Encontrar métodos de un objeto Python de 6 formas
-
Encuentre métodos de un objeto Python usando el método
dir
-
Encuentra el tipo de objeto Python usando la función
tipo
-
Encuentra el ID de objeto de Python usando la función
id
-
Encuentre métodos de un objeto Python usando el módulo
inspect
-
Encuentra objetos de Python usando el método
hasattr()
-
Encontrar objetos usando el método
getattr()
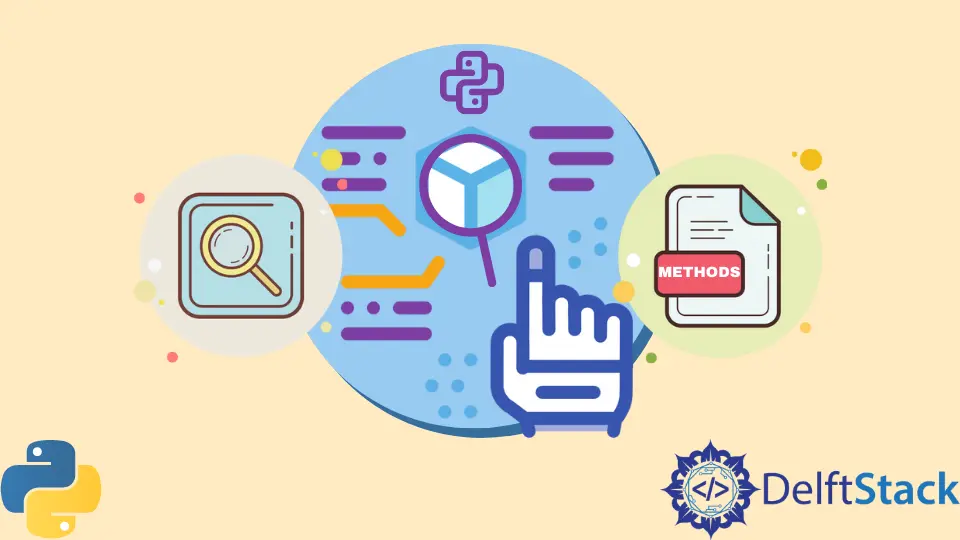
En la programación de Python, la capacidad de encontrar métodos de un objeto de Python de forma dinámica se denomina introspección. Dado que todo en Python es un objeto, podemos encontrar fácilmente sus objetos en tiempo de ejecución.
Podemos examinar aquellos que utilizan las funciones y módulos integrados. Es especialmente útil cuando queremos conocer la información sin leer el código fuente.
Este artículo cubre las seis formas sencillas que podemos utilizar para encontrar los métodos de un objeto Python. Vamos a sumergirnos en ello.
Encuentre métodos de un objeto Python usando el método dir
El primer método para encontrar los métodos es usar la función dir()
. Esta función toma un objeto como argumento y devuelve una lista de atributos y métodos de ese objeto.
La sintaxis de esta función es:
# python 3.x
dir(object)
Por ejemplo:
# python 3.x
my_object = ["a", "b", "c"]
dir(my_object)
Producción :
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
De la salida, podemos observar que ha devuelto todos los métodos del objeto.
Las funciones que comienzan con un guión bajo doble se denominan métodos dunder. Estos métodos se denominan objetos envoltorios. Por ejemplo, la función dict()
llamaría al método __dict__()
.
Hemos creado esta clase básica de Python para vehículos:
# python 3.x
class Vehicle:
def __init__(self, wheels=4, colour="red"):
self.wheels = wheels
self.colour = colour
def repaint(self, colour=None):
self.colour = colour
Si hacemos un objeto de esta clase y ejecutamos la función dir()
, podemos ver el siguiente Resultado:
# python 3.x
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'colour', 'repaint', 'wheels']
Podemos ver que enumera todos los métodos y sus atributos. Muestra los métodos que hemos creado, pero también enumera todos los métodos integrados de esta clase.
Además, también podemos verificar si el método es invocable usando la función callable()
y pasando el objeto como argumento.
Encuentra el tipo de objeto Python usando la función tipo
El segundo método consiste en utilizar la función type()
. La función type()
se utiliza para devolver el tipo de un objeto.
Podemos pasar cualquier objeto o valor en el argumento de la función type()
. Por ejemplo:
# python 3.x
print(type(my_object))
print(type(1))
print(type("hello"))
Esto mostrará la siguiente salida:
<class 'list'>
<class 'int'>
<class 'str'>
La función type()
ha devuelto el tipo de un objeto.
