How to Convert CSV File to JSON File in Python
-
Convert CSV File to JSON File in Python Using the
json.dump()
Method in Python -
Convert CSV File to JSON File in Python Using the
Dataframe.to_json()
Method in Python
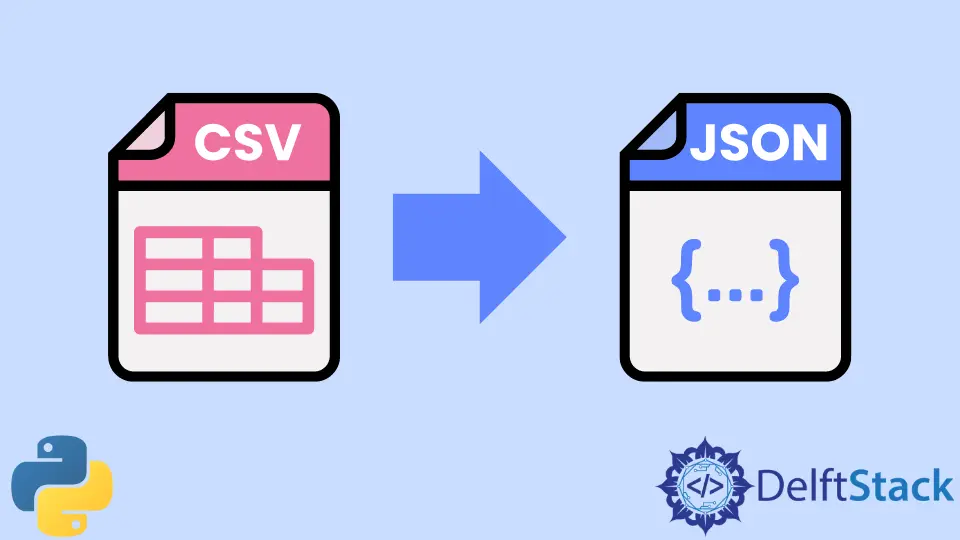
This tutorial will demonstrate various methods to read data from a CSV file and save it as a JSON file in Python. In web applications, the format used to save and transfer data is the JSON format. Suppose we have data saved in CSV (Comma Separated Values
) format, and we need to convert it to JSON format.
So, we need some method to convert the CSV format data to JSON format. We can convert the CSV file to a JSON file in Python by using the following methods.
Convert CSV File to JSON File in Python Using the json.dump()
Method in Python
The json.dump(obj, fp, indent=None, Seperator=None)
method takes data obj
as input and serializes obj
as the JSON formatted stream, and writes it to the file-like object fp
.
The indent
keyword argument can be used if we want to add the indentation to the data to make it easier to read. For indent
argument value equal to 0
, the method adds a newline after each value and adds indent
number of \t
at the start of each line.
The separator
argument is equal to (', ', ': ')
if indent
argument is None
; otherwise, it is equal to (',', ': ')
.
The below example code demonstrates how to use the json.dump()
method to save the data as JSON file in Python.
with open("file.csv", "r") as file_csv:
fieldnames = ("field1", "field2")
reader = csv.DictReader(file_csv, fieldnames)
with open("myfile.json", "w") as file_json:
for row in reader:
json.dump(row, file_json)
Convert CSV File to JSON File in Python Using the Dataframe.to_json()
Method in Python
The Dataframe.to_json(path, orient)
method of the Pandas
module, takes DataFrame
and path
as input and converts it into a JSON string, and saves it at the provided path
. If no path
is provided, the method returns the JSON string as output and returns nothing if the path
is provided.
The orient
argument is useful to specify how we want our JSON string to be formatted, and there are various options for both Series
and DataFrame
input.
Since the Dataframe.to_json()
method takes a DataFrame
as input, we will use the pandas.readcsv()
method to first read the CSV file as DataFrame
. The below example code demonstrates how to convert a CSV file to a JSON file in Python using the Dataframe.to_json()
method.
import pandas as pd
csv_data = pd.read_csv("test.csv", sep=",")
csv_data.to_json("test.json", orient="records")
Related Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python