查找 Python 对象的方法
-
使用
dir
方法查找 Python 对象的方法 -
使用
type
函数查找 Python 对象类型 -
使用
id
函数查找 Python 对象 ID -
使用
inspect
模块查找 Python 对象的方法 -
使用
hasattr()
方法查找 Python 对象 -
使用
getattr()
方法查找对象
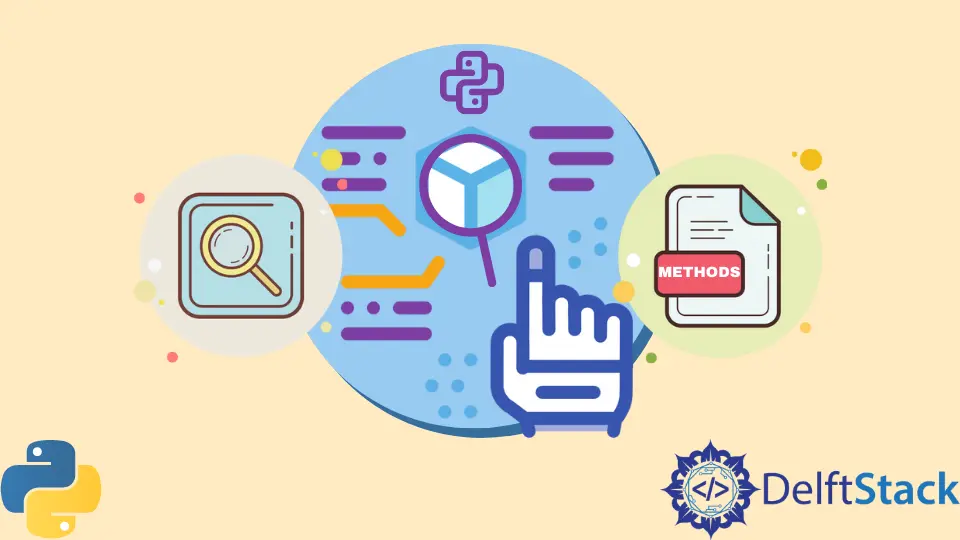
在 Python 编程中,动态查找 Python 对象的方法的能力称为自省。由于 Python 中的一切都是对象,因此我们可以在运行时轻松找到它的对象。
我们可以使用内置函数和模块检查那些。当我们想在不阅读源代码的情况下了解信息时,它特别有用。
本文介绍了我们可以用来查找 Python 对象的方法的六种简单方法。让我们深入了解一下。
使用 dir
方法查找 Python 对象的方法
查找方法的第一种方法是使用 dir()
函数。该函数将一个对象作为参数并返回该对象的属性和方法列表。
这个函数的语法是:
# python 3.x
dir(object)
例如:
# python 3.x
my_object = ["a", "b", "c"]
dir(my_object)
输出:
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
从输出中,我们可以观察到它已经返回了对象的所有方法。
以双下划线开头的函数称为 dunder 方法。这些方法称为包装对象。例如,dict()
函数将调用 __dict__()
方法。
我们已经创建了这个基本的 Vehicle Python 类:
# python 3.x
class Vehicle:
def __init__(self, wheels=4, colour="red"):
self.wheels = wheels
self.colour = colour
def repaint(self, colour=None):
self.colour = colour
如果我们创建这个类的对象并运行 dir()
函数,我们可以看到以下输出:
# python 3.x
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'colour', 'repaint', 'wheels']
我们可以看到它列出了所有方法及其属性。它显示了我们创建的方法,但也列出了该类的所有内置方法。
此外,我们还可以通过使用 callable()
函数并将对象作为参数传递来检查该方法是否可调用。
使用 type
函数查找 Python 对象类型
第二种方法是使用 type()
函数。type()
函数用于返回对象的类型。
我们可以在 type()
函数的参数中传递任何对象或值。例如:
# python 3.x
print(type(my_object))
print(type(1))
print(type("hello"))
这将显示以下输出:
<class 'list'>
<class 'int'>
<class 'str'>
type()
函数返回了一个对象的类型。
使用 id
函数查找 Python 对象 ID
要在 Python 中找出对象的 id,我们将使用 id()
函数。
此函数返回作为参数传递的任何对象的特殊 id。id 类似于该特定对象在内存中的特殊位置。
例如:
# python 3.x
print(id(my_object))
print(id(1))
print(id("Hello"))
执行这些命令后,我们将得到类似的输出:
140234778692576
94174513879552
140234742627312
使用 inspect
模块查找 Python 对象的方法
inspect
模块是我们可以用来查看有关实时 Python 对象的信息的另一种方法。该模块的语法是:
# python 3.x
import inspect
print(inspect.getmembers(object))
第一步是导入 inspect
模块。之后,我们将从 inspect
模块调用 getmembers()
函数并将对象作为参数传递。
例如:
# python 3.x
print(inspect.getmembers(my_object))
print(inspect.getmembers(Vehicle))
在上面的例子中,我们检查了两个对象:一个列表和 Vehicle 类的对象。执行代码后,我们得到以下输出:
# python 3.x
[('__add__', <method-wrapper '__add__' of list object at 0x7f8af42b4be0>), ('__class__', <class 'list'>), ('__contains__', <method-wrapper '__contains__' of list object at 0x7f8af42b4be0>), ('__delattr__', <method-wrapper '__delattr__' of list object at 0x7f8af42b4be0>), ('__delitem__', <method-wrapper '__delitem__' of list object at 0x7f8af42b4be0>), ('__dir__', <built-in method __dir__ of list object at 0x7f8af42b4be0>), ('__doc__', 'Built-in mutable sequence.\n\nIf no argument is given, the constructor creates a new empty list.