0>), ('__str__', <method-wrapper '__str__' of list object at 0x7f8af42b4be0>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b668d5a0>), ('append', <built-in method append of list object at 0x7f8af42b4be0>), ('clear', <built-in method clear of list object at 0x7f8af42b4be0>), ('copy', <built-in method copy of list object at 0x7f8af42b4be0>), ('count', <built-in method count of list object at 0x7f8af42b4be0>), ('extend', <built-in method extend of list object at 0x7f8af42b4be0>), ('index', <built-in method index of list object at 0x7f8af42b4be0>), ('insert', <built-in method insert of list object at 0x7f8af42b4be0>), ('pop', <built-in method pop of list object at 0x7f8af42b4be0>), ('remove', <built-in method remove of list object at 0x7f8af42b4be0>), ('reverse', <built-in method reverse of list object at 0x7f8af42b4be0>), ('sort', <built-in method sort of list object at 0x7f8af42b4be0>)] [('__class__', <class '__main__.Vehicle'>), ('__delattr__', <method-wrapper '__delattr__' of Vehicle object at 0x7f8af813a350>), ('__dict__', {'wheels': 4, 'colour': 'red'}), ('__dir__', <built-in method __dir__ of Vehicle object at 0x7f8af813a350>), ('__doc__', None), ('__eq__', <method-wrapper '__eq__' of Vehicle object at 0x7f8af813a350>), ('__format__', <built-in method __format__ of Vehicle object at 0x7f8af813a350>), ('__ge__', <method-wrapper '__ge__' of Vehicle object at 0x7f8af813a350>), ('__getattribute__', <method-wrapper '__getattribute__' of Vehicle object at 0x7f8af813a350>), ('__gt__', <method-wrapper '__gt__' of Vehicle object at 0x7f8af813a350>), ('__hash__', <method-wrapper '__hash__' of Vehicle object at 0x7f8af813a350>), ('__init__', <bound method Vehicle.__init__ of <__main__.Vehicle object at 0x7f8af813a350>>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b9617e20>), ('__le__', <method-wrapper '__le__' of Vehicle object at 0x7f8af813a350>), ('__lt__', <method-wrapper '__lt__' of Vehicle object at 0x7f8af813a350>), ('__module__', '__main__'), ('__ne__', <method-wrapper '__ne__' of Vehicle object at 0x7f8af813a350>), ('__new__', <built-in method __new__ of type object at 0x55a6b6698ba0>), ('__reduce__', <built-in method __reduce__ of Vehicle object at 0x7f8af813a350>), ('__reduce_ex__', <built-in method __reduce_ex__ of Vehicle object at 0x7f8af813a350>), ('__repr__', <method-wrapper '__repr__' of Vehicle object at 0x7f8af813a350>), ('__setattr__', <method-wrapper '__setattr__' of Vehicle object at 0x7f8af813a350>), ('__sizeof__', <built-in method __sizeof__ of Vehicle object at 0x7f8af813a350>), ('__str__', <method-wrapper '__str__' of Vehicle object at 0x7f8af813a350>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b9617e20>), ('__weakref__', None), ('colour', 'red'), ('repaint', <bound method Vehicle.repaint of <__main__.Vehicle object at 0x7f8af813a350>>), ('wheels', 4)]
hasattr()
メソッドを使用して Python オブジェクトを検索する
最後に、hasattr()
メソッドを使用して Python オブジェクトのメソッドを見つけることもできます。この関数は、オブジェクトに属性があるかどうかをチェックします。
このメソッドの構文は次のとおりです。
# python 3.x
hasattr(object, attribute)
この関数は、オブジェクトと属性の 2つの引数を取ります。属性がその特定のオブジェクトに存在するかどうかをチェックします。
例えば:
# python 3.x
print(hasattr(my_object, "__doc__"))
属性が存在する場合、この関数は True
を返します。それ以外の場合は、False
を返します。さらに、メソッドが見つかったら、help()
関数を使用してそのドキュメントを表示できます。
例えば:
# python 3.x
help(object.method)
getattr()
メソッドを使用してオブジェクトを検索する
hasattr()
メソッドとは対照的に、getattr()
メソッドは、特定の Python オブジェクトに存在する場合、属性の内容を返します。
この関数の構文は次のとおりです。
# python 3.x
getattr(object, attribute)
例えば:
# python 3.x
print(getattr(my_object, "__doc__"))
出力:
Built-in mutable sequence.
If no argument is given, the constructor creates a new empty list.
The argument must be an iterable if specified.
出力から、属性が存在することは明らかです。したがって、このメソッドがどのように機能するかについての詳細を含む内容を返しました。
これまで、オブジェクトのイントロスペクションを実行するためのいくつかの方法を見てきました。つまり、Python オブジェクトのメソッドと属性を 5つの異なる方法でリストしました。
この記事に従うことで、Python オブジェクトを評価し、イントロスペクションを実行できるようになります。
このガイドが役に立った場合は、共有してください。