Encuentra el ID de objeto de Python usando la función id
Para averiguar el id de un objeto en Python, usaremos la función id()
.
Esta función devuelve un id especial de cualquier objeto que se pase como argumento. La identificación es similar a un lugar especial en la memoria para ese objeto en particular.
Por ejemplo:
# python 3.x
print(id(my_object))
print(id(1))
print(id("Hello"))
Obtendremos un resultado similar después de ejecutar estos comandos:
140234778692576
94174513879552
140234742627312
Encuentre métodos de un objeto Python usando el módulo inspect
El módulo inspect
es otro método que podemos utilizar para ver información sobre los objetos activos de Python. La sintaxis de este módulo es:
# python 3.x
import inspect
print(inspect.getmembers(object))
El primer paso es importar el módulo inspect
. Después de eso, llamaremos a la función getmembers()
desde el módulo inspect
y pasaremos el objeto como argumento.
Por ejemplo:
# python 3.x
print(inspect.getmembers(my_object))
print(inspect.getmembers(Vehicle))
En el ejemplo anterior, hemos inspeccionado dos objetos: una lista y el objeto de la clase Vehículo. Después de ejecutar el código, obtenemos este Resultado:
# python 3.x
[('__add__', <method-wrapper '__add__' of list object at 0x7f8af42b4be0>), ('__class__', <class 'list'>), ('__contains__', <method-wrapper '__contains__' of list object at 0x7f8af42b4be0>), ('__delattr__', <method-wrapper '__delattr__' of list object at 0x7f8af42b4be0>), ('__delitem__', <method-wrapper '__delitem__' of list object at 0x7f8af42b4be0>), ('__dir__', <built-in method __dir__ of list object at 0x7f8af42b4be0>), ('__doc__', 'Built-in mutable sequence.\n\nIf no argument is given, the constructor creates a new empty list.\nThe argument must be an iterable if specified.'), ('__eq__', <method-wrapper '__eq__' of list object at 0x7f8af42b4be0>), ('__format__', <built-in method __format__ of list object at 0x7f8af42b4be0>), ('__ge__', <method-wrapper '__ge__' of list object at 0x7f8af42b4be0>), ('__getattribute__', <method-wrapper '__getattribute__' of list object at 0x7f8af42b4be0>), ('__getitem__', <built-in method __getitem__ of list object at 0x7f8af42b4be0>), ('__gt__', <method-wrapper '__gt__' of list object at 0x7f8af42b4be0>), ('__hash__', None), ('__iadd__', <method-wrapper '__iadd__' of list object at 0x7f8af42b4be0>), ('__imul__', <method-wrapper '__imul__' of list object at 0x7f8af42b4be0>), ('__init__', <method-wrapper '__init__' of list object at 0x7f8af42b4be0>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b668d5a0>), ('__iter__', <method-wrapper '__iter__' of list object at 0x7f8af42b4be0>), ('__le__', <method-wrapper '__le__' of list object at 0x7f8af42b4be0>), ('__len__', <method-wrapper '__len__' of list object at 0x7f8af42b4be0>), ('__lt__', <method-wrapper '__lt__' of list object at 0x7f8af42b4be0>), ('__mul__', <method-wrapper '__mul__' of list object at 0x7f8af42b4be0>), ('__ne__', <method-wrapper '__ne__' of list object at 0x7f8af42b4be0>), ('__new__', <built-in method __new__ of type object at 0x55a6b668d5a0>), ('__reduce__', <built-in method __reduce__ of list object at 0x7f8af42b4be0>), ('__reduce_ex__', <built-in method __reduce_ex__ of list object at 0x7f8af42b4be0>), ('__repr__', <method-wrapper '__repr__' of list object at 0x7f8af42b4be0>), ('__reversed__', <built-in method __reversed__ of list object at 0x7f8af42b4be0>), ('__rmul__', <method-wrapper '__rmul__' of list object at 0x7f8af42b4be0>), ('__setattr__', <method-wrapper '__setattr__' of list object at 0x7f8af42b4be0>), ('__setitem__', <method-wrapper '__setitem__' of list object at 0x7f8af42b4be0>), ('__sizeof__', <built-in method __sizeof__ of list object at 0x7f8af42b4be0>), ('__str__', <method-wrapper '__str__' of list object at 0x7f8af42b4be0>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b668d5a0>), ('append', <built-in method append of list object at 0x7f8af42b4be0>), ('clear', <built-in method clear of list object at 0x7f8af42b4be0>), ('copy', <built-in method copy of list object at 0x7f8af42b4be0>), ('count', <built-in method count