\nThe argument must be an iterable if specified.'), ('__eq__', <method-wrapper '__eq__' of list object at 0x7f8af42b4be0>), ('__format__', <built-in method __format__ of list object at 0x7f8af42b4be0>), ('__ge__', <method-wrapper '__ge__' of list object at 0x7f8af42b4be0>), ('__getattribute__', <method-wrapper '__getattribute__' of list object at 0x7f8af42b4be0>), ('__getitem__', <built-in method __getitem__ of list object at 0x7f8af42b4be0>), ('__gt__', <method-wrapper '__gt__' of list object at 0x7f8af42b4be0>), ('__hash__', None), ('__iadd__', <method-wrapper '__iadd__' of list object at 0x7f8af42b4be0>), ('__imul__', <method-wrapper '__imul__' of list object at 0x7f8af42b4be0>), ('__init__', <method-wrapper '__init__' of list object at 0x7f8af42b4be0>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b668d5a0>), ('__iter__', <method-wrapper '__iter__' of list object at 0x7f8af42b4be0>), ('__le__', <method-wrapper '__le__' of list object at 0x7f8af42b4be0>), ('__len__', <method-wrapper '__len__' of list object at 0x7f8af42b4be0>), ('__lt__', <method-wrapper '__lt__' of list object at 0x7f8af42b4be0>), ('__mul__', <method-wrapper '__mul__' of list object at 0x7f8af42b4be0>), ('__ne__', <method-wrapper '__ne__' of list object at 0x7f8af42b4be0>), ('__new__', <built-in method __new__ of type object at 0x55a6b668d5a0>), ('__reduce__', <built-in method __reduce__ of list object at 0x7f8af42b4be0>), ('__reduce_ex__', <built-in method __reduce_ex__ of list object at 0x7f8af42b4be0>), ('__repr__', <method-wrapper '__repr__' of list object at 0x7f8af42b4be0>), ('__reversed__', <built-in method __reversed__ of list object at 0x7f8af42b4be0>), ('__rmul__', <method-wrapper '__rmul__' of list object at 0x7f8af42b4be0>), ('__setattr__', <method-wrapper '__setattr__' of list object at 0x7f8af42b4be0>), ('__setitem__', <method-wrapper '__setitem__' of list object at 0x7f8af42b4be0>), ('__sizeof__', <built-in method __sizeof__ of list object at 0x7f8af42b4be0>), ('__str__', <method-wrapper '__str__' of list object at 0x7f8af42b4be0>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b668d5a0>), ('append', <built-in method append of list object at 0x7f8af42b4be0>), ('clear', <built-in method clear of list object at 0x7f8af42b4be0>), ('copy', <built-in method copy of list object at 0x7f8af42b4be0>), ('count', <built-in method count of list object at 0x7f8af42b4be0>), ('extend', <built-in method extend of list object at 0x7f8af42b4be0>), ('index', <built-in method index of list object at 0x7f8af42b4be0>), ('insert', <built-in method insert of list object at 0x7f8af42b4be0>), ('pop', <built-in method pop of list object at 0x7f8af42b4be0>), ('remove', <built-in method remove of list object at 0x7f8af42b4be0>), ('reverse', <built-in method reverse of list object at 0x7f8af42b4be0>), ('sort', <built-in method sort of list object at 0x7f8af42b4be0>)] [('__class__', <class '__main__.Vehicle'>), ('__delattr__', <method-wrapper '__delattr__' of Vehicle object at 0x7f8af813a350>), ('__dict__', {'wheels': 4, 'colour': 'red'}), ('__dir__', <built-in method __dir__ of Vehicle object at 0x7f8af813a350>), ('__doc__', None), ('__eq__', <method-wrapper '__eq__' of Vehicle object at 0x7f8af813a350>), ('__format__', <built-in method __format__ of Vehicle object at 0x7f8af813a350>), ('__ge__', <method-wrapper '__ge__' of Vehicle object at 0x7f8af813a350>), ('__getattribute__', <method-wrapper '__getattribute__' of Vehicle object at 0x7f8af813a350>), ('__gt__', <method-wrapper '__gt__' of Vehicle object at 0x7f8af813a350>), ('__hash__', <method-wrapper '__hash__' of Vehicle object at 0x7f8af813a350>), ('__init__', <bound method Vehicle.__init__ of <__main__.Vehicle object at 0x7f8af813a350>>), ('__init_subclass__', <built-in method __init_subclass__ of type object at 0x55a6b9617e20>), ('__le__', <method-wrapper '__le__' of Vehicle object at 0x7f8af813a350>), ('__lt__', <method-wrapper '__lt__' of Vehicle object at 0x7f8af813a350>), ('__module__', '__main__'), ('__ne__', <method-wrapper '__ne__' of Vehicle object at 0x7f8af813a350>), ('__new__', <built-in method __new__ of type object at 0x55a6b6698ba0>), ('__reduce__', <built-in method __reduce__ of Vehicle object at 0x7f8af813a350>), ('__reduce_ex__', <built-in method __reduce_ex__ of Vehicle object at 0x7f8af813a350>), ('__repr__', <method-wrapper '__repr__' of Vehicle object at 0x7f8af813a350>), ('__setattr__', <method-wrapper '__setattr__' of Vehicle object at 0x7f8af813a350>), ('__sizeof__', <built-in method __sizeof__ of Vehicle object at 0x7f8af813a350>), ('__str__', <method-wrapper '__str__' of Vehicle object at 0x7f8af813a350>), ('__subclasshook__', <built-in method __subclasshook__ of type object at 0x55a6b9617e20>), ('__weakref__', None), ('colour', 'red'), ('repaint', <bound method Vehicle.repaint of <__main__.Vehicle object at 0x7f8af813a350>>), ('wheels', 4)]
使用 hasattr()
方法查找 Python 对象
最后,我们还可以使用 hasattr()
方法来找出 Python 对象的方法。此函数检查对象是否具有属性。
此方法的语法是:
# python 3.x
hasattr(object, attribute)
该函数有两个参数:对象和属性。它检查该属性是否存在于该特定对象中。
例如:
# python 3.x
print(hasattr(my_object, "__doc__"))
如果属性存在,此函数将返回 True
。否则,它将返回 False
。此外,一旦我们找到了该方法,我们就可以使用 help()
函数来查看其文档。
例如:
# python 3.x
help(object.method)
使用 getattr()
方法查找对象
与 hasattr()
方法相反,getattr()
方法返回该特定 Python 对象存在的属性内容。
这个函数的语法是:
# python 3.x
getattr(object, attribute)
例如:
# python 3.x
print(getattr(my_object, "__doc__"))
输出:
Built-in mutable sequence.
If no argument is given, the constructor creates a new empty list.
The argument must be an iterable if specified.
从输出中可以清楚地看出该属性存在。因此,它返回了其内容以及有关此方法如何工作的详细信息。
到目前为止,我们已经研究了几种执行对象内省的方法。换句话说,我们以 5 种不同的方式列出了 Python 对象的方法和属性。
通过阅读本文,我们应该能够评估 Python 对象并进行自省。
如果本指南对你有帮助,请分享。