of list object at 0x7f8af42b4be0>), ('extend', <built-in method extend of list object at 0x7f8af42b4be0>), ('index', <built-in method index of list object at 0x7f8af42b4be0>), ('insert', <built-in method insert of list object at 0x7f8af42b4be0>), ('pop', <built-in method pop of list object at 0x7f8af42b4be0>), ('remove', <built-in method remove of list object at 0x7f8af42b4be0>), ('reverse', <built-in method reverse of list object at 0x7f8af42b4be0>), ('sort', <built-in method sort of list object at 0x7f8af42b4be0>)] [('__class__', <class '__main__.Vehicle'>), ('__delattr__', <method-wrapper '__delattr__' of Vehicle object at 0x7f8af813a350>), ('__dict__', {'wheels': 4, 'colour': 'red'}), ('__dir__', <built-in method __dir__ of Vehicle object at 0x7f8af813a350>), ('__doc__', None), ('__eq__', <method-wrapper '__eq__' of Vehicle object at 0x7f8af813a350>), ('__format__', <built-in method __format__ of Vehicle object at 0x7f8af813a350>), ('__ge__', <method-wrapper '__ge__' of Vehicle object at 0x7f8af813a350>), ('__getattribute__', <method-wrapper '__getattribute__' of Vehicle object at 0x7f8af813a350>), ('__gt__', <method-wrapper '__gt__' of Vehicle object at 0x7f8af813a350>), ('__hash__', <method-wrapper '__hash__' of Vehicle object at 0x7f8af813a350>), ('__init__', <bound method Vehicle.__init__ of <__main__.Vehicle object at 0x7f8af813a350>>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b9617e20>), ('__le__', <method-wrapper '__le__' of Vehicle object at 0x7f8af813a350>), ('__lt__', <method-wrapper '__lt__' of Vehicle object at 0x7f8af813a350>), ('__module__', '__main__'), ('__ne__', <method-wrapper '__ne__' of Vehicle object at 0x7f8af813a350>), ('__new__', <built-in method __new__ of type object at 0x55a6b6698ba0>), ('__reduce__', <built-in method __reduce__ of Vehicle object at 0x7f8af813a350>), ('__reduce_ex__', <built-in method __reduce_ex__ of Vehicle object at 0x7f8af813a350>), ('__repr__', <method-wrapper '__repr__' of Vehicle object at 0x7f8af813a350>), ('__setattr__', <method-wrapper '__setattr__' of Vehicle object at 0x7f8af813a350>), ('__sizeof__', <built-in method __sizeof__ of Vehicle object at 0x7f8af813a350>), ('__str__', <method-wrapper '__str__' of Vehicle object at 0x7f8af813a350>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b9617e20>), ('__weakref__', None), ('colour', 'red'), ('repaint', <bound method Vehicle.repaint of <__main__.Vehicle object at 0x7f8af813a350>>), ('wheels', 4)]
Encuentra objetos de Python usando el método hasattr()
Por último, también podemos usar el método hasattr()
para averiguar los métodos de un objeto Python. Esta función comprueba si un objeto tiene un atributo.
La sintaxis de este método es:
# python 3.x
hasattr(object, attribute)
La función toma dos argumentos: objeto y atributo. Comprueba si el atributo está presente en ese objeto en particular.
Por ejemplo:
# python 3.x
print(hasattr(my_object, "__doc__"))
Esta función devolverá true
si el atributo existe. De lo contrario, devolverá False
. Además, una vez que hayamos encontrado el método, podemos usar la función help()
para ver su documentación.
Por ejemplo:
# python 3.x
help(object.method)
Encontrar objetos usando el método getattr()
A diferencia del método hasattr()
, el método getattr()
devuelve el contenido de un atributo si existe para ese objeto Python en particular.
La sintaxis de esta función es:
# python 3.x
getattr(object, attribute)
Por ejemplo:
# python 3.x
print(getattr(my_object, "__doc__"))
Producción :
Built-in mutable sequence.
If no argument is given, the constructor creates a new empty list.
The argument must be an iterable if specified.
De la salida, está claro que el atributo existe. Por lo tanto, ha devuelto su contenido con detalles sobre cómo funciona este método.
Hasta ahora, hemos analizado varios métodos para realizar la introspección de objetos. En otras palabras, hemos enumerado los métodos y atributos de un objeto Python de 5 formas diferentes.
Siguiendo este artículo, deberíamos poder evaluar los objetos de Python y realizar la introspección.
Si esta guía te ayudó, compártela.